Learn to build an e-commerce app with .Net Core and Angular
Loại khoá học: Other IT & Software
Build a proof of concept e-commerce store using Angular, .Net and Stripe for payment processing
Mô tả
*** Now updated to .Net 7.0 and Angular 15 as at January 2023***
Have you learnt the basics of ASP.NET Core and Angular? Not sure where to go next? This course should be able to help with that. In this course we start from nothing and build a proof of concept E-Commerce store using these frameworks.
In this course we build a complete application from start to finish and every line of code is demonstrated and explained.
Here are some of the things you will learn about in this course:
Setting up the developer environment
Creating a multi project .net core application using the dotnet CLI
Creating a client side front-end Angular UI for the store using the Angular CLI
Learn how to use the Repository, Unit of Work and specification pattern in .net core
Using multiple DbContext as context boundaries
Using ASP.NET Identity for login and registration
Using the angular modules to create lazy loaded routes.
Using Automapper in ASP.NET Core
Building a great looking UI using Bootstrap
Making reusable form components using Angular Reactive forms
Paging, Sorting, Searching and Filtering
Using Redis to store the shopping basket
Creating orders from the shopping basket
Accepting payments via Stripe using the new EU standards for 3D secure
Publishing the application to Linux
Many more things as well
Tools you need for this course
In this course all the lessons are demonstrated using Visual Studio Code, a free cross platform code editor. You can of course use any IDE you like and any Operating system you like... as long as it's Windows, Linux or Mac.
Is this course for you?
This course is very practical, about 90%+ of the lessons will involve you coding along with me on this project. If you are the type of person who gets the most out of learning by doing, then this course is definitely for you.
Important: If you have never coded before and you want to learn .Net and Angular you would be better starting with my other .Net Core and Angular course before this one.
On this course we will build an example E-commerce store, completely from scratch using the DotNet CLI and the Angular CLI to help us get started. All you will need to get started is a computer with your favourite operating system, and a passion for learning how to build an application using ASP.NET Core and Angular.
Bạn sẽ học được gì
.Net Core
Angular
C# Generics
Repository and Unit of Work Pattern
Specification Pattern
Caching
Angular Lazy loading
Angular Routing
Angular Reactive Forms
Angular Creating a MultiStep form wizard
Accepting payments using Stripe
Angular Re-usable form components
Angular validation and async validation
Yêu cầu
- 3-6 Months prior coding experience
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
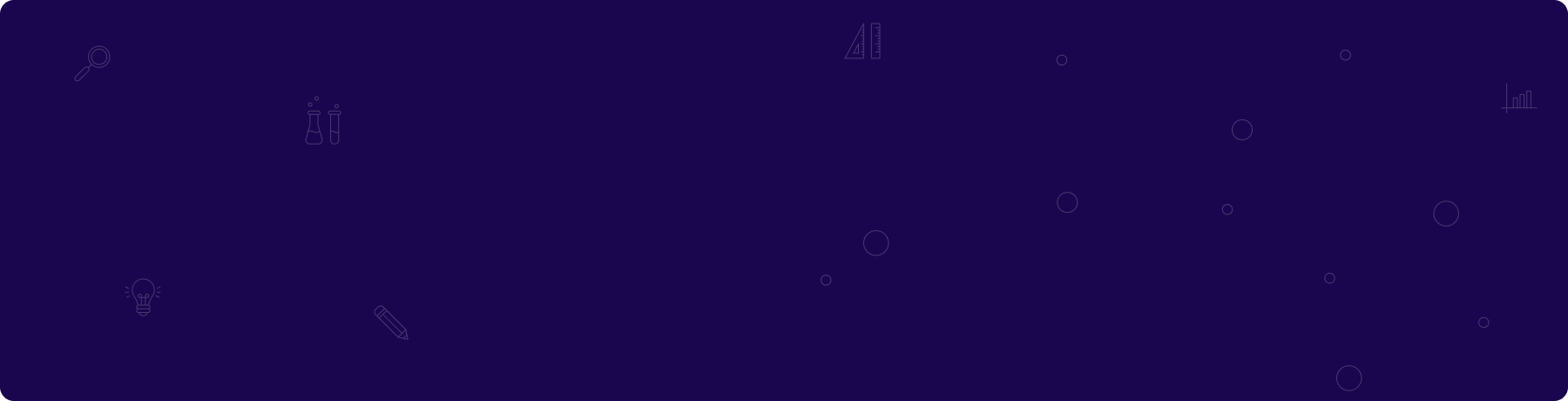
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng