Advanced C Programming Course
Loại khoá học: Other IT & Software
Become a True Master of the C Programming Language - Confidently Apply for Real Time or Embedded C Jobs or contracts!
Mô tả
What you will learn in this course?
The C programming language in 2020 is still one of the most popular and widely used languages. Having C programming skills gives you great career options, but learning the C language, particularly some of the trickier advanced stuff can be really difficult.
This course is designed to take your basic C skills to the next level and help you obtain mastery of the language by helping you understand advanced concepts of the C programming language, enabling you to master the art of problem-solving in programming using efficient, proven methods.
You’ll learn how to write high-quality C code and to make yourself more marketable for higher-level programming positions.
Just some of the topics in this huge 28-hour course include Threads, Function Pointers, Double Pointers, Recursion, Networking using Sockets, Bit manipulation, Macros, Signals, Storage Classes and loads more. Check the curriculum on this page for full details of what is included in this very comprehensive course.
By the end of this course, with your new-found skills, you will be able to apply for real-time/embedded C programming positions or any job that requires mastery of the C programming language and be able to apply your new skills developing your own Advanced C programs.
What's different about this course?
Jason Fedin is your instructor in this course and this course takes the skills you learned in that course to the next level.
This course focuses on the details and a thorough understanding of all advanced C programming concepts. This is not just a how-to course, it is a "why?" course.
You will learn how to implement specific advanced C concepts such as multi-threading and double pointers, in addition to learning why they are the best approach and how they make you a high-quality C programmer.
Many, many examples, challenges and quizzes are provided to test your understanding of every concept that you have learned.
This course is unique to other courses here at Udemy in that the details and the why are explained. We do not just go through projects and provide a how-to.
Who is the course aimed at?
This is not a beginner's course. It's assumed you have some knowledge of the C programming language, preferably having completed our Beginner course (here on Udemy) or similar training and/or commercial programming experience in C or a similar language.
Getting started
If you are ready to get started, click on the enroll or Add to Cart button on this page and start taking your C Language skills to the next level.
Bạn sẽ học được gì
Understand and be able to apply advanced concepts of the C programming language to create advanced C applications.
Understand Function and Double Pointers, Recursion, Bit Manipulation, Macros, Signals and loads more (check curriculam on this page for full list).
Learn all about threads and networking with Sockets.
Master the art of problem solving in programming using efficient, proven methods.
Be able to apply advanced C concepts to other programming languages.
How to write high quality C code, to make yourself more marketable for higher level programming positions and be apply for real-time/embedded programming positions.
Yêu cầu
- Basic knowledge of the C programming language (ideally having completed our Beginners course on Udemy).
- A computer running Microsoft Windows, Linux or the Mac operating systems.
- At least 4GB of ram on your computer.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
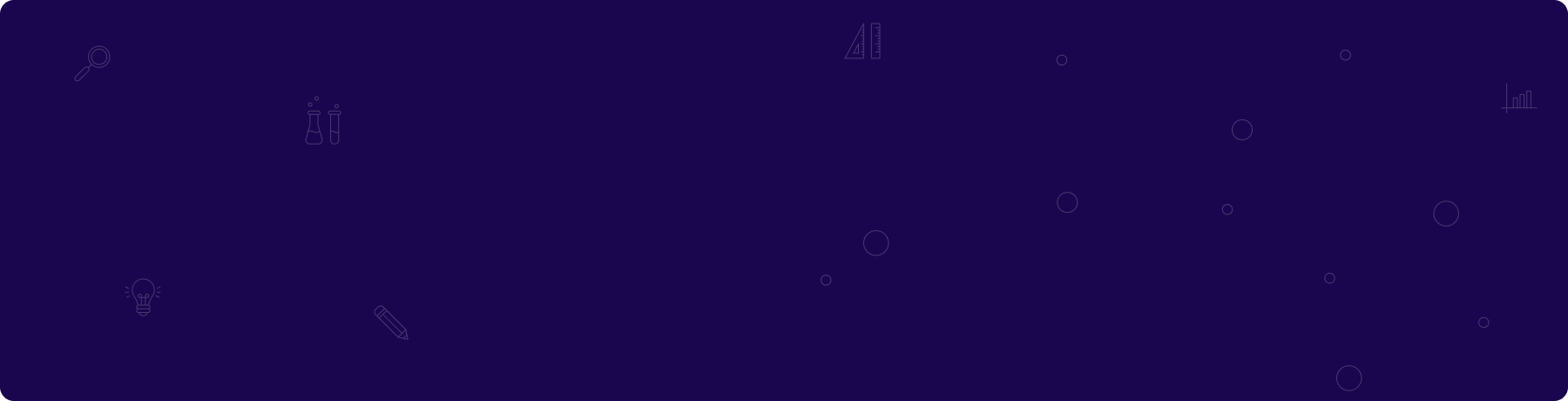
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng