Learn to build an e-commerce store with .Net, React & Redux
Loại khoá học: Web Development
How to build a real world application using some of the most popular and in demand frameworks, React and .Net
Mô tả
***The course has been refreshed in February 2023 to use .Net 7, React 18 and React Router 6***
Do you want to learn how to build a real world application using .Net, React and Redux? In this course we start from nothing and build a proof of concept E-Commerce store using these frameworks/libraries.
In this course we build a complete application from start to finish and every line of code is demonstrated and explained.
Here are some of the things you will learn about in this course:
Setting up the developer environment
Creating a .Net WebAPI application using the dotnet CLI
Creating a client side front-end React single page application for the stores user interface
Using Entity Framework to write code that queries and updates the database
Using ASP.NET Identity for login and registration
Using React Router to navigate between routes on the client
Using Automapper.
Building a great looking UI using Material Design
Making reusable form components using React hook form
Paging, Sorting, Searching and Filtering
Creating orders from the shopping basket
Accepting payments via Stripe using the new EU standards for 3D secure
Publishing the application to Heroku
Many more things as well
Tools you need for this course
In this course all the lessons are demonstrated using Visual Studio Code, a free cross platform code editor. You can of course use any IDE you like and any Operating system you like... as long as it's Windows, Linux or Mac.
Is this course for you?
This course is very practical, about 90%+ of the lessons will involve you coding along with me on this project. If you are the type of person who gets the most out of learning by doing, then this course is definitely for you.
Important: This course is aimed at beginners but there is an expectation you have written some code before - it is not suitable for those who have never coded before.
On this course we will build an example E-commerce store, completely from scratch using the DotNet CLI tool and the Create-React-App tool to help us get started. All you will need to get started is a computer with your favourite operating system, and a passion for learning how to build an application using .Net and React.
Bạn sẽ học được gì
Using .Net for the back end code
Using React for the client app or front-end
Using Redux for client side state management
Using the Material UI styling framework for React
Using the TypeScript language
Using the C# language
Using Entity Framework
Using ASPNETCore Identity for authentication
Yêu cầu
- Some programming experience required, but there is some pre-work you can do to get to the stage you need to be if never coded before
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
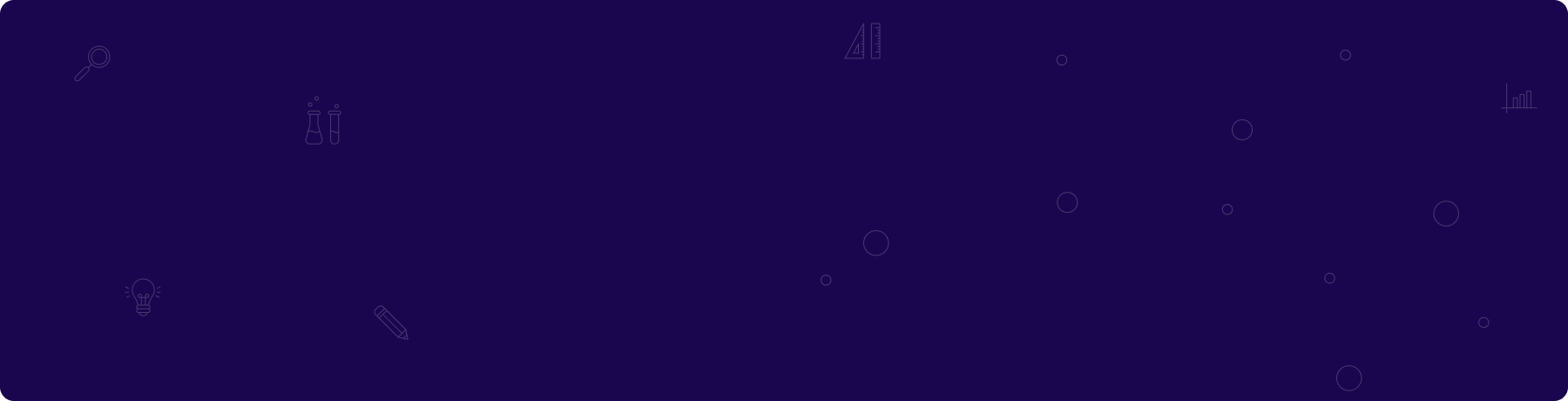
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng