Python Programming Master Course (2022)
Loại khoá học: Other IT & Software
Hone your programming skills by learning Python3 - master data structures, OOPS, problem solving and project building!
Mô tả
Welcome to Python Programming Master Course (2021) - the most comprehensive & robust Python3 Course by Coding Minutes designed for absolute beginners & developers!
Python is the hottest programming language of the 21st century. It is widely in Machine Learning & Data Science. Many top companies like Instagram, Spotify, Netflix, Google, Dropbox use python extensively for their projects which includes building web apps, data pipelines, data analysis & visualisation, deep learning at scale and large scale distributed systems.
This is the most comprehensive and detailed course for the Python programming language taught by Mohit Uniyal who is a data scientist turned instructor & Prateek Narang, a Google Engineer & algorithms instructor! Both the instructors have taught thousands of students in the last few years and have received amazing reviews. Whether you have never programmed before, already know basic syntax, or want to learn about the advanced features of Python, this course is for you!
With over 200+ lectures and more than 18 hours of video, this course ensures you build a solid foundation in Python. This course includes quizzes, mock tests, coding exercises and practical projects - URL Shortener & Text Generation.
This is a completely hands-on course, with interactive videos & coding in Jupyter Notebooks. You get the complete code repository to practice & revise on your machine.
We cover a wide variety of topics, including:
Installing Python
Different ways of running Python code
Python Fundamentals - Syntax
Operators and Expressions
Conditional Statements
For & while Loop
Lists
Tuples
Sets
Dictionaries
Functions
Arbitrary and Keyword arguments
Built-in Functions
File Handling
Error Handling
Modules & Packages
Object-Oriented Programming
Inheritance
Polymorphism
Iterators
Generators
Decorators
Algorithms Questions
and much more!
So what are you waiting for? Join Prateek & Mohit in this amazing journey, and start your journey of becoming a Python Champion today! Looking forward to see you in the course.
Bạn sẽ học được gì
Complete Python3 Programming
Logic Building & Problem Solving
Object Oriented Programming
Inheritance & Polymorphism
Intermediate - Advanced Python Concepts
Modules & Packages
File & Exception Handling
30+ Algorithmic Problems
Project - Markov Text Generation
Project - URL Shortner
TA Doubt Support
Yêu cầu
- No specific prerequisite for this course - this course beginner friendly!
- Passion to learn & excel, leave rest everything on us.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
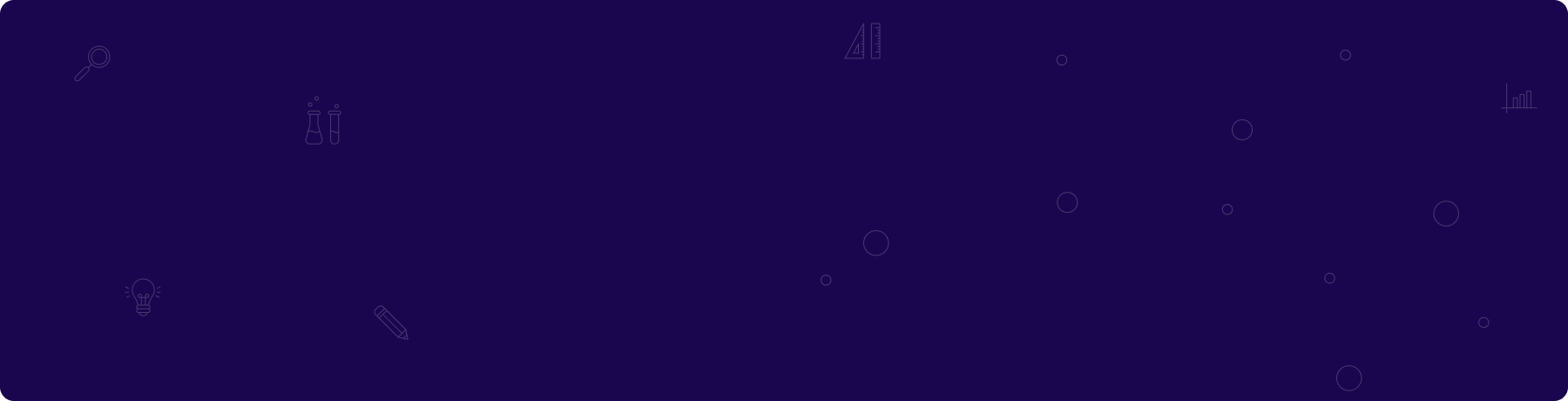
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng