Make a 2d Platformer in Unity 2020 using Design patterns
Loại khoá học: Web Development
Learn how to architect your codebase for a 2d platformer using design patterns.
Mô tả
Making games is fun but there is nothing more frustrating than adding new code to create a new game mechanic just to have the old code break. At this point it stops being fun and bug search begin - which honestly is the least fun thing to do when you could instead design a new level for your game. That is why it is so important to learn how to create maintainable and extensible code base for your game. If you search for a solution you will find Design patterns and SOLID principles. If you have every tried to learn design patterns you surely know that it is not intuitive when to use it. Each presents a solution but requires time and additional code to work. Implementing them just for the sake of it is just a waste of time - so when should we use them? The answer is - when adding new features or extending the old ones seems like a lot of work and effort.
In this course you will learn how to use state pattern as a base to create your character controller and how to refactor your code to other design patterns when you can see that adding new feature starts to be "painful" and unintuitive. At the end you will know the way of thinking behind the refactoring process and behind the decision when to use design patterns.
You will learn how to write decoupled classes - meaning that class A doesn't rely on Class B but if they both exist they can communicate witch each other to create our desired game mechanics.
This is an Intermediate course about creating a maintainable and extensible codebase and in effect a full 2d platformer game. You should know your way around Unity and feel comfortable coding in C# using Visual Studio IDE.
You will learn how to:
Create a character that can move and jump and extend it's character controller with new features like: climbing behaviour, attack logic etc.
How to reuse players character controller for enemies
How to create 3 types of enemies as well as a end level boss
How to use factor method pattern to make your code more maintainable
How to use Strategy pattern to implement simple AI system
Specific platformer features that we will implement:
picking up resources
respawn system
adding platforms with one-way collider
creating parallax effect for our level
melee and throwable weapons
and much more!
If for any reason you don't enjoy the course you have 30 days from the purchased date to get your money back - no questions asked.
I hope to see you in the course :)
-Peter
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
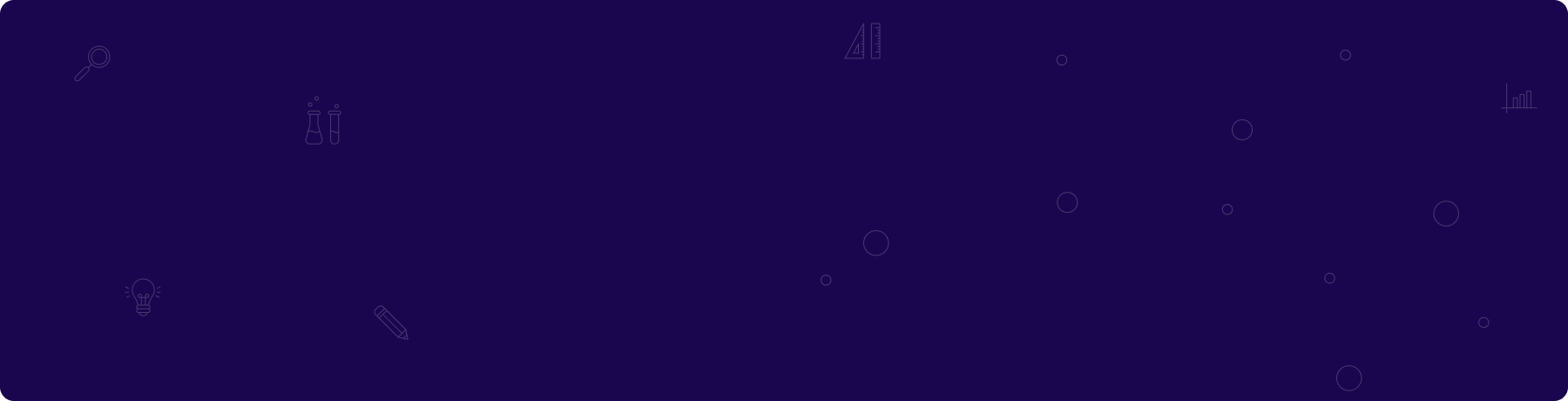
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng