.NET Core MVC - The Complete Guide 2024 [E-commerce] [.NET8]
Loại khoá học: Web Development
Build real world e-commerce application using ASP.NET Core MVC, Entity Framework Core and ASP.NET Core Identity.
Mô tả
This is a Beginner to Advanced level course on .NET 8 that will take you from basics all the way to advance mode. This course is for anyone who is new to ASP.NET Core or who is familiar with ASP.NET and wants to take the first stab at understanding what is different in ASP.NET Core. From there we would be building multiple projects to understand all concepts in .NET 8 as we will deploy our final application on Azure as well as IIS.
Throughout this course, we would understand the evolution of ASP.NET Core, and then we would take a look at the modified files and folder structure.
We would then take a look at new concepts in ASP.NET Core
We would build a small Razor application with CRUD operations using Entity framework for integration with the database.
We would build our Bulky Book website where we will learn advanced topics in ASP.NET MVC Core
Finally, we will deploy our Bulky Book website on Microsoft Azure and IIS.
What are the requirements?
3-6 months knowledge of c#
Visual Studio 2022
SQL Server Management Studio
What am I going to get from this course?
Learn the structure of ASP NET MVC Core Project
Learn the structure of ASP NET Core Razor Project
Learn the fundamentals of ASP NET MVC Core
Build 2 Projects throughout the course
Integrate Identity Framework and learn how to add more fields to Users
Interact with Razor class library for Identity
Integrate Entity Framework along with code first migrations
Sessions in ASP NET Core
Custom Tag Helpers in ASP NET Core
View Components and Partial Views in ASP NET Core
Bootstrap v5
Authentication and Authorization in ASP NET Core
Google and Facebook Authentication/Login
Role Management in ASP NET Core Identity
Email notifications
TempData/ViewBag/ViewData in ASP NET Core
Stripe Payment Integrations
Repository Pattern to Access Database
Seed Database Migrations Automatically
Deploying the website on Microsoft Azure
Bạn sẽ học được gì
Learn structure of ASP NET MVC Core (.NET 8) Project
Learn structure of ASP NET Core (.NET 8) Razor Project
Learn basic fundamentals of ASP NET MVC Core (.NET 8)
Build 2 Projects throughout the course
Integrate Identity Framework and learn how to add more fields to Users
Interact with Razor class library for Identity
Integrate Entity Framework along with code first migrations
Sessions in ASP NET Core (.NET 8)
Custom Tag Helpers in ASP NET Core (.NET 8)
View Components and Partial Views in ASP NET Core
Bootstrap v5
Authentication and Authorization in ASP NET Core (.NET 8)
Google and Facebook Authentication/Login
Role Management in ASP NET Core Identity
Email notifications
TempData in ASP NET Core (.NET 8)
Stripe Payment Integrations
Repository Pattern to Access Database
Seed Database Migrations Automatically
Deploying website on Microsoft Azure
Yêu cầu
- 3-6 months knowledge of C#
- Visual Studio 2022
- SQL Server Management Studio
- .NET 6
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
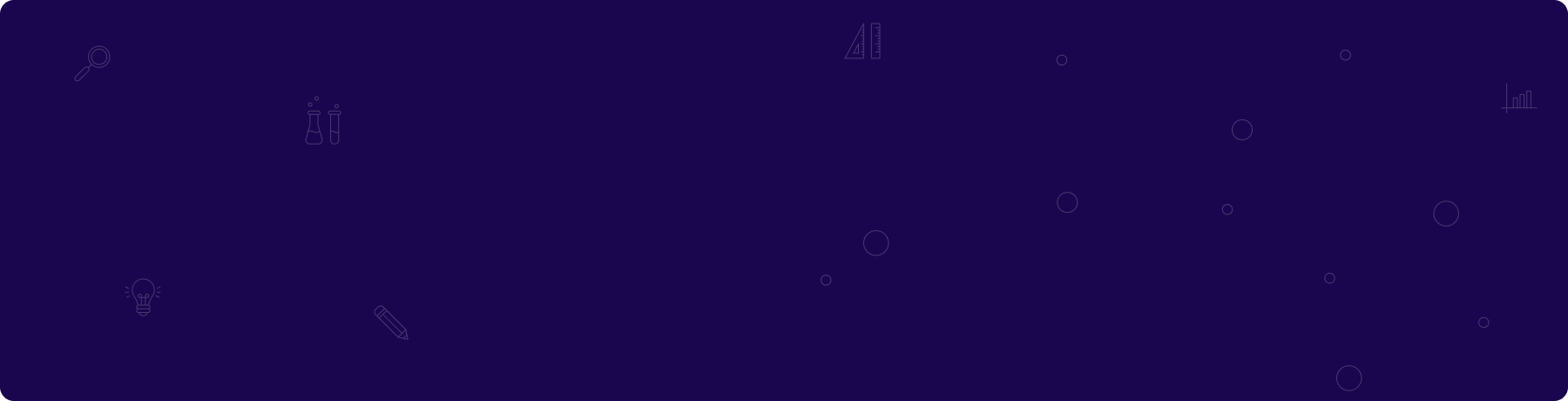
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng