Go Bootcamp: Master Golang with 1000+ Exercises and Projects
Loại khoá học: Programming Languages
Master and Deeply Understand Google's Go from Scratch with Illustrated In-Depth Tutorials & 1000+ Hands-On Exercises.
Mô tả
Go is a programming language created by Google, and this course is the most intuitive, in-depth, and highest-quality Go course on Udemy, with an insane level of attention to detail. You'll understand both the why and how. We've included thousands of animations, exercises, quizzes, examples, challenges, projects, and so on. By the end of the course, you'll become a confident Go programmer from scratch.
Why should you take this course now?
Watch ultra-detailed, entertaining, intuitive, and easy to understand illustrations and animations.
Solve 1000+ hands-on exercises (solutions are also included).
Create projects including a log parser, file scanner, spam masker, and more.
Learn Go programming tips and tricks that you can't find easily anywhere else.
Learn the Go internals and common Go idioms and best-practices.
Why should you learn Go (aka Golang and Go lang)?
Go is one of the most desired, easy to learn, and the highest paying programming languages. There are 1+ million Go programmers around the world, and the number is increasing each day exponentially. It's been used by Google, Facebook, Twitter, Uber, Docker, Kubernetes, Heroku, and many others.
Go is Efficient like C, C++, and Java, and Easy to use like Python and Javascript. It's Open-Source, Simple, Powerful, Efficient, Cross-Platform (OS X, Windows, Linux, ...), Compiled, Garbage-Collected, and Concurrent.
Go is best for Command-line Tools, Web APIs, Distributed Network Applications like Microservices, Database Engines, Big-Data Processing Pipelines, and so on.
What are you going to learn from this course (briefly)?
Go OOP: Interfaces and Methods
Internals of Methods and Interfaces
Functions and Pointers: Program design, pass by value, and addressability.
Implicit interface satisfaction
Type assertion and Type Switch
Empty interface: []interface{} vs interface{}
Value, Pointer, and Nil Receivers
Promoted Methods
Famous Interfaces
Tips about when to use interfaces
fmt.Stringer, sort.Sort, json.Marshaler, json.Unmarshaler, and so on.
Composite Types: Arrays, Slices, Maps, and Structs
Internals of Slices and Maps
Backing array, slice header, capacity, and map header
JSON encoding and decoding, field tags, embedding
Make, copy, full Slice expressions and append mechanics
UTF-8 encoding and decoding
Go Type System Mechanics
Type inference, underlying, predeclared, and unnamed types.
Untyped constants and iota.
Blank Identifier, short declaration, redeclaration, scopes, naming conventions
I/O
Process Command-Line Arguments, printf, working with files, bufio.Scanner, ...
How to create your own Go packages
How to run multiple Go files, and how to use third-party packages
Go tools
Debugging Go code, go doc, and others.
...and more.
Bạn sẽ học được gì
Learn from a Go Contributor
Learn Go Tips & Tricks that you can't find easily anywhere else
Go from a total Go beginner to a confident Go programmer
Practice Go with 1000+ Exercises (with included solutions)
Understand Go In-Depth with Animated Illustrations (Pass Interviews)
Learn the Go internals and common Go idioms and best-practices
Create a Log File Parser that parses log files
Create a Spam Masker that masks spammy words within a block of text
Create a command-line Retro Led Clock that shows time
Create Console Animations, Dictionary Programs, and more
Yêu cầu
- Access to a computer with an internet connection.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
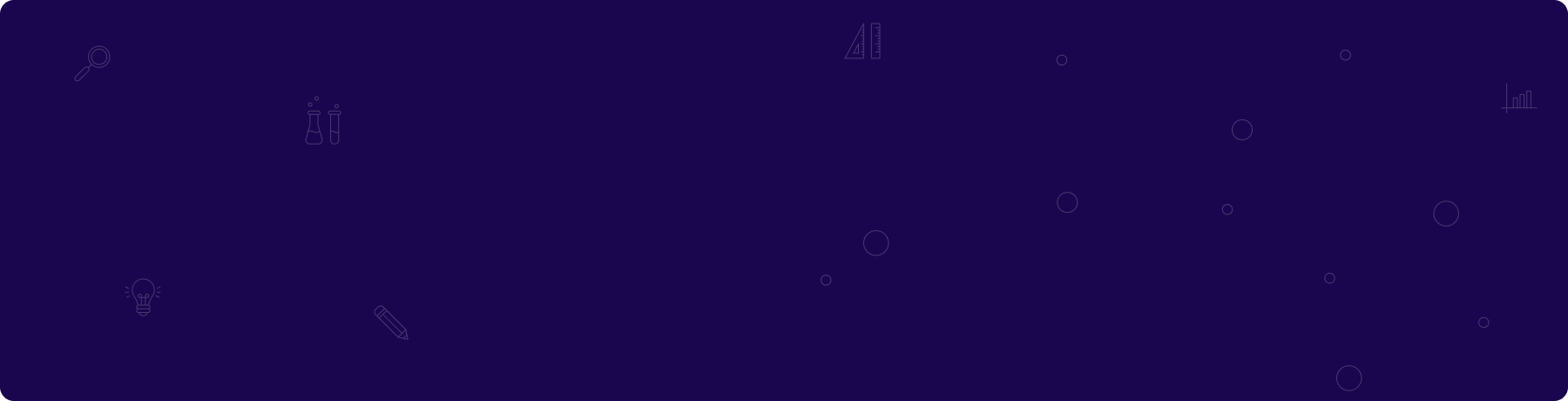
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng