Data Structures and Algorithms : DSA using C++
Loại khoá học: Programming Languages
DSA made easy. Learn, Analyze and CODE all Data Structures with examples. Learn about Recursion and Sorting
Mô tả
You may be new to Data Structure or you have already Studied and Implemented Data Structures but still you feel you need to explore more about Data Structure in detail so that it helps you challenging problems, Coding Challenges and work on Data Structures efficiently.
Whatever the reason, if you are looking for a course that focus on the implementations to give you a complete understanding of how things work, then this is the course for you.
This course goes over the theory of how things work, but only to give you what you need to know to understand the implementation covered.
This course about 50 hours covers each topic in greater details, every topic is covered on Whiteboard which gives you a classroom environment to understand the concepts of Data Structures clearly and improve your Problem Solving and Analytical Skills. Every topic is discussed, analysed and implemented with programs executed showing line-by-line coding and tracing each and every piece of Code.
After completing this course, you will have a clear understanding of Data Structures and Algorithms, how to analyse and implement them.
Course Contents
1. Working of Recursion
2. Arrays Basics and Representation
3. Array Abstract Data Type
4. Linked List and its types
5. Stacks and its applications
6. Queues
7. Trees with Binary Trees and other types
8. Dictionaries
9. Binary Search Tree
10. AVL Trees
11. Graphs
12. Hashing Technique
with Essentials of C++ Programming, which will be helpful in Implementing the Data Structures using C++
Bạn sẽ học được gì
Gain in-depth knowledge about each Data structures including Arrays, Linked List, Stacks, Queues, Trees, Graphs, Heaps, Hashing and Sorting
Coded fully using Object Oriented Programming Concepts in C++
Complete working of each Data structure with tracing during learning the concepts as well as during program execution
Analyse in-depth each of the Data structures
Basics to Advance Level Data Structures
Yêu cầu
- Some basic or no knowledge of C++
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
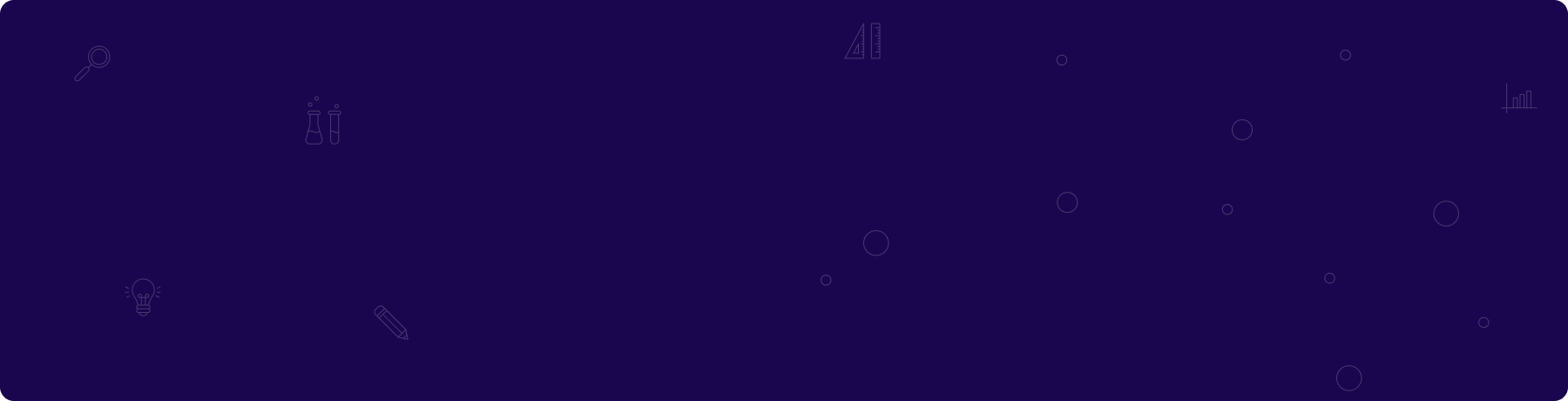
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng