MERN 2024 Edition - MongoDB, Express, React and NodeJS
Loại khoá học: Web Development
Build Full Stack Application from Scratch with React Router 6.4+, React Query, Mongoose 7+, Cloudinary, Axios
Mô tả
What's up everybody, this is John from Coding Addict and welcome to my MERN Stack course . During the course we will build full stack application (Jobify) from scratch, using MongoDB, Express, React and Node.js
During the course we will :
Create a React front-end application from scratch (VITE)
Add global styles to React Application
Build bunch of nice looking pages (Landing, Error, Register, Dashboard, etc)
Setup nice looking images
Setup routing using React Router 6.4+
Create server application from scratch
Use ES6 Modules on the back-end
Implement "nodemon" package
Setup MongoDB database in the cloud (Atlas)
Create routes and controllers
Extensively test in Thunder Client
Utilize "express-async-errors" package
Setup error handling in Express
Hash passwords
Setup validation layer with "express-validator"
Implement JWT for authentication and authorization
Connect front-end application with our server
Utilize "concurrently" package
Setup "proxy" in VITE
Programmatically navigate using React Router 6
Compare passwords
Setup nested pages and protected route using React Router 6
Implement logout functionality
Set JWT token in Postman programmatically
Implement various Axios configurations
Setup dayjs the front-end and back-end
Complete CRUD functionality
Setup permissions on the server
Create mock data (Mockaroo) and populate the database.
Setup nice charts and cards
Implement search/filter functionality on the server and front-end
Implement pagination on the server and front-end
Deploy MERN application to Render
Bạn sẽ học được gì
Learn how to build big full stack app from scratch
Learn how to connect Front-End application (React) with Backend application (Express, MongoDB, NodeJS)
Learn how to implement JWT for authentication and authorization
Learn how to implement React Hooks, Async/Await, React Router 6, Axios
Learn how to implement ES6 in Node
Learn how to deploy MERN app on Heroku
Learn best practices of Front-End and Server applications
Yêu cầu
- Good Grasp of Javascript
- Solid Understanding of ES6
- React Basics
- Node and Express Basics
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
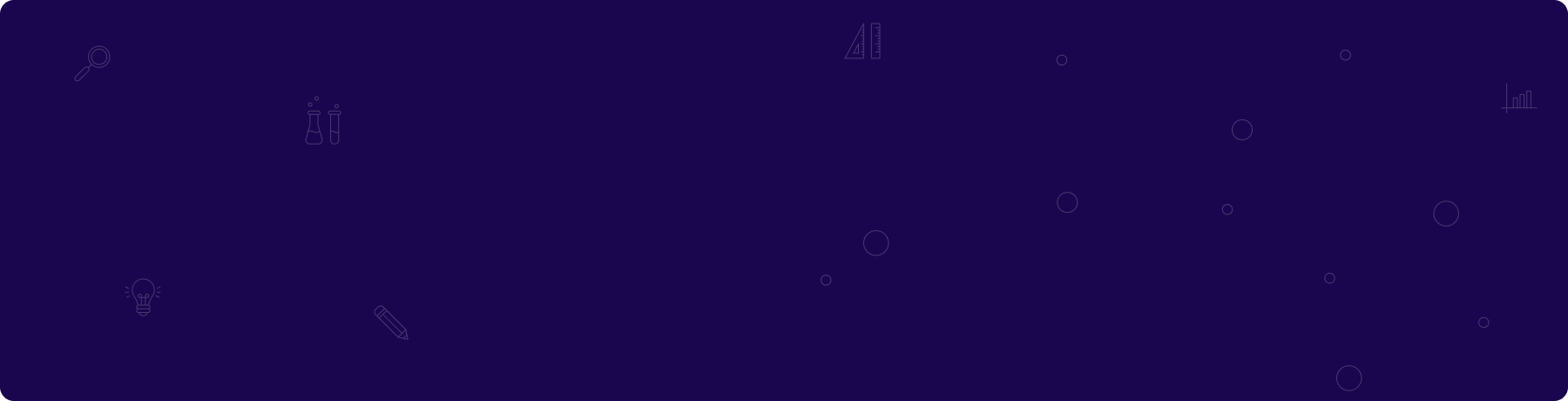
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng