Building Minimal APIs with ASP.NET Core 8 and EF Core
Loại khoá học: Web Development
ASP.NET Core, Web APIs, SQL Server, User System, GraphQL, Redis, REST, and more!
Mô tả
Learn how to develop Minimal APIs with ASP.NET Core from scratch with this amazing course.
We are going to see the entire life cycle of developing a Web API, from creating the solution, developing the endpoints, working on resource manipulation, to putting it into production in Azure and IIS.
In this course we will do a project which you will be able to publish and show as part of your portfolio.
We will also learn how to use Azure DevOps to configure a Continuous Integration and Continuous Delivery pipeline, to be able to publish your projects from their source code in Github, Bitbucket, or any other GIT repository provider.
Some of the topics we will see are:
Creation of REST Web APIs
Create a database
Use Entity Framework Core to read, insert, update, and delete records from a database
Create a user system so that our clients can register and log in to the Web API
We will use Json Web Tokens (JWT) for authentication
Claims-based authorization, so that only some users can use certain endpoints
Using cache to have a faster application
Using Redis for distributed cache
We will use GraphQL so that customers can indicate exactly what they want to consult
Web APIs are fundamental in modern web development. Since they allow us to centralize and protect the logic of our solutions. In addition, it is in a Web API that we typically have access to a central database with which all your users can communicate. Whether you build a social network, a delivery application, or even an office app, a Web API allows you to work on the back-end of mobile applications (Android, iOS, MAUI, etc.), web (React, Angular, Blazor, Vue, etc.), desktop, among others.
Bạn sẽ học được gì
Build Web APIs with .NET Core
Publish Web APIs in Azure
Use Azure DevOps to configure continuous integration and continuous delivery (CI/CD)
Develop a Web API using Minimal APIs
Yêu cầu
- Basic knowledge of C# (know what a class is, invoking functions, if statements, loops)
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
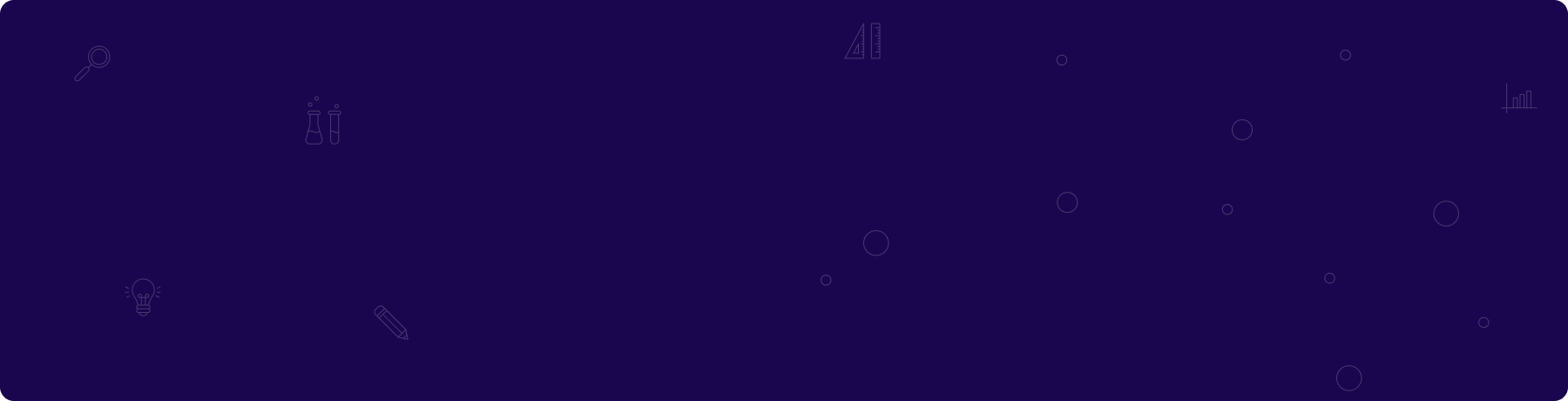
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng