Java Spring Framework 6 with Spring Boot 3
Loại khoá học: Other IT & Software
In this course, you will learn the highly demanded frameworks of enterprise world: Spring Framework 5 with Spring Boot
Mô tả
Spring 5 is a functional web framework for back-end development and is quite famous among Java developers when it comes to designing an enterprise-based application.
It consists of lots of modules and projects which makes it very huge.
The Spring Framework and Spring Boot enable developers to create high-performing, reusable, easily testable, and loose coupling enterprise Java applications.
It can be used to develop any Java application.
Knowledge of Spring framework has a huge demand in the enterprise market and Spring frameworks developers are paid handsomely.
Having Spring Framework on your resume will highlight you amongst other Java developer.
This course offers hands-on experience building Spring Framework applications using Spring Boot.
This course will be interactive and fun as I will code all the projects from scratch.
By taking this course you will have the latest skills that you need to build real applications using the Spring Framework.
Requirements for this Course:
Basic Java knowledge is required
Basic Knowledge of Servlet, JSP, and HTML is helpful
Knowledge of SQL and databases is helpful
Learn these super trending topics in Spring 5 and Spring Boot 2:
Spring Boot
Spring Core - IoC
Spring MVC
Spring AOP
Spring Data JPA
Spring REST
Spring Security
Who this course is for:
The course is for all Java developers: beginners to advanced who want to master Spring framework modules with Spring Boot
This learning path is for developers who wish to create their own web applications with Spring 5
This course is ideal for developers who wish to use the Spring Frameworks for enterprise application development
Bạn sẽ học được gì
Core Java
Spring Boot
Spring Core - IoC
Spring MVC
Spring AOP
Spring Data JPA
Spring REST
Spring Security
Yêu cầu
- Basic Java knowledge is required
- Basic Knowledge of Servlet, JSP, and HTML is helpful
- Knowledge of SQL and databases is helpful
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
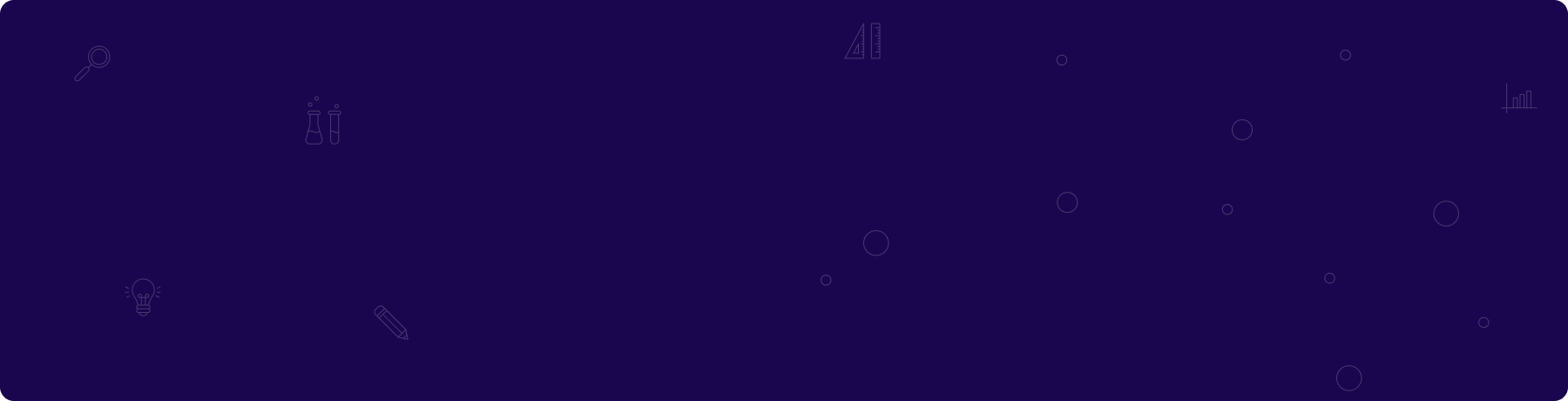
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng