JavaScript Algorithms and Data Structures Masterclass
Loại khoá học: Test Prep
The Missing Computer Science and Coding Interview Bootcamp
Mô tả
Updated in November 2018 with brand new section on Dynamic Programming!
This course crams months of computer science and interview prep material into 20 hours of video. The content is based directly on last semester of my in-person coding bootcamps, where my students go on to land 6-figure developer jobs. I cover the exact same computer science content that has helped my students ace interviews at huge companies like Google, Tesla, Amazon, and Facebook. Nothing is watered down for an online audience; this is the real deal :) We start with the basics and then eventually cover “advanced topics” that similar courses shy away from like Heaps, Graphs, and Dijkstra’s Shortest Path Algorithm.
I start by teaching you how to analyze your code’s time and space complexity using Big O notation. We cover the ins and outs of Recursion. We learn a 5-step approach to solving any difficult coding problem. We cover common programming patterns. We implement popular searching algorithms. We write 6 different sorting algorithms: Bubble, Selection, Insertion, Quick, Merge, and Radix Sort. Then, we switch gears and implement our own data structures from scratch, including linked lists, trees, heaps, hash tables, and graphs. We learn to traverse trees and graphs, and cover Dijkstra's Shortest Path Algorithm. The course also includes an entire section devoted to Dynamic Programming.
Here's why this course is worth your time:
It's interactive - I give you a chance to try every problem before I show you my solution.
Every single problem has a complete solution walkthrough video as well as accompanying solution file.
I cover helpful "tips and tricks" to solve common problems, but we also focus on building an approach to ANY problem.
It's full of animations and beautiful diagrams!
Are you looking to level-up your developer skills? Sign up today!
Bạn sẽ học được gì
Learn everything you need to ace difficult coding interviews
Master dozens of popular algorithms, including 6 sorting algorithms!
Implement 10+ data structures from scratch
Improve your problem solving skills and become a stronger developer
Yêu cầu
- Basic knowledge of JavaScript syntax
- NO experience with data structures or computer science needed!
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
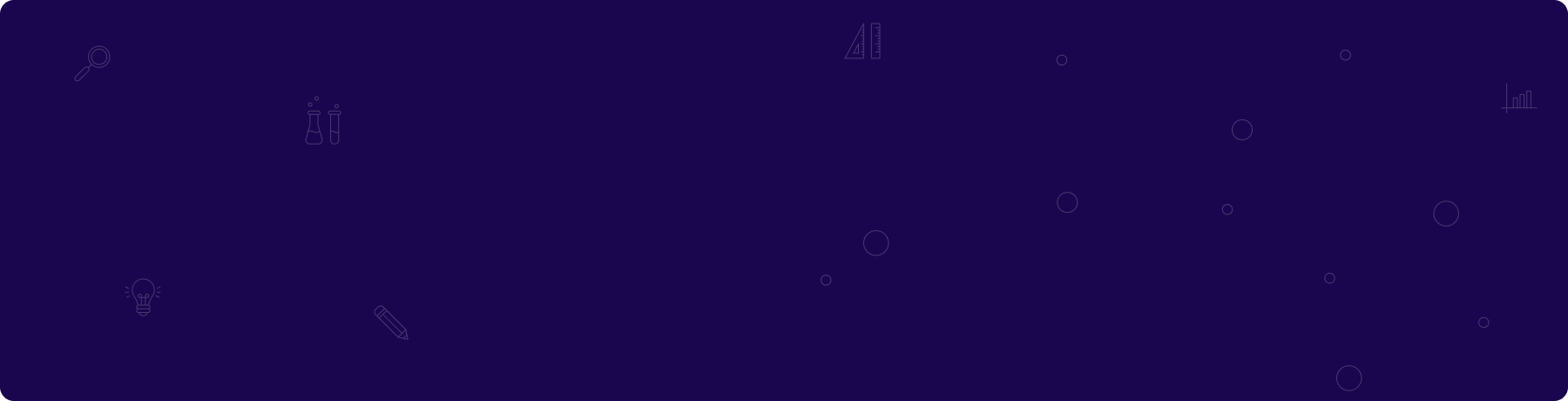
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng