Understanding Node.js: Core Concepts
Loại khoá học: Web Development
Understanding Node.js without any other NPM packages. Let's take your back-end engineering skills to the next level!
Mô tả
Welcome to the most comprehensive Node.js course on the internet!
In this course, we're going to do a deep dive into Node.js itself without cluttering our minds with other tools and NPM packages and truly master this powerful technology.
This course is heavily focused on computer science topics and fundamentals that are crucial to understand for becoming a great back-end engineer. You can only properly understand Node.js and unlock its full power if you understand these other computer science topics. So that's why we will also learn these other vital topics so that you can truly master Node.js and take your back-end engineering skills to a whole new level.
We will also use all these vital concepts that we'll learn in practice by building various exciting projects just using Node.js.
This is an intense course for people who want to get to the top of the field and get to a level of driving innovation and making an impact within the industry instead of just scratching the surface and following a few software trends and tools.
Each section of the course is like its own mini-course, and by completing each section, you'll learn some essential Node.js, computer science and back-end engineering concepts that will help you not just if you want to use Node.js but throughout your whole career as a software engineer. These things will stay with you for years and decades to come.
Let's do a quick walkthrough about what you will accomplish after completing some of the sections:
Understanding Buffers: Here, we will deeply understand buffers and how to work directly with binary data, which is essential for all the other sections.
Understanding File System: As a back-end developer, you'll work with files a lot, be it saving some data to disk, handling file uploads and many other examples, so it's essential to have a good understanding of them, which you'll gain after completing this section. We'll also learn how Node.js deals with files and master the "fs" module.
Understanding Streams: In this section, we're going to master Streams, which will allow us to develop highly-performant apps capable of handling terabytes of data with ease while having great memory usage. We'll build many mini-projects throughout the section, including an encryption-decryption app from scratch that could encrypt terabytes of data by directly modifying the binary data. This section lays the foundation for future sections where we'll utilize Streams heavily to create powerful and efficient network applications.
Understanding Networking: Node.js was primarily designed to create network applications, so it's of utmost importance that we gain a decent understanding of networking, which we will do after completing this section. Here's a list of items we'll learn in this section:
What exactly a network is
How the internet works
Mac Addresses
IPV4 & IPv6 Addresses
TCP
UDP
DNS
Fundamentals of deployment
We'll build 2 low-level apps using only Node.js, a chat and a file uploader app directly on top of TCP! And then, we'll deploy them to a Linux server in the most basic way without using unnecessary tools.
We'll see exactly what happens in our network card, every single 0s and 1s exchanged for a particular thing using Wireshark, and gain a much better understanding of networking and how most of the well-known protocols like HTTP, FTP, Email protocols, SSH, DNS and many others work. This section will broaden your horizons, and you'll realize that there are far more things that you can do with Node.js than just creating web servers.
Understanding HTTP: In this section, we'll utilize and combine all that we've learned from previous sections and finally deeply understand HTTP once and for all! We won't be learning how to use Express; instead, we will build something similar ourselves!
We'll start by understanding the most important HTTP concepts, such as connection types, client-server model, messages, requests, responses, HTTP Methods, status codes, mime types, necessary headers and so much more. And then emulate an HTTP protocol directly on top of TCP using the net module and see precisely every single 0s and 1s that get exchanged for an HTTP request and response interaction!
Once we understand the fundamentals of HTTP and the "http" module, we'll take things to the next level and start building our framework. And then, using our framework, we'll make a fully functional web application.
This section will take your web development skills to the next level. You'll have a much better understanding of how all these popular NPM packages, like Express, body-parser, Multer, cors, etc., that are built on top of the "http" module work. Well, you'll learn how to make them from scratch, just using them will not be that much of a problem!
Please note that there are many more exciting sections to come! The course is still in production, and the expected date for the course completion is within the fall of 2024.
If at any point in the course, you get stuck or feel like you need more clarification on something, I will be there in the QA to help you every step of the way from start to finish! Sign up today, and let's master Node.js and take your back-end engineering skills to a whole new level!
Bạn sẽ học được gì
Learn some of the vital concepts of back-end engineering
Get to a level capable of easily learning various NodeJS frameworks and libraries
Truly and deeply understand what exactly NodeJS is and see its full potentials far beyond just creating web servers
Understand and master NodeJS as it is without using any other NPM packages
Understand some of the most important operating systems concepts that every back-end developer needs to understand
Be able to easily learn and understand the NodeJS documentation
Learn how to directly deal with binary data and manipulate the 0s and 1s however you want
Learn the most important Networking concepts and understand where NodeJS exactly comes into play
Learn exactly what happens when you deploy your back-end applications rather than focusing on a few tools
Learn how to create low-level network applications directly on top of TCP or UDP and develop your own protocols
Deeply understand what HTTP really is and how it works
Get a deep understanding of the File System
Master Streams to develop highly performant and memory-efficient applications capable of dealing with terabytes of data with ease
Understand the EventEmitter object
Yêu cầu
- At least one year of programming experience
- A good knowledge of JavaScript
- While not mandatory, a little bit of experience with ExpressJS and HTML could be helpful
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
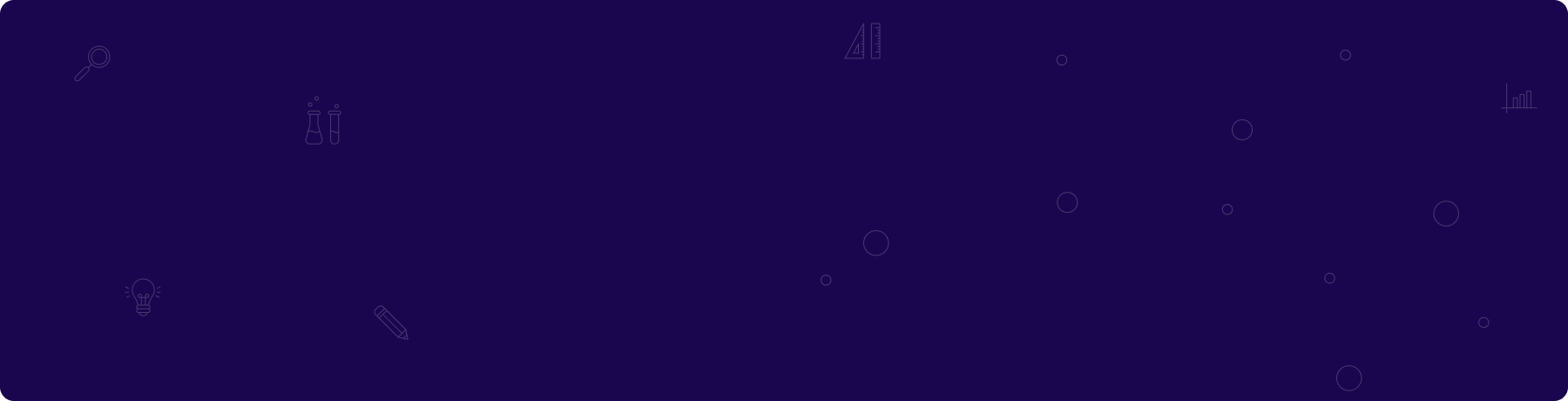
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng