Working with Vue 3 and Go (Golang)
Loại khoá học: Web Development
Learn how to work with Vue 3 and a Go back end from an award winning university professor.
Mô tả
Vue.js is, as they say on their website, an "approachable, performant and versatile framework for building web user interfaces." That sentence really does not give Vue its full due. It is arguably the best solution currently available for building highly interactive, easy to maintain, and feature-rich web applications currently available. Many developers find it easier to learn than React or Angular, and once you learn the basics, it is easy to move on to building more complex applications.
Go, commonly referred to as Golang, is an easy to learn, type safe, compiled programming language that has quickly become a favourite among people writing API back ends, network software, and similar products. The ease with which Go works with JSON, for example, makes it an ideal solution to develop a back end for a Single Page Application written in something like Vue.
This course will cover all of the things you need to know to start writing feature-rich, highly interactive applications using Vue.js version 3 for the front end, and Go for the back end API.
We will cover:
Working with Vue 3 using a CDN
Working with Vue 3 using the vue-cli, node.js and npm
Learn both the Options API and the Composition API for Vue 3
How to work with props
How to build reusable Vue components
How to build and use a data store with Vue 3
Creating, validating, and posting forms using fetch and JSON
Emitting and processing events in Vue
Conditional rendering in Vue
Animations and transitions in Vue
Working with the Vue Router
Protecting routes in Vue (requiring user authentication)
Caching components using Vue's KeepAlive functionality
Implementing a REST API using Go
Routing with Go
Connecting to a Postgresql database with Go
Reading and writing JSON with Go
Complete user authentication with Go using stateful tokens
Testing our Go back end with unit and integration tests
And much more.
Vue is one of the most popular front end JavaScript frameworks out there, and Go is quickly becoming the must-know language for developers, so learning them is definitely a benefit for any developer.
Bạn sẽ học được gì
Learn how to create interactive Web applications using Vue 3
Learn how to create a REST backend using Go (often referred to as Golang)
Learn how to create a secure user authentication system with Vue and Go
Learn best practices for creating a secure, scalable web application
Yêu cầu
- Some experience with HTML, Javascript, and Go
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
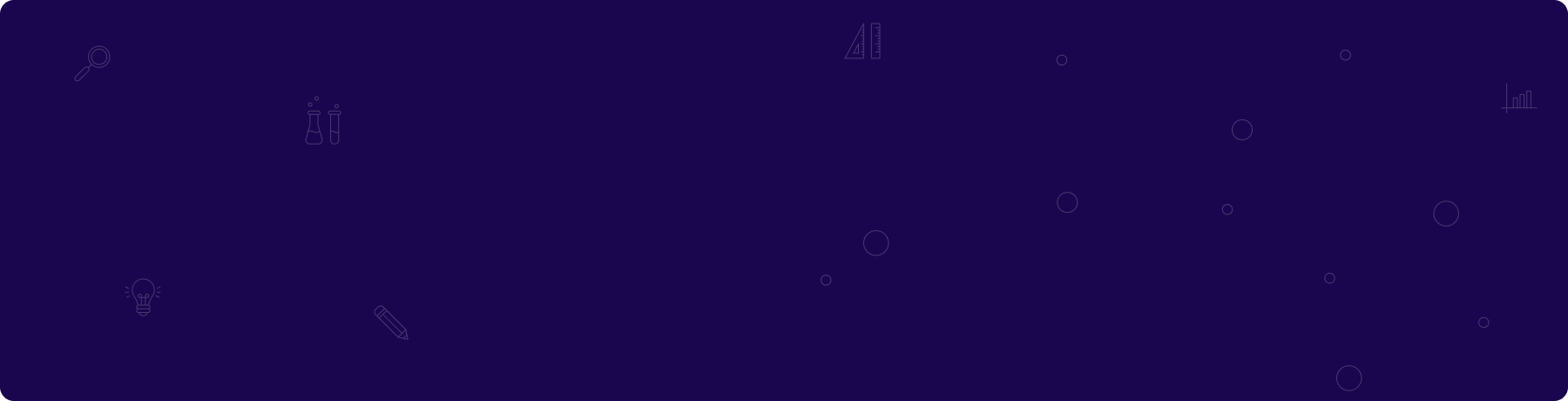
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng