gRPC Masterclass with Java & Spring Boot [2024 - Hands-On]
Loại khoá học: Software Engineering
Unlock the Power of gRPC for Scalable and Efficient API Design in Microservices Architecture
Mô tả
** Mastering gRPC: Building High-Performance Microservices with Protocol Buffers and Spring Boot **
In this comprehensive course, you will delve into the world of gRPC and learn how to leverage this cutting-edge technology to build high-performance and scalable APIs for your microservices. With gRPC, you can achieve up to 10 times the performance compared to traditional REST-based communication. You will discover the different types of gRPC APIs and understand how they can address common challenges in microservices communication.
By the end of this course, you will be comfortable developing microservices with gRPC, utilizing protocol buffers for data serialization, implementing load balancing strategies, handling authentication, managing errors, integrating gRPC with Spring Boot, and building multiple services that seamlessly interact with each other.
Course Content:
Protocol Buffers (Protobuf):
Learning Protobuf from scratch
Understanding Google's language-neutral and platform-neutral serialization format for structured data
Introduction to gRPC:
Understanding the benefits of gRPC for microservices communication
Exploring the different RPC types supported by gRPC
Unary API
Client Streaming API
Server Streaming API
Bidirectional Streaming API
Load Balancing:
Challenges and considerations in load balancing with gRPC
Exploring different load balancing strategies and their implementation with gRPC
Authentication:
Passing user session tokens for authentication in gRPC
Passing client service tokens for authorization in gRPC
Error Handling:
Error handling techniques in gRPC
Handling errors through metadata
Utilizing Protobuf OneOf for error handling
Error handling through exceptions
Spring Boot Integration:
Integrating gRPC with Spring Boot microservices
Developing multiple services and ensuring seamless integration between them
Best Practices and Real-World Considerations:
Discussing best practices for gRPC-based microservices development
Addressing real-world challenges and considerations in gRPC implementations
Join this course and unlock the power of gRPC to build highly performant, scalable, and efficient microservices using Protocol Buffers and Spring Boot. Gain the skills necessary to overcome common challenges in microservices communication, achieve superior performance, and streamline your API design with gRPC.
Bạn sẽ học được gì
Complete gRPC from scratch
10X Performance
Spring Boot Integration
Inter microservice communication
Unary, Client Streaming, Server Streaming & Bi Directional Streaming API
Load Balancing
Interceptors
Protocol Buffers / Protobuf
SSL / TLS
Metadata / Context / CallOptions
Yêu cầu
- Knowledge on Java 8 or above
- Comfortable with Indian Accent
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
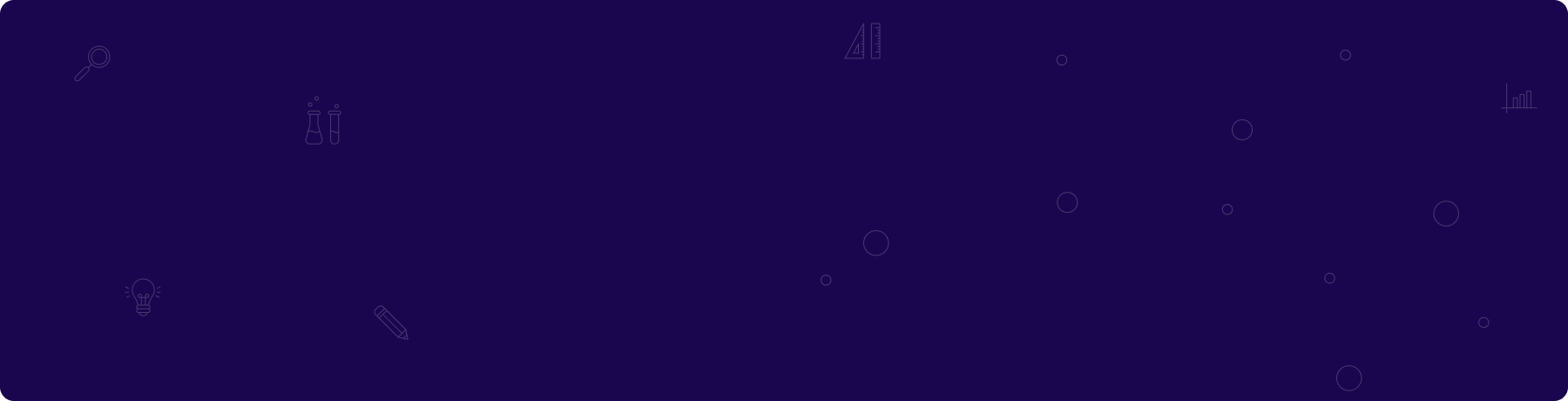
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng