Learn Python Programming - Beginner to Master
Loại khoá học: Programming Languages
Become a Python Expert. for Both Academics and Industry. 100+ Challenges
Mô tả
Learn Python Programming - course is curated for Beginner to Master.
Every topic is covered in depth with practical examples.
100+ Challenges to make you expert in Problem Solving using Python
By the end of the course you will understand Python extremely well and will be able to build your own Python applications.
Resources are available for every lectures.
Answer Quiz at the end of major topics, to feel confident.
Do Projects using Tkinter, GUI Programming.
Use Laptop or PC to learn and practice Python.
IDLE is used for demonstrating the concepts and PyCharm is used for Developing Programs. You can use any IDE, of your choice.
Course Content:
Fundamental Concepts and Features of Python
Learn to use PyCharm, Jupyter Notebook and IDLE.
Explore Numeric DataTypes
Conditional and Loop Statements
Explore Advance Datatypes - List, Tuple, Set, Dictionary
Write Error-free Programs by Handling Exception
Multithreaded Programs
More and more about Functions
Object-Oriented Programming
File Handling and CSV Files
Database Programming using Sqlite
Modules
Data Structures
Date and Time
OS
Math
NumPy
GUI Programming using Tkinter
Do Projects in GUI Programming
You can always ask Questions in Q&A section. you can find Q&A section under each video lecture.
Every Lecture contains notes in Resources.
Bạn sẽ học được gì
Master Python Programming by doing 100+ Challenges
Detail understanding of fundamentals
Build Multithreaded Applications
using Python for Database Programming
Build GUI Applications
Master art of Functional and Object-Oriented Programming
Learn Modules - DataStructure, OS, NumPy, Math, DateTime and Tkinter
Yêu cầu
- No Programming Experience Needed
- Laptop or PC with access to Internet
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
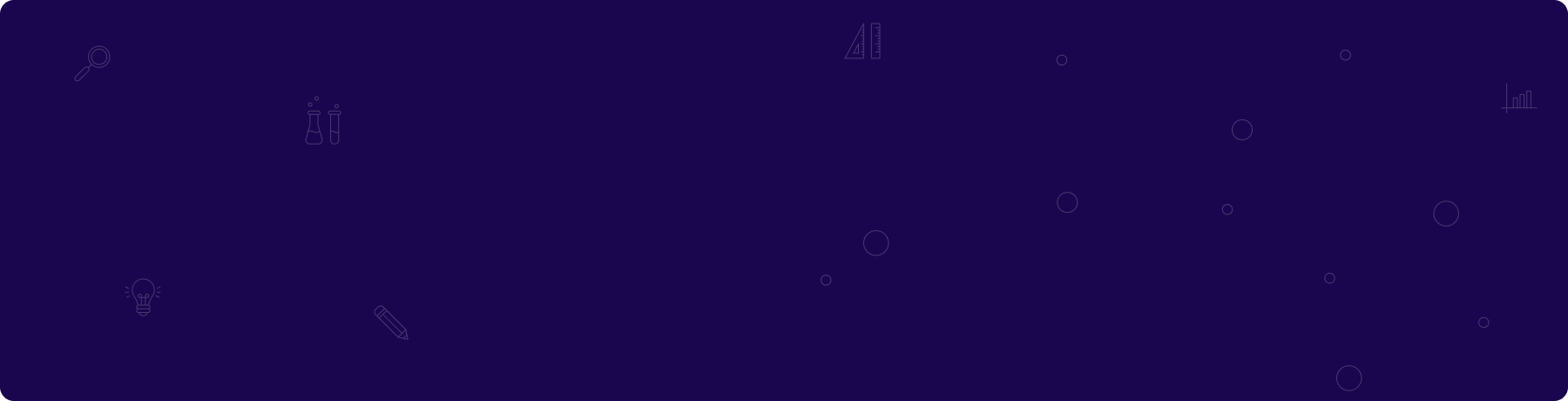
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng