Building Applications with React 17 and ASP.NET Core 6
Loại khoá học: Web Development
Use React, Hooks, ASP.NET Core, Entity Framework Core, Bootstrap, Leaflet and JWT to create a complete web application
Mô tả
With ASP.NET Core we can develop Web APIs using C#.
With React you can create modern, fast and flexible web applications.
In this course we will use both tools to create a project. We will make an application with its database, user system, back-end and UI, where you will put into practice the concepts learned in the course.
At the end we are going to publish our React application and our ASP.NET Core application.
Some of the topics that we will see:
Web API development with ASP.NET Core
Database in SQL Server using Entity Framework Core
User system with Json Web Tokens (JWT)
Developing a Single Page Application (SPA) with React
Use React Hooks to create modern functional components
Creating forms using formik and yup
Make HTTP requests from React to ASP.NET Core using Axios
Create reusable components in React
Using React Router for browsing
Using maps with leaflet
Save spatial data to a database with NetTopologySuite
Allow users to upload images to be saved to Azure Storage or locally
We will configure CORS to allow our React application to communicate with our Web API
We will use environment variables, both in ASP.NET Core and React, so as not to hardcode the development and production URLs in our applications.
Upon completion of this course, you will have sufficient knowledge to face development challenges involving ASP.NET Core and React applications.
Bạn sẽ học được gì
Make a Web API with .NET Core
Develop React web applications
Use React Hooks for building functional components
Use Entity Framework Core for building a SQL Server database
Yêu cầu
- The student should know the basics of HTML5 and CSS3
- The student should know the basics of C# (functions, variables, loops, conditionals)
- The student should know the basics of JavaScript
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
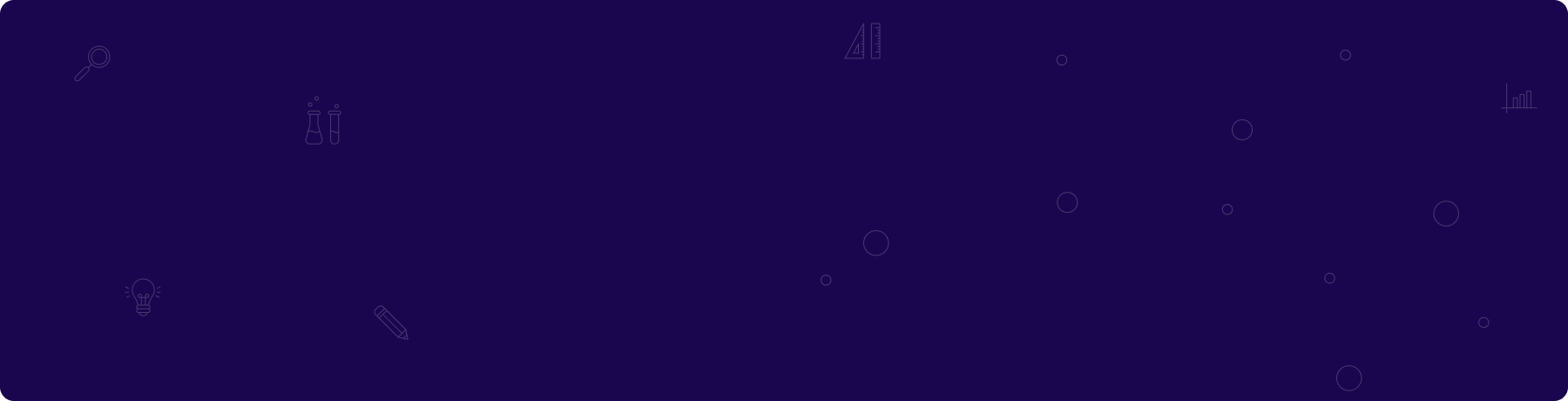
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng