C/C++ Pointers & Applications
Loại khoá học: Other IT & Software
Learn the applications of pointers such as usage in dynamic arrays, linked lists, function pointers, callbacks, etc
Mô tả
C & C++ are very powerful languages when it comes to performance & flexibility. But there are some features that are complex and take time to master. One of such features is pointers. Pointers is what separates C/C++ from other languages. These are incredibly powerful as they allow programs to access memory directly and manipulate it.
This course focuses on pointers and their applications.It leans more towards implementation in C++, rather C. You'll learn the basics of pointers and then move on to understanding and implementing arrays, pointers to arrays & heap based arrays. You'll also learn advanced memory management by creating a custom dynamic array (just like std::vector<T> in standard C++ library). You'll use placement new & delete to directly place objects in a memory pool allocated through operator new function. As you'll see later in the course, this is a powerful mechanism to optimize usage of heap memory with user-defined objects.
After arrays, you'll learn how to use pointers to create node-based data structures. We'll focus on two types of linked lists - singly & doubly linked lists. You'll understand the difference between arrays and lists and also learn how to access the elements of both the data structures without having to know their internal structure. This is possible by creating context variables that allows access in a container-agnostic manner.
Pointers are invaluable while working with strings. You'll learn how to create dynamic strings using pointers. This will be shown with an implementation of a string class.
The next important topic you'll learn and implement will be function pointers. You'll understand how function pointers work and how we can simplify their syntax. You'll also master the complexity of creating arrays of function pointers and functions that return function pointers. On top of that, you'll be comfortable with functions returning pointer to functions that themselves return pointer to other functions. Confused? See the section on function pointers.
That's not all. You'll also learn how to create pointer to members (which have even a more complex syntax than function pointers).
Furthermore, you'll learn how to create callbacks through functions pointers. This course will show you how to optimize the callbacks through function objects. Function objects are more powerful than functions pointers as callbacks.We'll use this knowledge and apply it in many examples to reinforce the concept of pointers to functions.
This course also introduces some commonly used containers of the C++ standard template library (STL), such as std::array, std::vector & std::list. By the time you hit these topics, you'll already know how these are implemented internally. How about that!
This course relies on some modern C++ (C++11) features to simplify things, such as auto, std::initializer_lists, type aliases. Even if you don't know about these features, the course has videos on these topics to get you started. Additionally, there are four full length videos dedicated to discussion on move semantics.
I hope you enjoy this course!
Bạn sẽ học được gì
Understand in depth how pointers work
Understand the applications of pointers
Understand efficient implementation of basic data structures
Understand how callback mechanism works through pointers and objects
Yêu cầu
- Basic knowledge of C & C++
- Should understand basic syntax of pointers
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
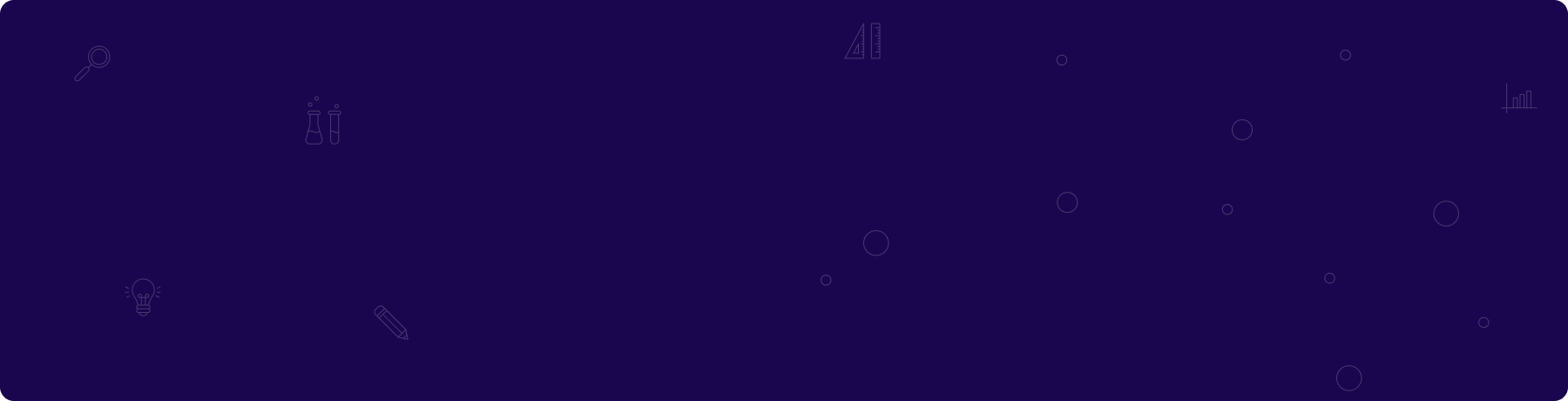
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng