Entity Framework Core - A Full Tour
Loại khoá học: IT Certifications
Learn how to use and take advantage of the full feature set of Entity Framework Core in your .NET Core applications.
Mô tả
Overview
In this course, Entity Framework Core - A Full Tour, you will learn to work with data in your .NET applications.
When courses are created for .NET technologies, the details of Entity Framework and its sheer power are often neglected. We get distracted with abstractions and layers and need to focus on what Entity Framework is doing and can do.
In this course, we will review the general benefits of using Entity Framework Core, which is Microsoft’s flagship Object Relational Mapper (ORM), to relieve you of many concerns and challenges that come with this component of software development. We will also discover how EF Core translates classes and references to Database Models and Relationships.
We will learn how to write queries, update databases incrementally, roll back changes, and explore the myriad capabilities that Entity Framework Core affords us. This course is compatible with .NET 6 / .NET 7 / .NET 8.
When you finish this course, you’ll have the skills and knowledge of Entity Framework Core needed to easily interact with data and write queries for .NET Core applications.
By the end of watching this course, you'll be able to:
Construct a data model using code-first and database-first workflows.
Understand Entity Framework Commands for all operating systems.
Use migrations to manage database changes.
Apply Database validations and constraints.
Perform CRUD operations using LINQ.
Apply best practices with Entity Framework.
Extending Data Contexts
Understand how Change Tracking works.
Manage Database Structure using Fluent API
Handle One-To-One, One-To-Many and Many-To-Many Relationships
Entity Framework Core 6 New Features
PREREQUISITES
To take this course, you should have at least three months of experience programming in C#. If you need to strengthen your C# fundamentals, you can take my C# beginner course C# Console and Windows Forms Development with LINQ & ADO .NET
You can also explore Database Development, which you can look at in the Complete Microsoft SQL Server Database Design Masterclass course.
Content and Overview
To take this course, you will need to know C#. Even if you have little exposure to the .NET development stack, this course is beginner-friendly and has development tips.
This premium course is smartly broken up to highlight related activities based on each module in the application being built. We will also look at troubleshooting and debugging errors as we go along, implementing best practices, writing efficient logic, and understanding why developers do things the way they do. Your knowledge will grow, step by step, throughout the course, and you will be challenged to be the best you can be.
The course is complete with working files hosted on GitHub, including some files to make it easier for you to replicate the demonstrated code. You will be able to work alongside the author as you work through each lecture and will receive a verifiable certificate of completion upon finishing the course.
Clicking the Take This Course button could be the best step to increase your income and marketability quickly! Also, remember that if you think the course is not worth your spending, you have a full 30 days to get a no-questions-asked refund!
It's time to take action!
See you in the course!
Bạn sẽ học được gì
Entity Framework Core - In depth
Understand the differences between database-first and code-first workflows
Use migrations to manage changes to your database
Apply best practices with Entity Framework
Query data using LINQ (using query syntax and extension methods)
Use Fluent API To Manage Constraints and Design
Implement Database Relationships
Understand Change Tracking
Conduct RAW SQL Queries
Execute Stored procedures, Functions, View Queries
Yêu cầu
- Some C# Knowledge
- Some Database Development Knowledge
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
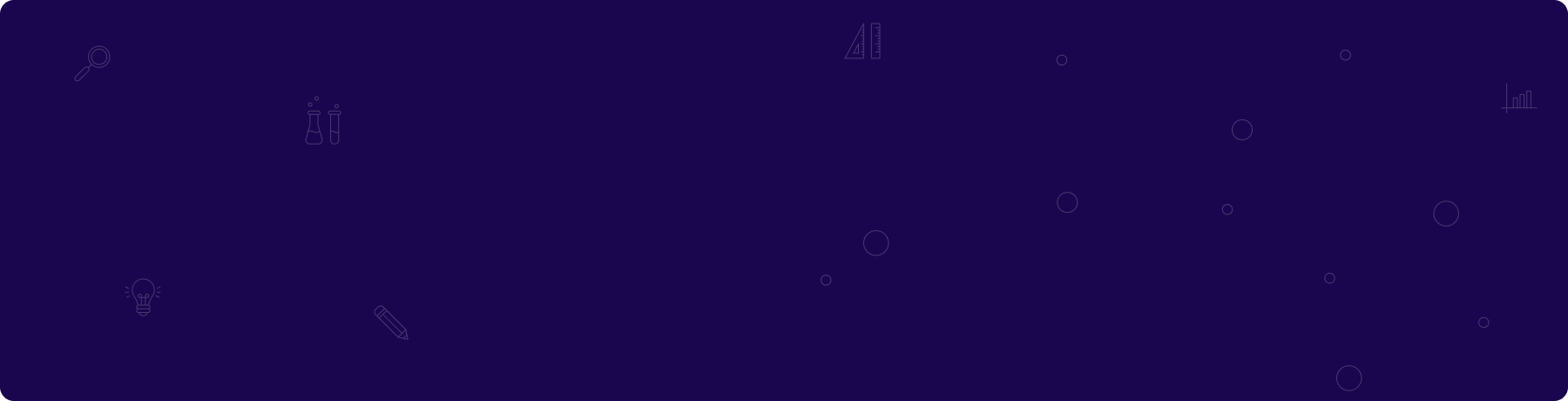
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng