Build RESTFUL APIs using Kotlin and Spring Boot
Loại khoá học: Programming Languages
A complete hands on approach to learn the Kotlin language and build Restful APIs using Kotlin SpringBoot.
Mô tả
Kotlin is the Modern, concise and safe programming language and is one of the popular JVM language in this day and age.
It’s also interoperable with Java and other languages, and provides many ways to reuse code between multiple platforms for productive programming.
This course will focus on using Kotlin for Server-Side Development using SpringBoot framework. This is a pure hands-on oriented course which covers these two topics:
Covers Kotlin Fundamentals thats necessary for Java Developers
Build RestFul APIs using SpringBoot and Kotlin
Section 1: Getting Started With the Course
This section covers the course objectives and the prerequisites that are needed to make the most out of this course.
Section 2: Getting Started with Kotlin Programming Language
In this section, I will introduce you to Kotlin Programming Language and why its a powerful language for enterprise development.
Introduction to Kotlin
How Kotlin Works with the JVM?
Section 3: Kotlin Fundamentals
In this section, we will explore the fundamentals of Kotlin.
val & var variables in Kotlin
Basic Types - Int, Long, Double, String
Conditionals - If and when block
Ranges , Loops
while & do-While
break, labels and return
Section 4: Functions in Kotlin
In this section, we will learn about functions in Kotlin and different ways of declaring and using them
Defining and Invoking Functions
Default Value Parameters & Named Arguments
Top-Level Functions and Top-level Properties
Section 5: Classes, Interfaces and Inheritance
In this section, we will learn about classes, inheritance and interfaces in detail.
Introduction to class - Creating a class and objects
Primary Constructors
Secondary Constructors
initializer code using init block
Data Classes
Custom Getters and Setters
Inheritance - Extending Classes
Inheritance - Override Functions, Variables
object keyword for creating instance of the class
companion object Keyword
Interfaces
Interfaces - Handling Conflicting Functions
Interfaces - Defining and Overrding Variables
Visibility Modifiers
Type Checking, Casting and Smart Cast
Enum class
Section 6: Nulls in Kotlin
In this section, we will learn about handling nulls in Kotlin
Nullable & Non-Nullable types in Kotlin
Safe Call(?) , Elvis Operator(?:) & Non Null Assertion(!!) to deal with Null Values
Invoking or assigning a Nullable Type to a Non-Nullable Type
Section 7: Collections, Arrays & Lamda Expressions
In this section, I will introduce you to collections, arrays and lambda expressions in Kotlin
Introduction to Collections
Introduction to Lamda Expressions
Lambdas and Higher Order Functions
Filter Operations on Kotlin Collections
Map Operations on Kotlin Collections
FlatMap Operations in Collections
Working With HashMaps
Lazy Evaluation of Collections using Sequences
Nullability in Collections
Section 8 : Exceptions In Kotlin
In this section, I will cover the exceptions in kotlin and the techniques to handle them.
Handling Exceptions using try-catch
Section 9 : Scope Functions
In this section, I will introduce you all to scope functions in Kotlin and its usage.
Introduction to Scope Functions
apply & also Scope Function
let Scope Function
with & run Scope Function
Section 10 : Getting Started with Kotlin and Spring Boot
In this section, I will explain the overview of the app we are going to build and build a very simple API.
Overview of the app & Project Setup
Build a Simple Endpoint - Greeting Controller
Constructor Injection in Spring
Setting up different profiles in Spring Boot
Set up Logging in Kotlin
Section 11 : Integration/Unit Testing using Junit 5
In this section, I will code and explain about the techniques to write different types of test cases using spring boot and Kotlin.
Introduction to Automated Tests & Setting up JUnit5
Integration Test for Controller
Unit Test for Controller - Using the Mockk Mocking library
Section 12 : Build the Course Catalog Service
In this section, we will build the Course Catalog Service to manage the Courses
Set up the Course Entity & CourseDTO
Create CourseRepository & Configure JPA in application.yml file
Build the POST Endpoint for adding new Course I
ntegration test for the POST endpoint using JUnit5
Build the Get Endpoint to retrieve all Courses
Integration test for the GET endpoint to retrieve all the courses
Build the Update Endpoint to update a Course
Integration test for the PUT endpoint using JUnit5
Build the DELETE endpoint to delete a Course
Section 13: Unit Testing Controller layer (Web Tier)
In this section, we will code and learn about how to write unit tests for the controller
Setting up the Unit Test for the CourseController
Unit test for the Post Endpoint in CourseController
Unit test for the GET Endpoint in CourseController
Unit test for the PUT Endpoint in CourseController
Unit test for the DELETE Endpoint in CourseController
Section 14 : Bean Validation using Validators and ControllerAdvice
In this section, we will code and learn the different techniques to apply bean validations and handle exceptions using the ControllerAdvice Pattern
Name and Category as Mandatory using @NotBlank Annotation
Implement Custom Error Handling using ControllerAdvice pattern
Handle Global RuntimeException using ControllerAdvice Pattern
Section 15 : Custom JPA queries using Spring Data JPA and DB Layer testing using @DataJpaTest
In this lecture, we will learn about the techniques to write custom JPA queries and the techniques to test the DB layer using the DataJpaTest
Retrieve Courses By Name using JPA Query Creation Function
Retrieve Courses By Name using Native SQL query
Testing Mutliple sets of Data using @Parameterized test
Section 16: GET Endpoint to retrieve Courses By Name using @RequestParam
In this section, we will code and learn about the usage of RequestParam in the controller endpoint.
Use existing GET endpoint to retrieve Courses by Name
Write Integration test to retrieve course by Name
Section 17 : Entity RelationShips using Spring Data JPA
In this section, I will explain the technique to express the relationships in JPA using Entity and Data classes in Kotlin
Adding Instructor Entity in to the Course Catalog Service
Adding the relationship in the Entity Class
Instructor Controller to Manage Instructor Data
Update CourseService to validate Instructor Data
Fix the CourseController Integration Tests
Fix the CourseController Unit Tests
Section 18 : Integrating with Postgres DB
In this section, we will code and learn to integrate the postgres DB in to the course catalog service.
Setting up the Postgres DB and App to interact with Postgres
Test the app with Postgres DB
Section 19 : Integration Testing using TestContainers
In this section, we will code and learn to integrate the testcontainers to run integration test.
Setting Up TestContainers for the Integration Test
Configure @DataJpaTest with TestContainers
Section 20 : Java & Kotlin Interoperability
In this section, we will code and learn about the interoperability between Java and Kotlin.
Invoking Kotlin Code from Java Class
Invoking Java Code from Kotlin
Useful JVM annotations in Kotlin
By the end of this course, you will be comfortable writing code using the Koltin Programming language and Build RestFuL APIs using SpringBoot and Kotlin.
Bạn sẽ học được gì
Kotlin Programming Language and its benefits
Write Code using Kotlin Programming Language
Building Applications using Kotlin
Build RestFul Services using SpringBoot and Kotlin
Test Kotlin using JUnit5
Kotlin and Java Interoperability
Integrate Spring Data JPA with Kotlin
Integration testing DB Layer using TestContainers & JUnit5
Unit Testing Functions calls using Mockk Library
Yêu cầu
- Java 11 or Higher is Required
- Experience working with any IDE( Intellij, Eclipse)
- Experience working with Java
- Experience building application using SpringBoot
- Gradle or Maven Build tool Experience is must
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
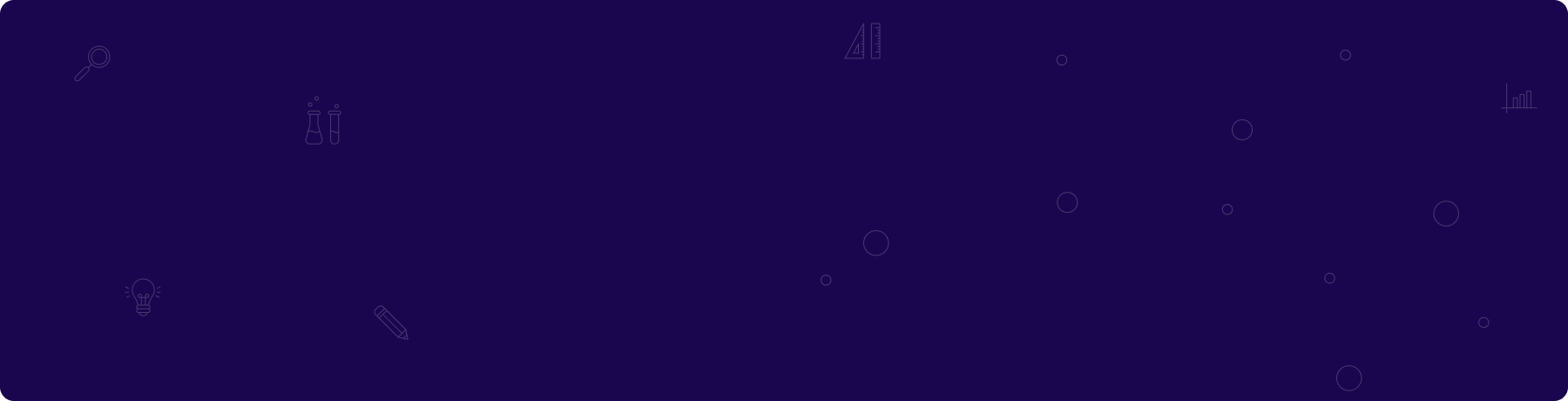
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng