Object Oriented Programming with C# - Beginner to Advanced
Loại khoá học: Web Development
Detailed step-by-step guide to Classes, Interfaces and many other OOP Concepts - Object-Oriented Programming Concepts
Mô tả
This course will lay the foundations of Object Oriented Programming (OOP) in your mind, allowing you to progress to more systematic and cleaner Programming methods.
The course is aimed at students who have at least some coding experience, preferably with C# (but Java or any other similar language is also acceptable).
There are so many things that you will learn in this course, some of the most noted ones are:
- What is an object and a class
- What is object oriented programming
- Class Constructors
- Namespaces
- Should you or should you not use the "this" keyword
- Fields and Properties
- Different ways in which Properties can be used
- Const and Readonly constants, the difference between them and how to use them
- Static Fields and Static (Singleton) Classes
- Enumerations
- Access Modifiers
- The 4 Pillars of Object Oriented Programming - Inheritance, Abstraction, Encapsulation, Polymorphism
- Many, many quality code guidelines
Each of these topics will be looked upon in great detail, and each of them are accompanied by a variety of Exercises. Practice makes perfect.
There are tons and tons of tutorials on Youtube, so why would you have to pay for this course?
The answer is very simple, in this course you will get every single lecture systemized in such way, that it ensures smooth transition between the previous and the following topic. Guaranteeing great learning experience.
There are no stones left unturned, everything is explained in great detail (but not too much, that would be boring :) )
The video lectures in the course are produced with the highest possible audio and video quality. No static noises to disturb you while you watch the videos, no blurry images, everything is crystal clear with crisp audio!
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
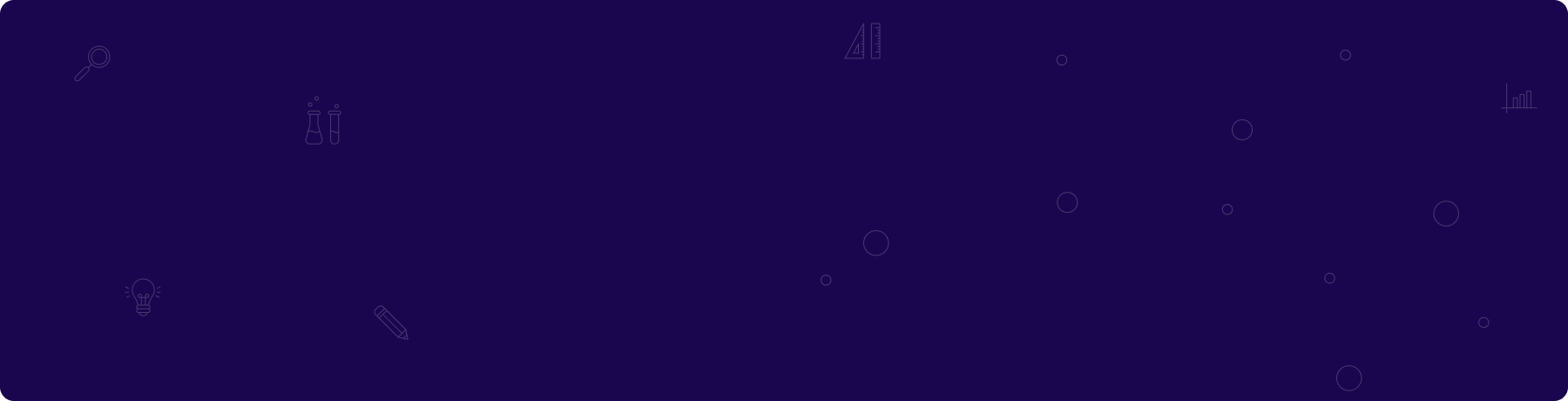
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng