Data Structures and Algorithms: In-Depth using Python
Loại khoá học: Programming Languages
Implement Data Structures and Algorithms in Python
Mô tả
This course will help you in better understanding of the basics of Data Structures and how algorithms are implemented in high-level programming language. This course consists of lectures on data structures and algorithms which covers the computer science theory + implementation of data structures in python language. This course will also help students to face interviews at the top technology companies. This course is like having personal tutors to teach you about data structures and algorithms.
There’s tons of concepts and content in this course. To begin the course:
We have a discussion of why we need data structures.
Then we move on to discuss Analysis of Algorithms ie Time and Space complexity, though the Asymptotic Notation ie Big O, Omega and Theta are taken up at the end of this course so that you do not get confused and concentrate on understanding the concepts of data structures.
We have a programming environment setup to make sure you have all the software you need in order to get the hands-on experience in implementing Data structures and algorithms.
Then we get to the essence of the course; algorithms and data structures. Each of the specific algorithms and data structures is divided into two sections. Theory lectures and implementation of those concepts in Python. We then move on to learn:
Recursion
Stacks, Queues, Deques
Linked List
Trees & Binary Trees
Binary Search Trees
Priority Queues and Heaps
Graphs & Graph Traversal Algorithms
Searching and Sorting algorithms
Again, each of these sections includes theory lectures covering data structures & their Abstract Data Types and/or algorithms. Plus the implementation of these topics in Python.
Bạn sẽ học được gì
Learn Data Structures, Abstract Data Types and their implementation in Python
Implementation of Searching Algorithms in Python
Implementation of Stacks, Queues, Linked List, Binary Trees, Heaps and Graphs in Python
Implementation of Binary Tree Traversal Techniques in Python
Graph traversals techniques ie Depth First Search and Breadth-First Search in Python
Implementation of Sorting Algorithms in Python
Enhance Analytical Skill and efficiently use searching and sorting algorithms in real applications
Yêu cầu
- Prior knowledge of Programming any high level language
- Basic knowledge of Python Programming
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
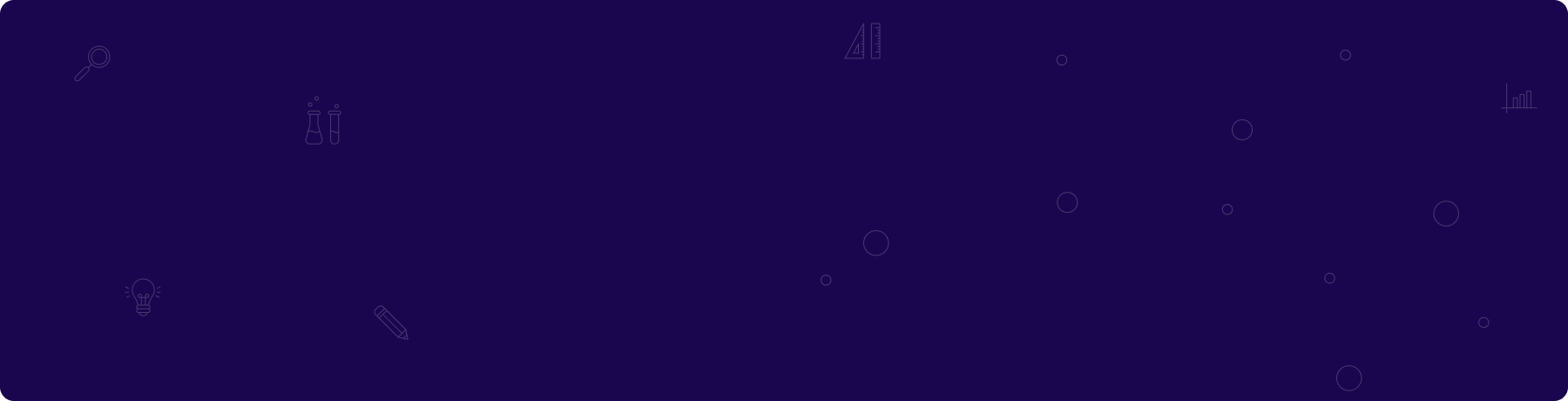
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng