Mastering Data Structures & Algorithms using C and C++
Loại khoá học: Programming Languages
Learn, Analyse and Implement Data Structure using C and C++. Learn Recursion and Sorting.
Mô tả
You may be new to Data Structure or you have already Studied and Implemented Data Structures but still you feel you need to learn more about Data Structure in detail so that it helps you solve challenging problems and used Data Structure efficiently.
This 53 hours of course covers each topic in greater details, every topic is covered on Whiteboard which will improve your Problem Solving and Analytical Skills. Every Data Structure is discussed, analysed and implemented with a Practical line-by-line coding.
Source code for all Programs is available for you to download
About Instructor
I am the Instructor of this course, I have been teaching this course to university students for a long period of time, I know the pulse of students very well, I know how to present the topic so that it’s easy to grasp for students.
I know how to use White board to explain the topic and also to make it memorable. Remembering the thing and using them in right place is more important than just understanding the topic.
After Completing Course
After completing this course you will be confident enough to take up any challenging problem in coding using Data Structures.
Course Contents
1. Recursion
2. Arrays Representation
3. Array ADT
4. Linked List
5. Stack
6. Queues
7. Trees
8. Binary Search Tree
9. AVL Trees
10. Graphs
11. Hashing Technique
Bạn sẽ học được gì
Learn various Popular Data Structures and their Algorithms.
Develop your Analytical skills on Data Structure and use then efficiently.
Learn Recursive Algorithms on Data Structures
Learn about various Sorting Algorithms
Implementation of Data Structures using C and C++
Yêu cầu
- Previous knowledge of Programming in C and C++
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
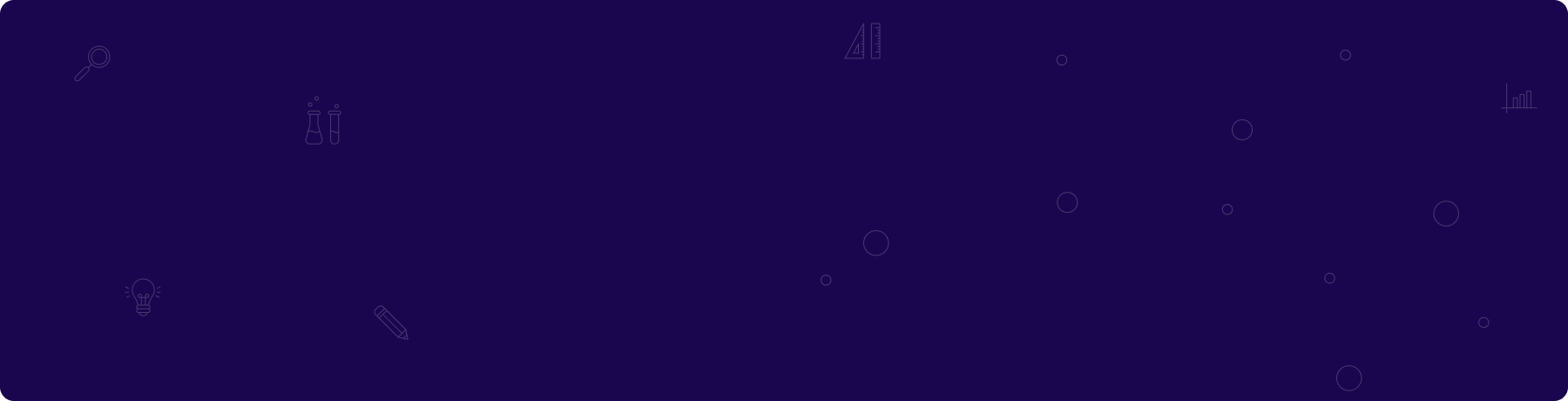
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng