Angular Deep Dive - Beginner to Advanced (Angular 17)
Loại khoá học: Web Development
Updated to Angular 16: Learn all the Advanced Features of the Angular Core and Common modules
Mô tả
This course will give you a solid foundation on the Angular platform by giving you with an in-depth guide tour of all the advanced features available in the Angular Core and Common modules.
These are the baseline modules from which all other modules in the Angular ecosystem are built with, so this is the part of Angular that you want to learn first, and in as much detail as possible.
In this course, you will get an exhaustive guided tour of all the basic and also the advanced functionality available in these two Angular essential modules (including Angular Elements).
This guide will cover everything from the most commonly used features of Angular, up until the most advanced topics of Angular Core, such as the multiple modes of change detection, style isolation, dependency injection, content projection, internationalization, standalone components and more.
The course starts from the beginning, assuming no prior knowledge of Angular. Each concept is introduced based only on previous concepts, so there are no forward references to help with the learning process.
Even though the course starts with the essential concepts of Angular, it will quickly evolve into intermediate to advanced topics. So no matter what your current Angular level is, there is something for everyone in this course!
To help you make the most out of the standalone components feature, you have available a full section on how to migrate an existing application to standalone components, and completely remove NgModules from your application.
Course Overview
This course will start with a quick and practical introduction to the Angular framework. You are going to set up your development environment, and using the Angular CLI you will quickly scaffold a small Angular project from scratch.
Using this initial playground, we are then going to answer some of the most common Angular questions: Why Angular, what are its main advantages and key features? We will answer this by demonstrating how the change detection mechanism works, and introduce some of the Angular template syntax.
We will then cover one by one all the features of the Angular Core and Common modules, which include:
Custom components with @Component
Components @Input and @Output, event Emitters
ngFor
ngIf
ngClass
ngStyle
ngSwitch
Observables
Built-In Pipes
Async Pipe
Custom Pipes
@Injectable and Custom Services
Lifecycle Hooks (ngOnInit, ngOnDestroy, etc.)
HTTP Client - GET POST PUT DELETE
@ViewChild and AfterViewInit
@ViewChildren
ng-content and Component Projection
@ContentChild and AfterContentInit
@ContentChildren
ng-template & ng-container
ngTemplateOutlet
AfterContentChecked and AfterViewChecked
ngDoCheck
Normal Change Detection
@Attribute
OnPush Change Detection
Custom Change Detection
View Encapsulation modes
@Directive
@Host
@HostListener
@HostBinding
Structural Directives
@Inject
@Optional
@Self
@SkipSelf
Hierarchical Injector
Custom Pipe
@NgModule
host, host-context, etc.
@NgPlural and other i18n features
Angular Elements
Angular Standalone Components
What Will You Learn In this Course?
This course will give you advanced practical knowledge of the Angular framework. After taking this course you will feel very comfortable building Angular Applications, as you will have a detailed understanding of everything that is made available by the Angular framework core modules.
Bạn sẽ học được gì
Code in Github repository with downloadable ZIP files per section
Understand key questions about Angular: Why Angular, what are the benefits?
Know how to build and style your own custom Angular Components
Learn in detail all the functionality available in Core Directives
Feel comfortable with Intermediate topics like Template Querying, Content Projection, Dynamic Templates and more
Have in-depth knowledge how to build custom Attribute and Structural Directives
Feel comfortable with Advanced topics like View Encapsulation, Change Detection, Dependency Injection, Lifecycle Hooks and more
Learn in detail about custom Modules, custom Pipes and Internationalization (i18n)
Learn all about Angular Elements (Advanced)
Yêu cầu
- Just some Javascript, HTML & CSS
- No previous knowledge of Angular is required
- Starts with Angular Fundamentals, but quickly evolves to Intermediate to Advanced topics
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
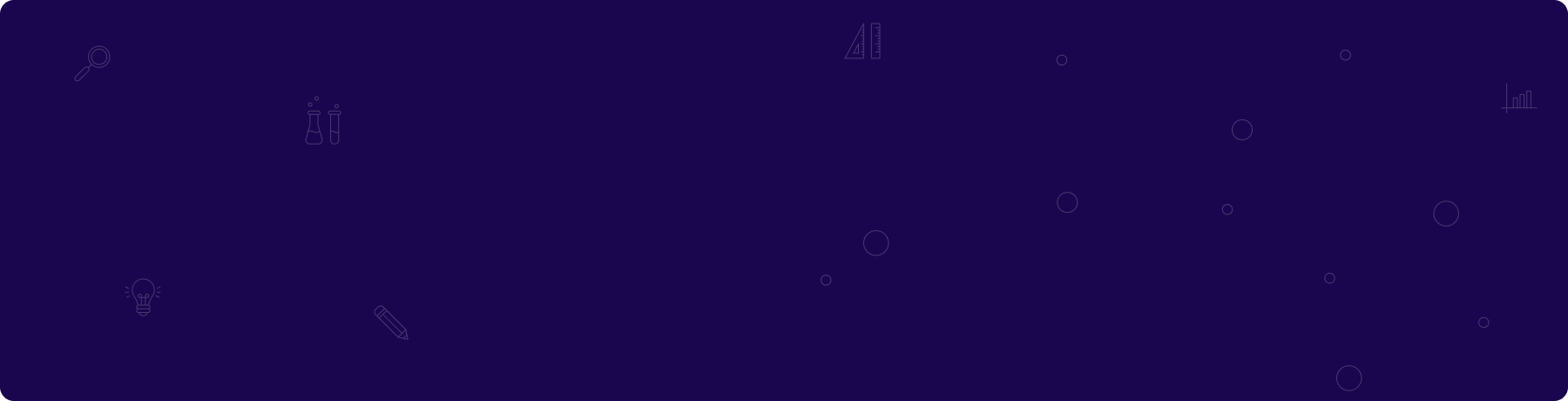
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng