[NEW] Full-Stack Java Development with Spring Boot 3 & React
Loại khoá học: Web Development
Build FULL-STACK Web Apps using Java, Spring Boot 3, Spring Data JPA, Spring Security, JWT, JavaScript, React JS & MySQL
Mô tả
In this hands-on project-oriented course, you will dive into the world of full-stack web app development using the powerful combination of Spring Boot and React JS.
In this course, you will build two full-stack web applications (Employee Management System and Todo Management App) using Spring Boot, Spring Security, Spring Data JPA, JWT, React JS, and MySQL database.
In this course, we will use the latest version of Spring Boot (3+), Spring Security (6+), React JS (18+), and MySQL database (8+). We will use modern and popular tools to build full-stack web applications such as IntelliJ IDEA, VS Code, Maven, Postman, NPM, etc.
What is React JS?
React JS is a JavaScript library used to build user interfaces (UI) on the front end.
React is not a framework (unlike Angular, which is more opinionated).
React is an open-source project created by Facebook.
What is Spring Boot?
Spring boot to develop REST web services and microservices.
Spring Boot has taken the Spring framework to the next level. It has drastically reduced the configuration and setup time required for spring projects.
You can set up a project with almost zero configuration and start building the things that actually matter to your application.
Course Topics:
1. React JS Fundamentals
2. Spring Boot Fundamentals
3. Project 1: Employee Management System
Build Employee Management Module - Backend Implementation using Spring Boot
Build Employee Management Module - Frontend Implementation using React JS
Build Department Management Module - Backend Implementation using Spring Boot
Build Department Management Module - Frontend Implementation using React JS
Style Web Pages using the Bootstrap CSS framework
4. Project 2: Todo Management App
Todo Management Module - Backend Implementation using Spring Boot
Todo Management Module - Frontend Implementation using React JS
Secure REST APIs using Spring Security
Build Register and Login REST APIs
Implement Register and Login Features in React App
Secure REST APIs using JWT (JSON Web Token)
Using JWT (JSON Web Token) in React App
Style Web Pages using the Bootstrap CSS framework
Tools and technologies used in this course:
Server-side:
Java 17+
Spring Boot 3+
Spring Data JPA (Hibernate)
Maven
IntelliJ IDEA
MySQL database
Postman
Client-side:
React JS 18+
React Hooks
React Router
Axios
Bootstrap CSS framework
Visual Studio Code IDE
VS Code extensions
Node JS
NPM
Bạn sẽ học được gì
Learn to develop a FULL-STACK Web Application with React and Spring Boot
Build Employee Management System FULL-STACK Web Application with React and Spring Boot
Build Todo Management FULL-STACK Web Application with React and Spring Boot
Learn Spring Boot Fundamentals and REST API Basics
Learn React JS Fundamentals
Building RESTful APIs with Spring Boot
Consuming RESTful APIs in React JS
Data Persistence with Spring Data JPA and Hibernate
Secure REST APIs using Spring Security
Build User Registration and Login Module
Secure REST APIs using JWT (JSON Web Token)
Using JWT (JSON Web Token) in React App
Style Web Pages using the Bootstrap CSS framework
Use Modern and Popular tools to build full-stack web applications such as IntelliJ IDEA, VS Code, Maven, Postman, NPM, etc.
Yêu cầu
- Java 17+
- Familiar with IntelliJ IDEA and VS Code
- Familiar with HTML, CSS and JavaScript
- No Spring Boot REST API experience is needed, You will learn building Spring Boot REST APIs from the scratch
- No React JS experience is needed, I will teach React JS from the scratch
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
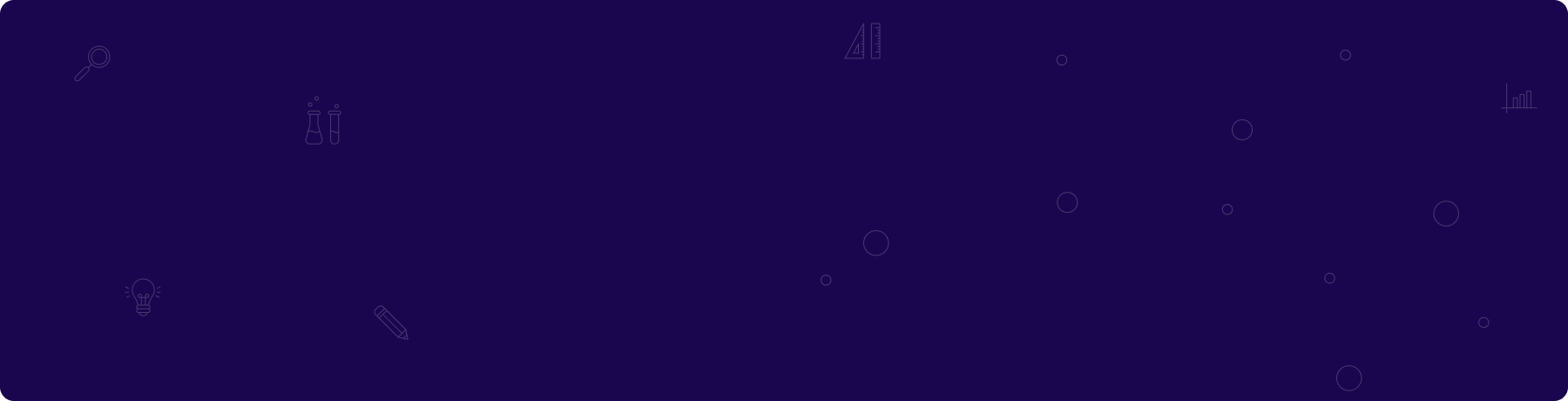
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng