Java Application Performance Tuning and Memory Management
Loại khoá học: Programming Languages
Discover how coding choices, benchmarking, performance tuning and memory management can optimize your Java applications
Mô tả
In this course we'll understand what can cause performance issues in our applications, and how to resolve them. This includes a review of some of the options available to us as developers at design-time - how to make good coding choices for optimal performance. For example, when should you pick an ArrayList over a LinkedList? How much difference does the StringBuilder really make? Is Lambda syntax more or less efficient at certain operations? We'll also learn about various ways that we can configure the virtual machine to provide better performance at run-time, with a range of runtime arguments. We'll also be diving deep into how the virtual machine manages memory, and how the garbage collection process works and impacts on application performance.
Along the way we'll be learning about the Just In Time compiler, performance testing and benchmarking, decompiling bytecode, using the GraalVM as an alternative virtual machine and more.
About Java Versions: This is the second iteration of this course and it is designed for all the current versions of Java that have long term support (Java 8 , Java 11 and Java 17). You can follow along with any of these versions. You can also use either the Oracle or the OpenJDK JVMs. For developers using other JVM languages (such as Kotlin, Scala and Groovy) all of the JVM configuration parts of this course will still be relevant, and some of the coding choices may be useful to consider also. (Note that there's even a review of whether Kotlin provides better or worse performance than Java!)
Bạn sẽ học được gì
What can cause performance issues in our applications, and how to resolve them.
The choices available to us as developers at design-time - how to make good coding choices for optimal performance.
How to configure the virtual machine to provide better performance at run-time.
The JVM's Just In Time compiler.
How the virtual machine manages memory.
Performance testing and benchmarking.
Yêu cầu
- Basic working knowledge of Java programming is needed, but you don't need to be a Java expert!
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
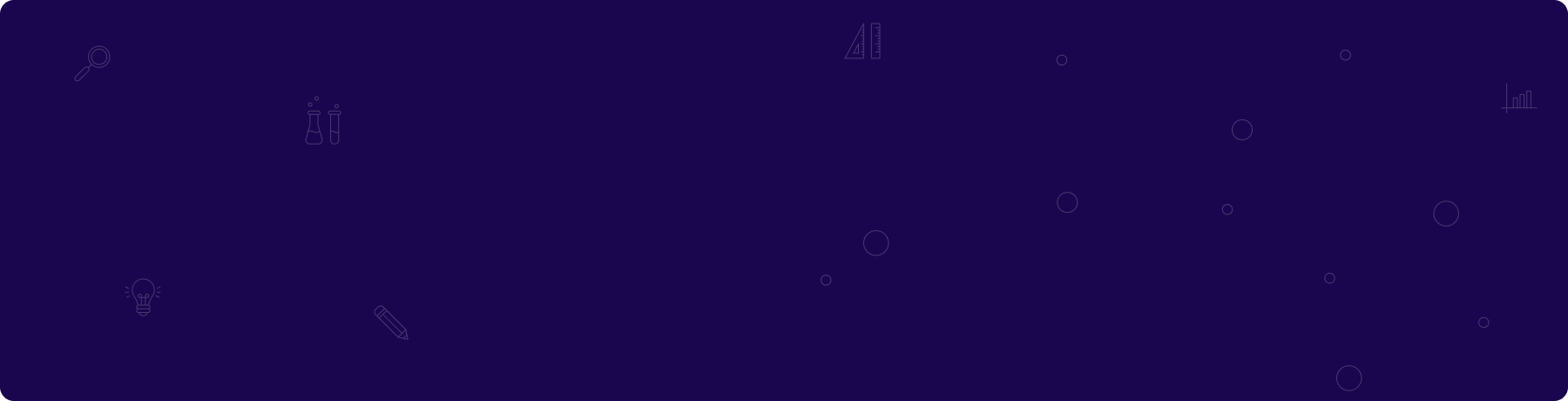
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng