Applied JavaScript by Building a Full-Stack Web App
Loại khoá học: Web Development
Learn fundamental programming concepts while building a fully-featured multiplayer game with modern JavaScript!
Mô tả
I want to teach you JavaScript in the best way possible — by directly using it! Under my guidance, you will build a modern web application that will be an interactive guessing game. You will build a REST API with Node.js and create responsive web pages powered by React and Next.js. Along this journey, you will learn all the fundamental principles that real programmers use to solve problems.
Practice makes perfect
Throughout the course, there are numerous exercise points where you are invited to pause the video and tackle a challenge so that you can practise and retrain the things you already learned. The key ingredient in this course is you. But don’t worry, I will always walk you through my solution at the end of each exercise.
The right tool for the job
JavaScript is the most popular programming language in the world, and for good reason — it is used in thousands of applications, such as backend servers, web pages and even desktop applications like the Slack messaging app. Knowing this programming language is a fantastic means to boost your career.
Learning should be fun
Programming fills me with joy. I aim to bring this passion with me when teaching my students. This course is exactly the kind that I would love to take myself — I make things fun and engaging.
Have a problem? No problem!
Errors are natural in programming. I will be in the Q&A section ready to take on your questions. Every lesson also comes with a transcript of the code changes; that way, you can also confirm your solution independently.
Let’s get started
I am thrilled to have you here and I can’t wait to take you with me on this journey. See you in the course!
- Rick
Bạn sẽ học được gì
Learn modern JavaScript to solve real problems
Understand the fundamental concepts of web development
Develop a complete web application including backend and frontend
Build a REST API with NodeJS and Express
Create responsive web pages using React and NextJS
Upload your code to GitHub
Deploy your application to AWS
Yêu cầu
- No programming experience needed — I will explain everything you need
- A computer where you can install software (Windows, Mac, Linux)
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
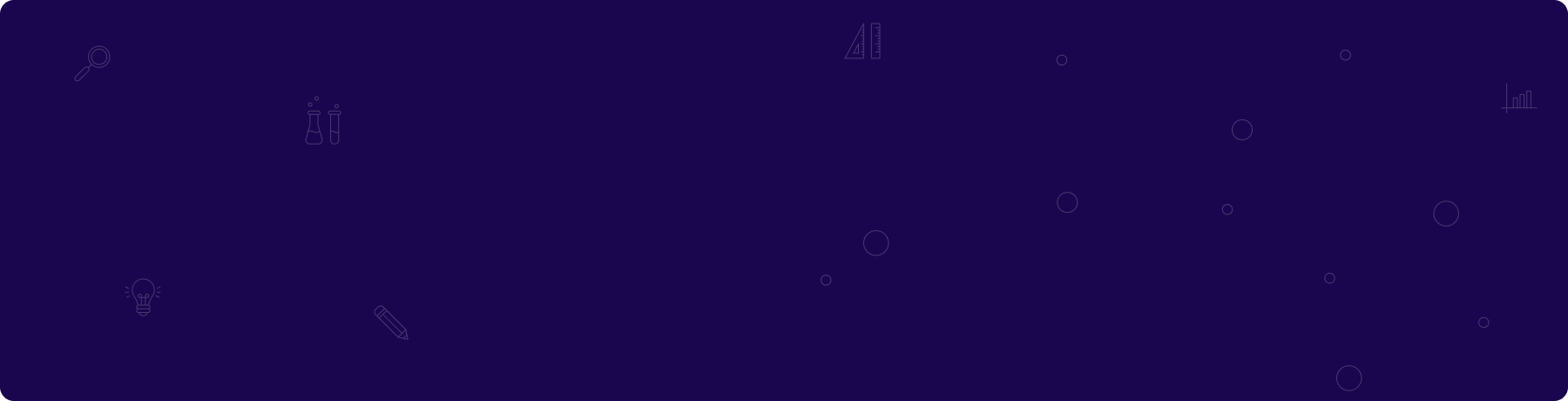
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng