TypeScript Complete Course - Beginner To Advanced + Project
Loại khoá học: Web Development
Learn TypeScript in-depth and also build a full-stack app using TypeScript with React, Material UI, Node and TypeORM.
Mô tả
The only complete TypeScript course on the marketplace to get you building TypeScript apps like a pro.
This is the only complete course about TypeScript on the marketplace. Apart from giving you complete and in-depth knowledge about TypeScript, I also teach you how to use TypeScript in conjunction with other technologies such as ReactJS, Material UI, Tanstack Query/ React Query, NodeJs, Express, TypeORM, and MySQL. This is important because while developing a project, in most cases, you would not be using TypeScript in isolation.
This course contains 240+ Videos which in total contain more than 19 hours of content. I teach you the latest features of TypeScript in a very practical manner providing you with the in-depth knowledge you need to master TypeScript.
Once we are through with the theoretical part of the TypeScript and we understand the intrinsics of the language, we set off to build a full stack application. We build a task management application where you can create a new task and set the priority as well as the status of the task. We also build real-time task counters and work on a complete server state synchronization.
While building the front end of this application, you learn how to use TypeScript with React, Material UI, React Query, and Context API. And, when we build the REST API with Node as a backend service for the application, we get practical hands-on experience on how to use Typescript in conjunction with NodeJS along with Express, TypeORM, and Mysql database. If you are a full-stack developer, you can enjoy and build the complete project with me. And of you, skillsets are limited to the backend or front-end. You can choose the module based on your skills as these modules are self-contained and do not have a dependency on each other.
Detailed Breakdown Of Topics Covered In The Course:
What is TypeScript
How TypeScript helps You Write Better Code
Introduction To Types
Different types of types in TypeScript
Primitive types
Object types
Array types
Tuples
Enums
Unions
Intersection types
Custom types
Type inference
Void
Never
Functions in TypeScript
Call signatures
Function overloading
Polymorphic functions
Generics
Classes in TypeScript
Inheritance
Accessors and Mutators
Constructors
Access modifiers
Static members
Abstract classes
Method Overriding
Interfaces
Multiple inheritance with interfaces
TypeScript Compiler and how to modify it for your needs
JavaScript Prototypes and Objects
Prototypical inheritance
Property descriptors
Decorators
Decorator factories
Class decorators
Method decorators
Parameter decorators
Decorators on static members of a class
Property decorators
Multiple decorators and execution sequence
Subtypes and Supertypes
Typecasting
Type widening
Totality
Discriminated unions
Index Accessed types
KeyOff operator
Mapped types
Conditional Types
Type definitions for third-party libraries
Building a full stack application
Application front-end using - TypeScript with React, Material UI, React Query / Tanstack Query, and Context API.
Application backend REST API using- TypeScript with NodeJS, TypeORM, and MySql
Why You Should Learn TypeScript
TypeScript is a strongly typed programming language that builds on JavaScript. TypeScript is a superset of JavaScript and can be used along with JavaScript, allowing slow and steady upgradation. TypeScript has been voted the second most loved programming language in the Stack Overflow 2020 Developer survey.
Since the introduction of NodeJS to the community a decade ago, JavaScript has become the most widely used cross-platform programming language. While the size, scope, and complexity of JavaScript programs have grown exponentially, the JavaScript language's ability to express the relationships between different entities of code has not. The most common errors that programmers make are type errors: a specific type of value was used when a different type of value was expected. This could result from simple typos, a failure to understand a library's API surface, incorrect assumptions about runtime behavior, or other errors. TypeScript's goal is to be a static type checker for JavaScript programs.
Apart from providing type checking and strict types, TypeScript comes with a bunch of features that help you to write Object Oriented Code. These features are specific to TypeScript and do not exist in JavaScript.
Who Should Take This Course?
If you are an existing JavaScript developer and want to up your JavaScript game with TypeScript. This course is a perfect fit for you.
If you already have some basic TypeScript knowledge and want to dive deep into advanced concepts. This course is a perfect fit for you.
If you want to learn how to use TypeScript and existing technologies such as React, Material UI, Context API, React Query, Node, and TypeORM. This course will help you learn TypeScript in conjunction with these technologies.
Who Should Not Take This Course?
Since TypeScript is a superset of JavaScript, this course is not for you if you have never worked on JavaScript. You do not need to be an expert at JavaScript but should have some basic knowledge of JavaScript to benefit from this course.
Suppose you are a new programmer looking for your first programming language course. Then this course is not for you. Since TypeScript builds up on JavaScript, some prior knowledge of JavaScript programming is needed to be able to take this course.
Bạn sẽ học được gì
Learn TypeScript from scratch starting with the basic type safety and types offered by TypeScript to advanced OOP features.
Deep dive into Object Oriented Programming features offered by TypeScript. Includes lectures about classes, abstract classes, access modifiers and interfaces.
Understand advanced TypeScript features such as Generics, Decorators, Totality, Type Widening, Mapped Types, Conditional Types and so on.
Create a full stack application using TypeScript and learn how to use TypeScript with technologies like React, Material UI, Tanstack Query, NodeJs, TypeORM
Use TypeScript with front-end frameworks like React JS, Tanstack/React Query, Context API and Material UI
Use TypeScript for back-end development using NodeJS, Express, TypeORM and MySQL
Yêu cầu
- Basic knowledge of JavaScript. You do not need to be an expert.
- NO prior TypeScript Knowledge Needed. You will learn TypeScript from scratch in this course.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
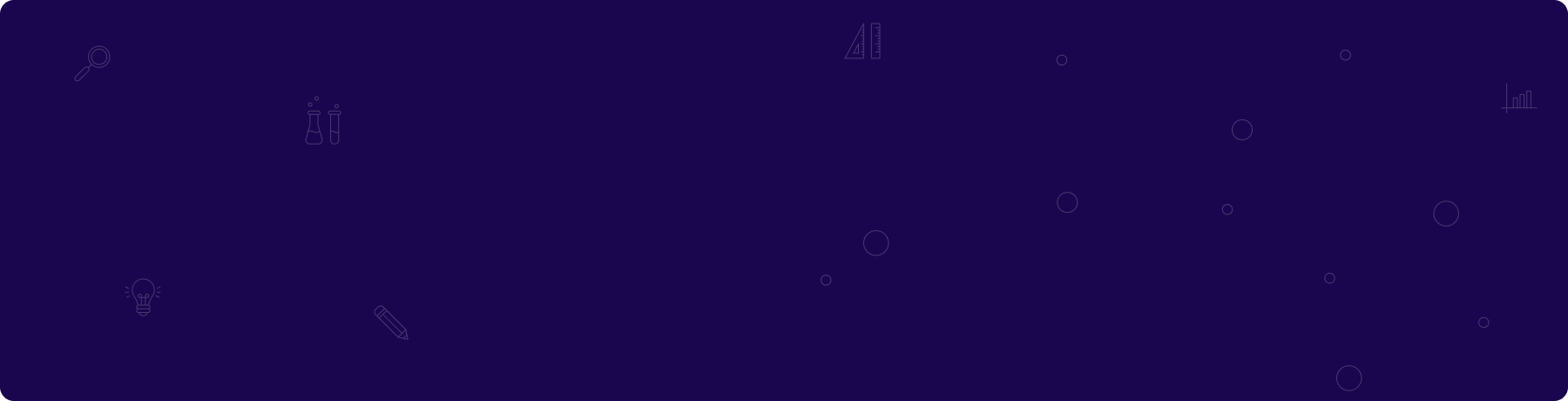
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng