Competitive Programming Essentials, Master Algorithms 2023
Loại khoá học: Software Engineering
Master competitive coding techniques - maths, number theory, dynamic programming, advanced data structures & algorithms
Mô tả
Equip yourself with essential programming techniques required for ACM-ICPC, Google CodeJam, Kickstart, Facebook HackerCup & more. Welcome to Competitive Programming Essentials - the ultimate specialisation on Algorithms for Competitive Coders!
The online Competitive Programming Essentials by Coding Minutes is a highly exhaustive & rigorous course on Competitive Programming. The 50+ hours course covers the breadth & depth of algorithmic programming starting from a recap of common data structures, and diving deep into essential and advanced algorithms.
The course structure is well-researched by instructors who not only Competitive Coders but have worked with companies like Google & Scaler. This course will help you to get a solid grip of fundamental concepts & comes with practice questions so that you sail through online coding challenges and code-athons with ease. The course is divided into 10 modules and 50 sections covering topics like Mathematics, Number Theory, Bitmasking, Inclusion-Exclusion, Meet in the Middle Techniques, Segment Trees, Fenwick Trees, Square Root Decomposition, Graph Algorithms, Shortest Paths, Game Theory, Pattern Matching, Binary Search, Greedy Techniques, Dynamic Programming and even more.
The problem setters of the course are Siddharth Singhal and Rajdeep Singh. Both are upcoming software developers at Microsoft and Razorpay respectively. They both exhibit excellent knowledge of Data Structures and Algorithms and are avid competitive programmers.
Many top companies like Google, Facebook, Amazon, Directi, CodeNation, Goldman Sachs etc encourage Competitive Programming and conduct coding competitions to hire smart people who can solve problems.
Course Highlights
Instructors from Google & Scaler Academy
50+ hours of high quality & structured content
In-depth coverage of all topics
Exhaustive Course Curriculum
Code Evaluation on Coding Exercises
Lifetime Access
Complimentary TA Doubt Support
Bạn sẽ học được gì
Understand & implement important techniques in Competitive Programming
Learn advanced techniques to optimise naive solutions
Ace code-thons and online coding competitons on Codeforces, HackerRank
Get ready for ACM-ICPC, Google Kickstart, CodeJam & more
Yêu cầu
- Comfortable with at-least one programming language
- Understanding & ability to code using data structures
- Basic problem solving experience
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
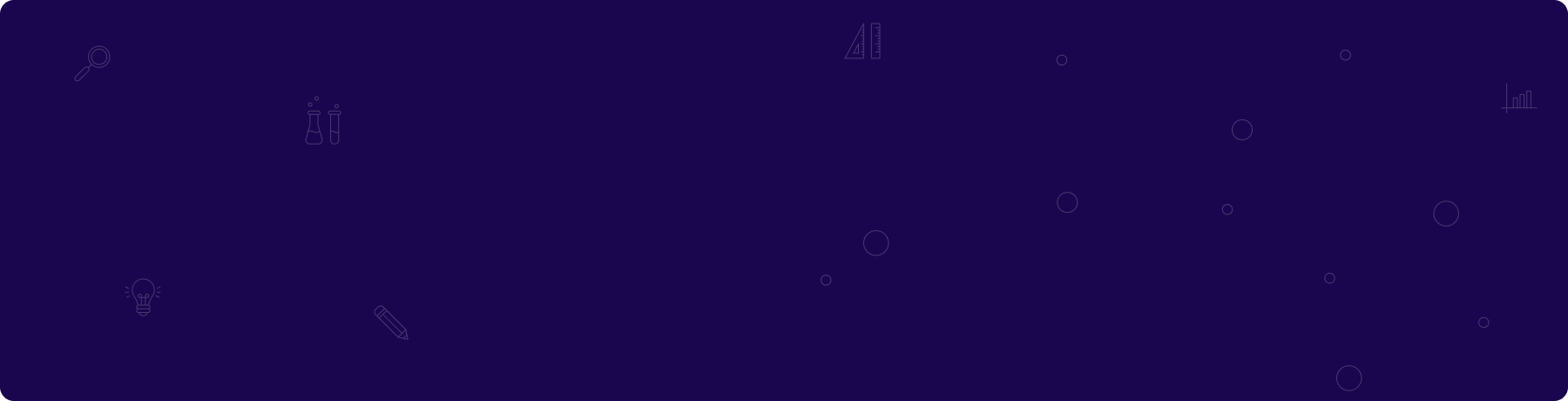
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng