Spring Framework 6: Beginner to Guru
Loại khoá học: Other IT & Software
Learn All Things Spring! Spring Framework 6, Spring Boot 3, Spring MVC, Spring Data JPA, Spring Security, Spring WebFlux
Mô tả
This course is All Things Spring!
Do you wish to master Spring Framework 6 and Spring Boot 3? Then this is the course for you.
This course is for developers with no previous Spring Framework or Spring Boot experience.
This course has been developed by a back end developer, for back end developers!
Inside this course, you will learn about:
Build a Spring Boot Web App
Use Spring for Dependency Injection
Create RESTful Web Services with Spring MVC
Create RESTful Web Services with Spring Webflux
Create RESTful Web Services with Spring Webflux.fn
Learn Best Practices using Project Lombok with Spring
Create MapStruct Mappers as Spring Components
Spring MockMVC with Mockito and JUnit 5
Spring Data JPA
Spring Data MongoDB
Spring Data R2DBC (Reactive)
Spring RestTemplate
Spring WebClient
Spring WebTestClient
Spring Security HTTP Basic Authentication
Spring Security OAuth2 Authentication w/ JWT
Spring Authorization Server
Spring WebMVC OAuth2 Resource Server
Spring WebFlux OAuth2 Resource Server
Spring Cloud Gateway
Spring Boot Maven Plugin
Spring Boot Gradle Plugin
Use Java Bean Validation with Spring
Spring Boot Auto-Configuration with MySQL
Use Spring Boot and Flyway for Database Migrations
Hibernate Database Relationship Mapping with Spring Data JPA
Course Extra - IntelliJ IDEA Ultimate
Students enrolling in the course can receive a free 4 month trial license to IntelliJ IDEA Ultimate! Get hands on experience using the Java IDE preferred by Spring Framework professionals!
Course Extra - Access to a Private Slack Community
You're not just enrolling in a course --> You are joining a community learning Spring.
With your enrollment to the course, you can access an exclusive Slack community. Get help from the instructor and other Spring Framework Gurus from around the world - in real time! This community is only available to students enrolled in this course.
This is a very active Slack community with over 18,700 Spring Framework Gurus!
This is the largest online community of people learning Spring in the world.
With your enrollment, you can join this community of awesome gurus!
Closed Captioning / Subtitles
Closed captioning in English is available for all course videos!
PDF Downloads
All keynote presentations are available for you to download as PDFs.
Lifetime Access
When you purchase this course, you will receive lifetime access! You can login anytime from anywhere to access the course content.
No Risk - Money Back Guarantee
You can buy this course with no risk. If you are unhappy with the course, for any reason, you can get a complete refund. The course has a 30 day Money Back Guarantee.
Bạn sẽ học được gì
Learn to Build a Spring Boot Web Application
Perform Dependency Injection with Spring Framework 6
Learn Best Practices for Building RESTful APIs
Use Project Lombok to Speed Up Your Development
Create RESTful Web Services using SpringMVC
Test Spring MVC using Spring MockMVC with Mockito
Use Spring Data JPA with Spring MVC
Validate Data using Bean Validation
How to Access a MySQL Database with Spring Boot
Use Flyway for Database Migrations
Create RESTful APIs Using Spring Data REST
How to use Spring RestTemplate
Configure HTTP Basic Auth with Spring Security
How to Use and Configure Spring Authorization Server
Create a Spring OAuth2 Resource Server
Learn Functional Reactive Programming
Learn Spring Data Mongo with Webflux-fn
How to Configure Spring Cloud Gateway
Yêu cầu
- Basic Java knowledge is required
- HTTP and HTML Knowledge is very helpful
- Knowledge of SQL and databases is helpful
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
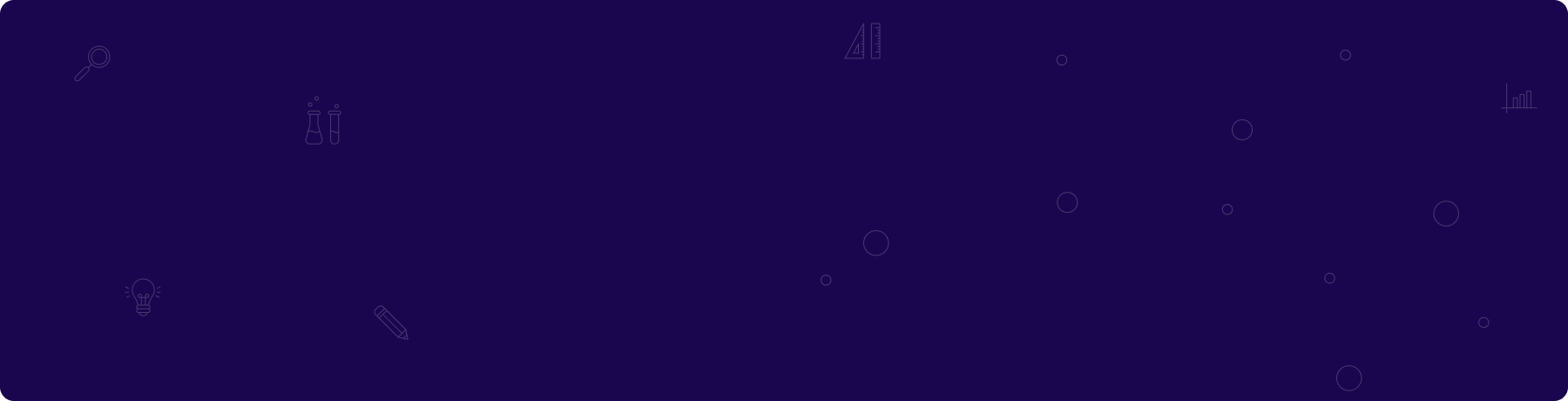
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng