Comprehensive Ruby Programming
Loại khoá học: Programming Languages
Learn how to program in the Ruby programming language, starting from scratch and moving to advanced coding techniques.
Mô tả
Course updated April 2021: Ruby 3 tutorials added along with a deep dive into Ruby modules and a Ruby Gem walk through that shows how to generate QR Codes in Ruby.
Coding has become one of the most critical skills you can have for furthering your career. Whether you are an experienced developer who wants to learn a new language or you are new to programming, this course can be your comprehensive Ruby coding guide. Starting with the foundational principles such as syntax and scaling up to advanced topics like metaprogramming and big data analysis, I wanted to create a curriculum that will give you all of the tools you need to be a professional Ruby developer. A few of the key topics that you will learn in this course are:
Object oriented programming
Built in Ruby methods
Core programming skills
Custom algorithm development
Big data analysis
Metaprogramming
Using Ruby Gem libraries
Regular Expressions
Ruby programming best practices
An introduction to the Ruby on Rails and Sinatra web frameworks
Building 10 Ruby programs that solve complex Project Euler mathematical equations
And much more!
With over a decade of real world development experience, I have engineered this curriculum to ensure it focuses on the skills you will need to be a professional Ruby developer. Each section has an interactive quiz to ensure you are understanding the material and you also will be given access to the source code for each lesson. After you have completed all of the videos and quizzes you will be given a certificate of completion in Comprehensive Ruby Programming and you will be ready to start building real world Ruby projects. Finally, in addition to the video lectures and quizzes, I will also be your code mentor throughout the course and will walk you through any challenges that you may face.
Bạn sẽ học được gì
Build programs in the Ruby programming language
Know how to work with the Ruby syntax
Create custom algorithms
Work with loops and iterators
Learn object oriented programming
Work with OOP inheritance
Start building real life programs in Ruby
Yêu cầu
- This course starts at the beginning with how to install Ruby and work with it on multiple machines, so simply have a computer that's connected to the Internet and you'll be ready.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
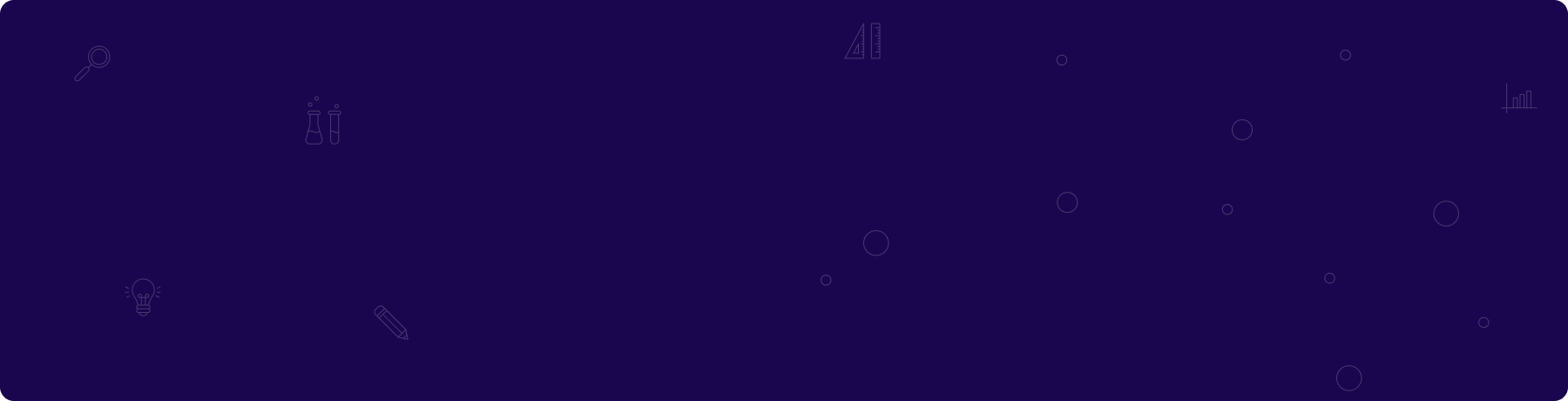
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng