Python Foundations for Data Analysis & Business Intelligence
Loại khoá học: Other IT & Software
Master the building blocks of base Python for data analysis & BI, with hands-on demos & unique, real-world projects!
Mô tả
This is a hands-on, project-based course designed to help you master the core building blocks of Python for data analysis and business intelligence.
We'll start by introducing the Python language and ecosystem, installing Anaconda and Jupyter Notebooks where we'll write our first lines of code, and reviewing key Python data types and properties.
From there we'll dive into foundational tools like variables, numeric and string operators, loops, custom functions, and more. You'll learn how to create and manipulate raw data, define conditional logic, loop through iterables or indices, and extract values stored in a wide variety of data types including dictionaries, lists, tuples, and more.
Throughout the course you'll play the role of a Data Analytics Intern for Maven Ski Shop, the world's #1 store for skis, snowboards and winter gear. Using the skills you learn throughout the course, you'll help the Maven team track inventory, pricing, and sales performance using your Python skills.
COURSE OUTLINE:
Why Python for Analytics?
Introduce the Python analytics ecosystem and why it’s the programming tool of choice for many data analysts
Jupyter Notebooks
Install Anaconda and create your first Jupyter Notebook, a user-friendly Python coding environment designed for data analysis
Python Data Types
Introduce native Python data types, common use cases, type conversion methods, and key concepts like iteration and mutability
Variables
Learn how to name and store values in memory using variables, as well as how to overwrite, delete and track them
Numeric Data
Learn how to work with numeric data, and use numeric functions to perform a range of arithmetic operations
Strings
Learn how to manipulate text via indexing and slicing, calculate string lengths, apply various string methods, and print f-strings to include variables
Conditional Logic
Learn how to use IF statements and Boolean operators to establish conditional logic and control the flow of your programs
Sequence Data Types
Learn how to create, modify, and nest lists, tuples, and ranges, all of which allow you to store many values within a single variable
Loops
Understand the logic behind For and While loops and learn how to refine loop logic and handle common errors
Dictionaries & Sets
Address the limitations of working with lists and explore common scenarios for using dictionaries and sets in their place
Functions
Learn how to create custom functions in Python to boost productivity, and how to import external functions stored in modules or packages
Manipulating Excel Sheets
Import the openpyxl package and manipulate data from an Excel worksheet using the Python skills you’ve learned throughout the course
Final Project
Import and manipulate data from an Excel workbook
Join today and get immediate, lifetime access to the following:
11+ hours of high-quality video
Python Foundations PDF ebook (300+ pages)
Downloadable project files & solutions
Expert support and Q&A forum
30-day money-back guarantee
If you're an Analyst, Data Scientist, or Business Intelligence professional looking to build a strong Python foundation and add powerful skills to your resume, this is the course for you!
Happy learning!
-Chris Bruehl (Python Expert & Lead Instructor, Maven Analytics)
__________
Looking for our full business intelligence stack? Search for "Maven Analytics" to browse our full course library, including Excel, Power BI, MySQL, Tableau and Machine Learning courses!
See why our courses are among the TOP-RATED on Udemy:
"Some of the BEST courses I've ever taken. I've studied several programming languages, Excel, VBA and web dev, and Maven is among the very best I've seen!" Russ C.
"This is my fourth course from Maven Analytics and my fourth 5-star review, so I'm running out of things to say. I wish Maven was in my life earlier!" Tatsiana M.
"Maven Analytics should become the new standard for all courses taught on Udemy!" Jonah M.
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
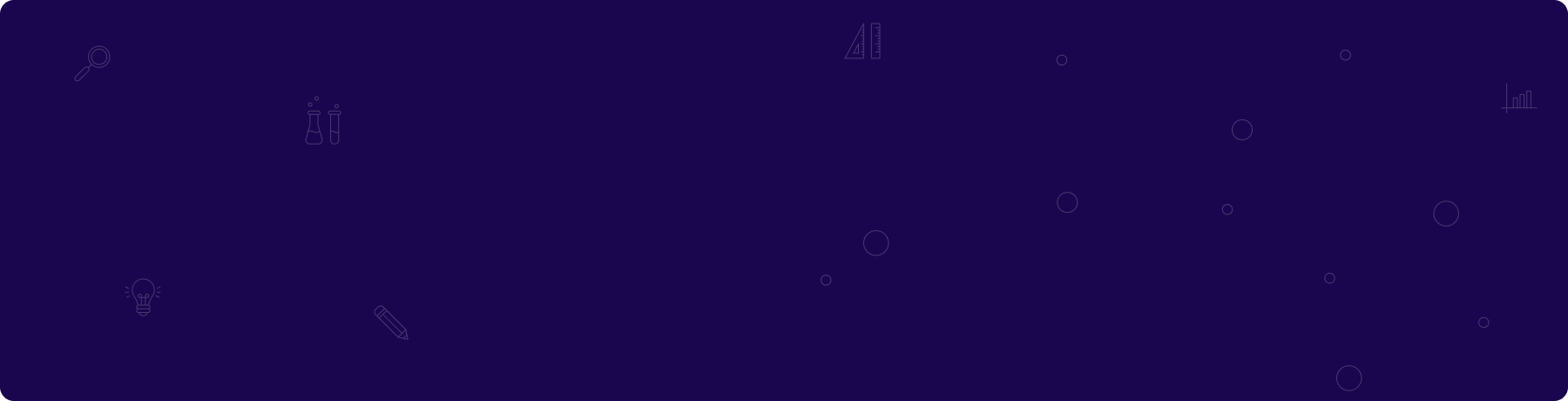
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng