Learn JavaScript: Full-Stack from Scratch
Loại khoá học: Programming Languages
Understand the JavaScript language itself, Node.js, MongoDB, The Web Browser and More To Create Meaningful Applications
Mô tả
Learn the incredibly popular and in demand JavaScript language. This course makes no assumptions of prior computer programming experience. We begin with the very basics and slowly but surely work our way up to writing JavaScript code to power every aspect of an application.
There are countless JavaScript courses in the world; here's what makes this one unique:
A strong emphasis on the "why" and not just the "how"
As few assumptions as possible; it's a pet peeve of mine when instructors assume I know something I don't
As few "just download my existing project to get you up and running" moments as possible. It's another pet peeve of mine when instructors have you use an existing solution that just "automagically" works and you miss a potential learning experience of setting it up yourself. We do copy-and-paste HTML templates (since the focus of the course is not about HTML) but aside from that I explain things from the ground up.
Here's what we'll learn in the course:
The JavaScript language itself
The Web Browser Environment
The Node.js environment
The MongoDB environment
The Express framework for creating servers
User registration & user-generated content
Authentication (both stateful with sessions and stateless with JSON Web Tokens)
... and much more!
I encourage you to watch the freely available first lesson titled "Where Do We Begin?" to get a better feel for the course.
This course may be brand new, but this isn’t my first time teaching. I’ve led training sessions for Fortune 500 companies and I’ve already helped over 65,000 people on Udemy and received the following feedback:
"Brad definitely has some of the best techniques to embed the lesson into your mind… hands down these are the best tutorials I have had the opportunity to view."
"Presentation is concise without being tedious… you honestly feel that you have a thorough understanding of the subject."
"…[Brad] explained the process. Not memorize this or that, he explained the process. If you're looking to take a course to understand the foundations of creating websites, look no further."
Become highly valuable and relevant to the companies that are hiring JavaScript developers; in one convenient place alongside one instructor. If you're ready to begin coding your own applications from the ground up - I'll see you on the inside!
Bạn sẽ học được gì
The JavaScript language itself
How to control a database (MongoDB) with JavaScript
How to control the Web Browser with JavaScript
How to implement user registration, log-in, log-out & user generated content
How to setup a server by using Node JS and Express
Yêu cầu
- No prerequisite knowledge required; the only thing you need is a computer (that you are allowed to install software on) and an internet connection.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
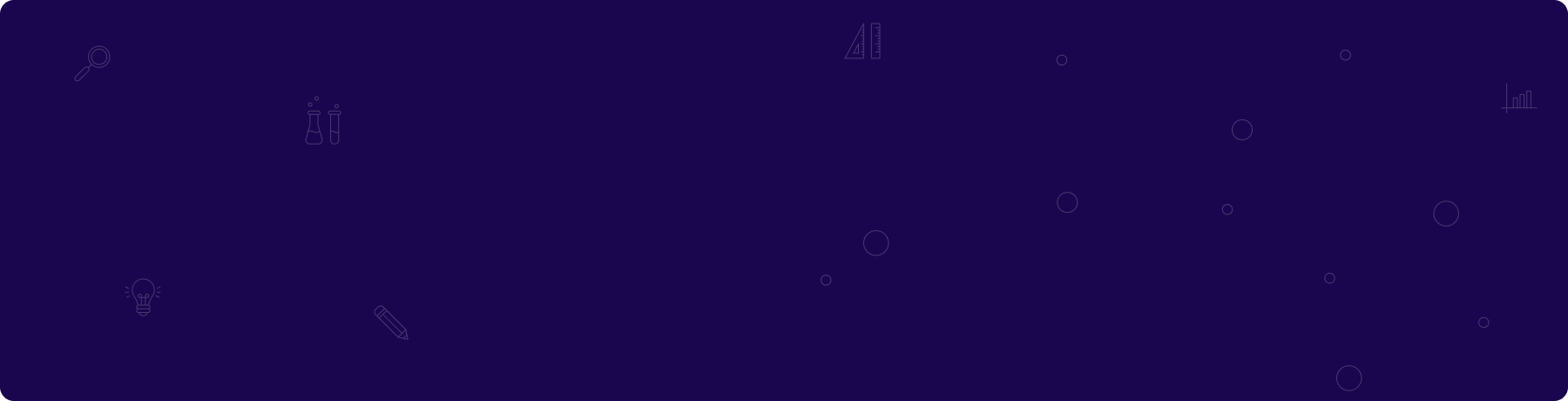
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng