Kotlin Coroutines and Flow for Android Development [2024]
Loại khoá học: Mobile Development
The Complete Guide! Get a deep understanding of Kotlin Coroutines and Flow to use them successfully in your Android Apps
Mô tả
Google recommends Kotlin Coroutines and Flow as the preferred solution for asynchronous programming on Android. Sooner or later, probably every Android developer will get in touch with these topics.
This course will give you a deep understanding of Kotlin Coroutines and Flow and show how to implement the most common use cases for Android applications.
This course consists of two big parts: The Coroutines part and the Flow part.
Before being able to use Flows in our applications, we first need a solid understanding of Coroutines. That’s why Coroutines are covered first. However, if you already have some experience with Coroutines, then you can also start with the Flow part right away, and jump back to lessons of the Coroutines part whenever needed.
In the part about Coroutines, first, we will take a detailed look at the advantages of Kotlin Coroutines over other approaches for asynchronous programming, like RxJava and Callbacks.
Then, we will talk about some theoretical fundamentals. These include:
Routines vs. Coroutines
Suspend Functions
Coroutines vs. Threads
Blocking vs. Suspending
Multithreaded Coroutines
Internal workings
Next, we will implement some of the most common use cases for Kotlin Coroutines in Android applications. These include:
Performing network requests with Retrofit sequentially and concurrently
Implementing Timeouts and Retries
Using Room with Coroutines
Performing background processing with Coroutines
Continuing Coroutine execution even when the user leaves the screen.
To improve your learning experience, this course also challenges you with several exercises.
Learning Coroutines can be overwhelming because you need to learn a lot of new concepts. However, we are going to start simple and as our use cases will get more and more complex, we will learn about new concepts step-by-step. These new concepts are:
Coroutine Builders (launch, async, runBlocking)
Coroutine Context
Coroutine Dispatchers
Structured Concurrency
Coroutine Scopes (viewModelScope, lifecycleScope, GlobalScope)
Jobs and SupervisorJobs
scoping functions (coroutineScope{} and supervisorScope{})
Cooperative Cancellation
Non-Cancellable Code
We will also make a deep dive into Exception Handling and discuss concepts like:
exception handling with try/catch
exception handling with CoroutineExceptionHandlers
when to use try/catch and when to use a CoroutineExceptionHandler
exception handling in Coroutines started with launch and async
exception handling specifics of scoping functions coroutineScope{} and supervisorScope{}
Unit Tests are very important for every codebase. In the course's final section, we will write unit tests for most of the coroutine-based use cases we implemented earlier. We will discuss concepts like
TestCoroutineDispatcher
creating a JUnit4 Rule for testing coroutine-based code
runBlockingTest{} Coroutine Builder
virtual time
Testing sequential and concurrent execution
TestCoroutineScope
In the part about Kotlin Flow, we first cover all the basics. We will answer the question “What is a Flow?” and then we discuss the benefits and drawbacks of reactive programming.
Afterward, we are going to have a look at different Flow builders and operators:
basic flow builders
terminal operators
terminal operator “launchIn()”
terminal operator “asLiveData()”
lifecycle operators
intermediate operators
In our first real Flow use case, we use a Flow to create a live stock-tracking feature, that uses all the available basic flow components.
In the next module, we will take a look at Exception Handling and Cancellation with Kotlin Flow.
In the following module, you will learn about StateFlow and SharedFlow and the following concepts:
how to make Coroutines lifecycle-aware with the “repeatOnLifecycle()” suspend function
Hot Flows VS Cold Flows
Converting Flows to SharedFlows with the “shareIn()” operator
Converting Flows to StateFlows with the “stateIn()” operator
When to use SharedFlow and when to use StateFlow
Next, you will learn about Channels, how they differ from hot flows, and when they are useful in Android Applications.
By the end of this course, you will have a fundamental understanding of Kotlin Coroutines and Flows and be able to write readable and maintainable, asynchronous, and multithreaded Android Applications.
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
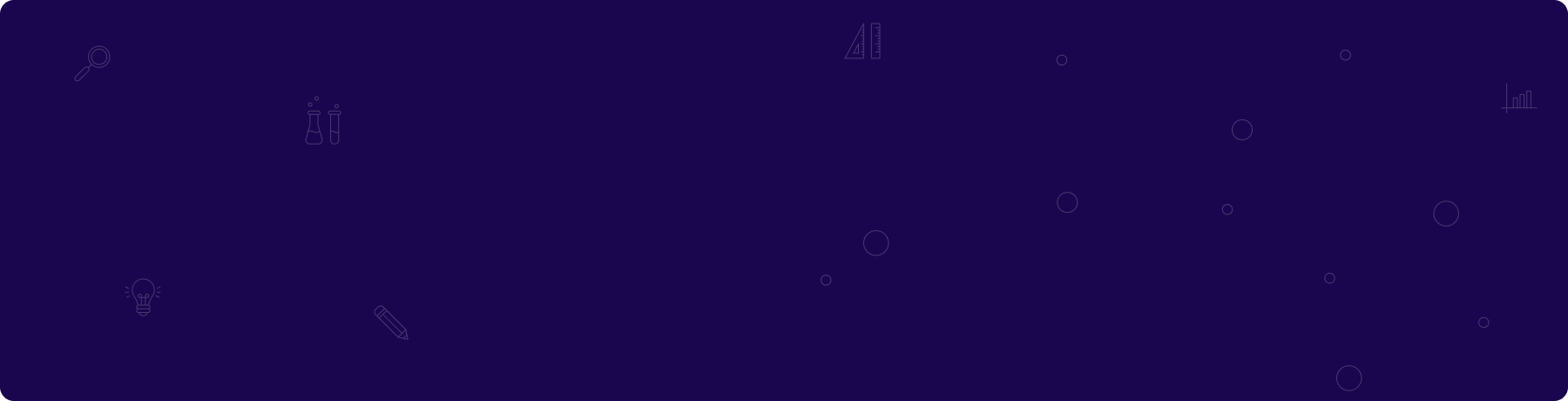
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng