The Complete Web Development Bootcamp
Loại khoá học: Web Development
Practical Full Stack Web Development Bootcamp: JavaScript, React, Python, Docker, Flask, API, Bootstrap, Git and Postman
Mô tả
This is the React and Python Flask Full Stack Web Development Bootcamp. It is a practical course where you will start building real application from the first lecture. Application will consist of the frontend and backend parts. The frontend will be built using JavaScript React. The backend API will be built using Python Flask.
The practical full stack web development bootcamp includes: JavaScript, React, Python, Flask, API, Git and VS Code
During the creation of the frontend app you will perform the following practical tasks:
Initialize a React app using create-react-app
Create different React Components
Use useEffect and useState React hooks
Adjust favicon.ico in the frontend app
Create and insert an svg logo
Making API request to the Unsplash API
Using React props and state
While making an API app you will perform the following practical tasks:
Create a Python virtual environment using pipenv
Install and use Python packages such as Flask, dotenv and Requests
Creating Flask routes
Making external API calls from the Flask app
Accepting requests from the clients
You will also learn how to use following applications:
Visual Studio Code
Git and GitHub
Postman
With this course you will get lifetime-long access to more than 100 lectures and tens of practical exercises. After the course you will become a full stack web developer with practical knowledge about JavaScript React and Python Flask.
You will also get 30-days money-back guarantee. No questions asked!
Don't wait and join the course now!
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
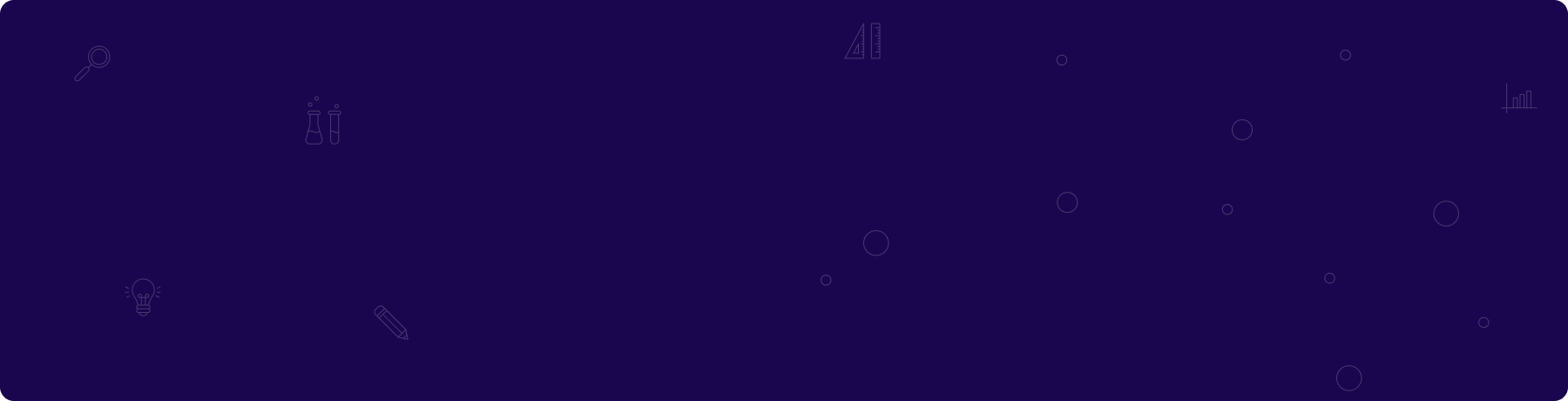
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng