JavaScript and TypeScript: The Complete Guide (Vite & Node)
Loại khoá học: Programming Languages
Fundamentals of Web Development with JavaScript and TypeScript
Mô tả
Welcome to JavaScript and TypeScript: The Complete Guide (Vite & Node.js)! In this course, you will learn the fundamental concepts of both JavaScript and TypeScript, two of the most popular programming languages for web development. You will start from the beginning and progress to build interactive and responsive web applications.
TypeScript is a powerful superset of JavaScript that adds strong typing and object-oriented features to the language. It allows you to write cleaner, more maintainable, and more efficient code and is quickly becoming the preferred choice for professional developers. In this course, you will learn how to leverage these features to write better code and build more robust web applications.
Throughout the course, you will learn how to use JavaScript and TypeScript to create modern web applications (both front-end and back-end) and gain practical experience through exercises and projects. You will also learn how to debug and troubleshoot code and master best practices and design patterns for web development.
Whether you are new to programming or an experienced developer looking to enhance your skills, this course is designed to help you master JavaScript and TypeScript and build web applications like a professional. By the end of the course, you will have a solid foundation in these languages, front-end (Vite.js), and back-end (Node.js), and be well on your way to becoming a competent web developer.
Bạn sẽ học được gì
Learn TypeScript without knowing TypeScript
Learn how to use JavaScript and TypeScript to create front-end and back-end applications with Node and Vite.
Become an expert in writing clean, maintainable and efficient code with JavaScript and TypeScript.
Gain hands-on experience in creating web applications through projects and practical exercises.
Learn Node js.
Learn TypeScript.
Learn JavaScript.
Learn Zod.
Learn Express js.
Learn Asynchronous Programming (Promises & Async/Await).
Learn about the Event Loop.
Yêu cầu
- To get the most out of this course, it is recommended that you have a basic understanding of pseudocode and algorithms, as well as some familiarity with HTML and CSS. This will help you understand the concepts and techniques taught in the course and apply them to real-world web development projects.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
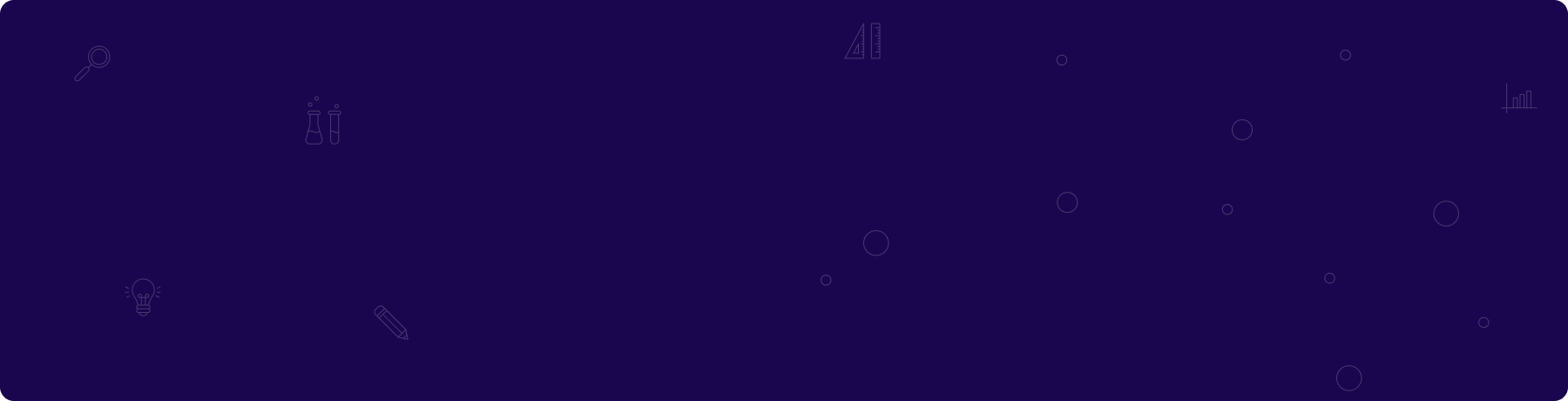
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng