[NEW] Spring Security 6 Zero to Master along with JWT,OAUTH2
Loại khoá học: Other IT & Software
Spring Security 6 , SpringBoot 3 Security, CORs, CSRF, JWT, OAUTH2, OpenID Connect, KeyCloak
Mô tả
'Spring Security Zero to Master' course will help in understanding the Spring Security Architecture, important packages, interfaces, classes inside it which handles authentication and authorization requests in the web applications. It also covers most common security related topics like CORs, CSRF, JWT, OAUTH2, password management, method level security, user, roles & authorities management inside web applications.
Below are the important topics that this course covers,
Spring Security framework details and it features
How to adapt security for a Java web application using Spring Security
Password Management in Spring Security with PasswordEncoders
Deep dive about encoding, encryption and hashing
What is CSRF, CORS and how to address them
What is Authentication and Authorization. How they are different from each other.
Securing endpoint URLs inside web applications using Ant, MVC & Regex Matchers
Filters in Spring Security and how to write own custom filters
Deep dive about JWT (JSON Web Tokens) and the role of them inside Authentication & Authorization
Deep dive about OAUTH2 and various grant type flows inside OAUTH2.
Deep dive about OpenID Connect & how it is related to OAUTH2
Applying authorization rules using roles, authorities inside a web application using Spring Security
Method level security in web/non-web applications
Social Login integrations into web applications
Set up of Authorization Server using KeyCloak
The pre-requisite for the course is basic knowledge of Java, Spring and interest to learn.
Bạn sẽ học được gì
Spring Security framework details and it features.
How to adapt security for a Java web application using Spring Security
What is CSRF, CORS, JWT, OAUTH2
Applying authorization rules using roles, authorities inside a web application using Spring Security
Method level security in web/non-web applications
Yêu cầu
- Java
- Basics of Spring framework
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
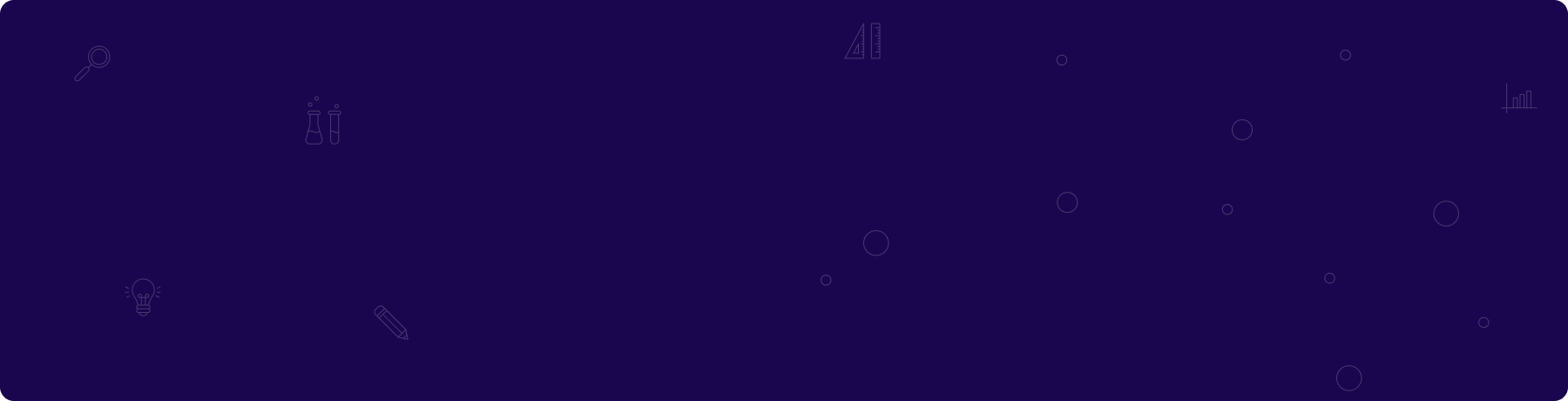
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng