Quasar V1: Cross-Platform Apps (with Vue 2, Vuex & Firebase)
Loại khoá học: Web Development
Use Quasar V1, Vue JS 2, Vuex & Firebase to build a Cross Platform App for Web, iOS, Android, Mac & Windows
Mô tả
In this course I’ll show you how to use Quasar Framework V1 (along with Vue JS 2, Vuex & Firebase) to create real-world, cross-platforms apps using a single Vue JS codebase; and get these apps production-ready and deployed to all the major platforms - Web, iOS, Android, Mac & Windows.
Throughout this course we'll create a real-world app called Awesome Todo. In this app we can add, edit or delete tasks and mark them as completed.
We can also sort tasks by name or date and search through tasks using a search bar.
It's also going to have a Settings page, with 2 real settings which change the way the app works - and which persist when app is closed and restarted (or the browser reloaded on the web version). It will also have a help page, a "visit our website" link and an "email us" link.
The app will have its own back-end created using a Firebase Realtime Database. Users can register, log in and see their data sync in realtime across all of their devices.
We'll get the app production ready for all the different platforms - web, iOS, Android, Mac & Windows.
You'll learn all of the basics of Quasar Framework, including the Quasar CLI, Quasar Components, Quasar Plugins, Quasar Directives, Platform Detection, Layouts, Theming & various Quasar Utilities.
I'll also show you all of the basics of Vue.js, including Data Binding, Events, Computed Properties, Components, Directives, Filters, Lists & Lifecycle Hooks.
You'll learn how to manage the state of your app using Vuex, where I'll cover State, Mutations, Actions & Setters.
I'll cover all of the basics of Firebase, including Authentication, Reading data, Writing data & protecting your data with Database Rules.
By the end of this course, you will be able to create your own real-world apps, with real back-ends which work on all the different platforms.
NOTE: This course is for Quasar V1 (with Vue 2). Quasar V2 (with Vue 3) is not covered in this course.
Bạn sẽ học được gì
How to create a real-world, cross-platform app for web, iOS, Android, Mac and Windows using Quasar Framework V1 and Firebase
How to manage the state of your app using Vuex
How to create a back-end for the app using Firebase Realtime Database - including user authentication, reading and writing data
All the essentials of Quasar Framework V1 and VueJS 2
Yêu cầu
- Basic HTML and CSS knowledge is required
- Basic JavaScript knowledge is beneficial but not required
- Basic VueJS knowledge is beneficial but not required
- A Mac for development is preferred
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
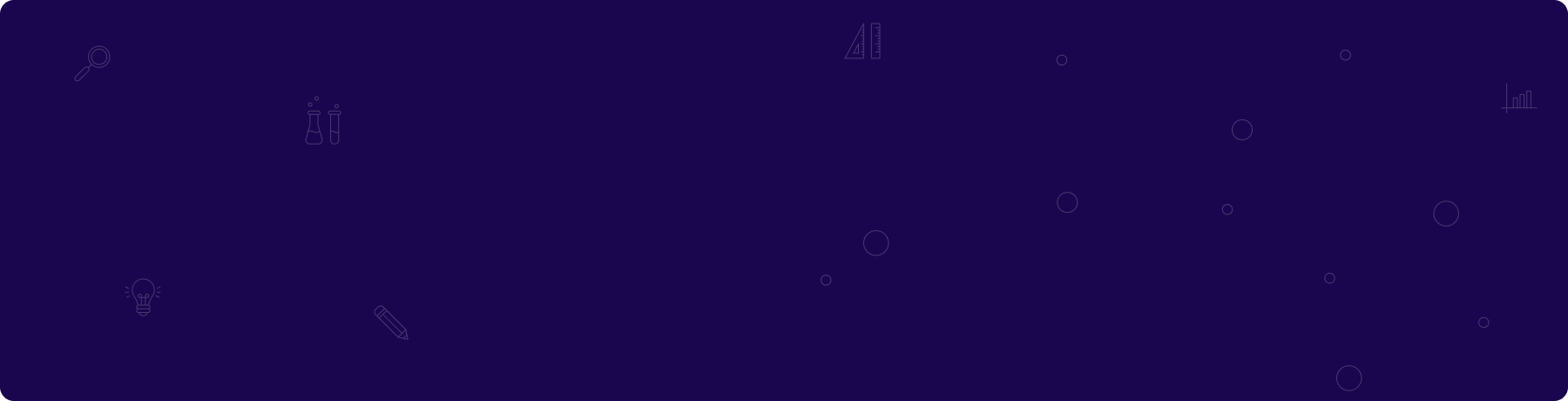
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng