Spring 6 & Spring Boot 3 for Beginners (Includes 6 Projects)
Loại khoá học: Software Engineering
Spring Framework Core 6, Spring Boot 3, Spring Security 6, REST API, Spring MVC, Spring WebFlux, JPA, Thymeleaf, Docker
Mô tả
In this course, you will learn Spring Framework Core 6, Spring Boot 3, REST API, Spring MVC, WebFlux, Spring Security, Spring Data JPA, Docker, Thymeleaf, IntelliJ IDEA, Maven, and Building Projects
No Spring framework experience is needed, I will teach you all the Spring framework core features so that you will understand Spring Boot in-depth and how it works behind the scenes.
What will you learn?
- Learn Spring IOC Container with Examples
- Learn Java-based configuration with Examples
- Learn Annotation-based configuration with Examples
- Learn Spring core annotations with Examples
- Learn Dependency Injection
- Learn Constructor, Setter, and Field Dependency Injection
- Learn Spring beans scopes, life cycle, and annotations
- Build REST APIs using Spring Boot
- Learn Spring Data JPA fundamentals
- Build User Management Project using Spring boot, Spring Data JPA, and MySQL database.
- Using DTO (Data Transfer Object) Pattern
- Using Mapping Libraries to Map Entity to DTO and Vice Versa
- Exception Handling in Spring Boot Application
- Validation in Spring Boot Application
- Spring Boot Actuator (Production-Ready Feature)
- Transaction Management with Spring Boot and Spring Data JPA
- Build Real-Time Search REST API
- Build Reactive CRUD REST APIs using Spring WebFlux and Reactive MongoDB
- Integration Tests for Reactive CRUD REST APIs
- Learn Spring MVC Concepts
- Learn Thymeleaf Fundamentals (Thymeleaf Crash Course)
- Learn to build a REAL-TIME web application (Student Management System) using Spring MVC, Spring boot, Spring Data JPA, Thymeleaf, and MySQL database.
- Learn Form and Bean Validations using Hibernate Validator
- Learn how to use Bootstrap CSS 5 Framework for styling web pages.
- Learn how to connect Spring boot application with MySQL database
- Learn how to use three-layer architecture - controller, service, and repository/DAO layers.
- Learn how to Create JPA entities
- Learn how to create Spring Data JPA repositories for JPA Entities
- Learn how to secure Web applications using Spring Security
- Learn how to use Spring Security's Authentication and Authorization
- Learn how to implement Registration, Login, and Logout features
- Dockering Spring Boot Application Step by Step
- Dockering Spring Boot MySQL CRUD Application Step by Step
- Dockering Spring Boot MySQL CRUD Application Step by Step using Docker Compose
- 25+ Spring and Spring Annotations for Interviews
What is Spring Boot?
Spring Boot is basically an extension of the Spring framework which eliminated the boilerplate configurations required for setting up a Spring application.
Spring Boot is an opinionated framework that helps developers build Spring-based applications quickly and easily. The main goal of Spring Boot is to quickly create Spring-based applications without requiring developers to write the same boilerplate configuration again and again.
What is Spring MVC?
Spring MVC is a popular module in Spring Framework and it is used to develop web applications as well as RESTful web services.
Spring MVC is called a web framework because it provides all the required components to develop a complete web application.
The Spring MVC framework provides Model-View-Controller (MVC) architecture and ready components that can be used to develop flexible and loosely coupled web applications
What is Thymeleaf?
Thymeleaf is a modern server-side Java template engine for both web and standalone environments, capable of processing HTML, XML, JavaScript, CSS, and even plain text.
The main goal of Thymeleaf is to provide an elegant and highly-maintainable way of creating templates.
It's commonly used to generate HTML views for web applications.
Thymeleaf is a very popular choice for building UI so we will be using Thymeleaf to build the view layer in the Spring MVC web application (Blog App).
Technologies and Tools Used:
- Java 17
- Spring Boot 3
- Spring Framework 6
- Spring MVC 6
- Thymeleaf
- Bootstrap CSS 5 Framework
- Spring Security 6
- Spring Data JPA
- Hibernate Framework 6
- Spring WebFlux
- MySQL Database
- Maven
- IntelliJ IDEA
- Docker
Some Amazing Reviews from the Students for this Course:
" You are the best tutor in my life till now, and you give so much brightness for students life."
" I strongly recommend this course for beginners. I thoroughly enjoyed this course. Good support by the trainer for doubt resolving."
" If you are new to programming you must buy Ramesh's courses because he explains and repeats everything in the learning process and he uses not outdated technologies as others. This is really amazing for learning. I know what I'm talking about, I bought all the top Spring courses, I can compare it. A big plus of Ramesh cources is, if you have any questions, Ramesh himself will answer them very quickly. Don't hesitate."
"I have like 4-5 courses in spring / spring boot. I think, this one is best one to start with. Because others I cant understand much, get tired of them, cant go so deep trying to understand what is going on. But this Course, goes step by step, you understand concepts., you practise them. And there is no gaps that you have to figure yourself. I think very beginner friendly! I suggest everyone"
"Excellent Explanation and great knowledge instructor. one of the best course on udemy."
"Beginner Friendly Course, Instructor Knows What's He Teaching Very Well."
"A perfect course to gain lots of knowledge and hands-on experience on spring framework & It's deep and detailed (+ beginners friendly). Thoroughly enjoyed the course and looking forward to the next course."
"Just started the course, the spring core concepts spring ioc container and dependency injection is nicely explained with examples. Amazing course and recommended for beginners and professionals."
"Great course as usual from Ramesh, Springs 6 is the lastest version and this course has a lot of great information."
"Excellent course for both beginners and expert java developers who never used Spring. Strongly recommended!"
"Great work sir, This is really good course. Each and Every module of this course is helpful. If anyone at beginner level or know little bit about spring and spring boot, I would say you should definitely purchase this course."
Bạn sẽ học được gì
Spring Framework Core Features - Spring IOC Container, Java-based configuration, Annotation-based configuration, Dependency Injection, etc
You will learn fundamentals of Spring Framework from zero, no previous experience required
Learn Spring Boot fundamentals and features - Spring Boot Internals, Auto Configuration, Spring Initializr and Starter Projects
Learn building Spring Boot REST APIs
Learn Spring Data JPA fundamentals
Build User Management Project using Spring Boot, Spring Data JPA, and MySQL database
Learn using DTO Pattern, Mapping Libraries
Learn Exception Handling, Validation, Spring Boot Actuator, Transaction Management in Spring Boot App
Build Todo Management Project using Spring Boot 3, Spring Data JPA, IntelliJ IDEA, Maven and MySQL Database
Secure REST APIs using Spring Security 6, Spring Boot 3 and MySQL Database
Build Reactive CRUD REST APIs using Spring WebFlux and Reactive MongoDB
Learn how to write Integration Tests for Reactive CRUD REST APIs
Learn Spring MVC Concepts
Learn Thymeleaf Fundamentals (Thymeleaf Crash Course)
Learn to build a REAL-TIME web application (Student Management System) using Spring MVC, Spring boot, Spring Data JPA, Thymeleaf and MySQL database.
Learn How to Create Spring Data JPA Repositories for JPA Entities
Build Registration and Login System Web Application using Spring MVC, Spring boot, Spring Data JPA, Thymeleaf and MySQL database.
Dockering Spring Boot Application Step by Step
Dockering Spring Boot MySQL CRUD Application Step by Step
Dockering Spring Boot MySQL CRUD Application Step by Step using Docker Compose
25+ Spring and Spring Boot Annotations for Interviews
Yêu cầu
- Java
- No Spring framework experience is needed, I will teach you all the Spring framework core features
- No Spring Boot experience is needed, I will teach you everything about Spring boot and build the projects
- No Docker experience is needed, I will teach you how to docker Spring Boot Applications from the scratch
- It's great if you are little bit familiar using IntelliJ IDEA
- No Thymeleaf experience is needed, I will teach you Thymeleaf fundamentals to build Spring MVC web applications
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
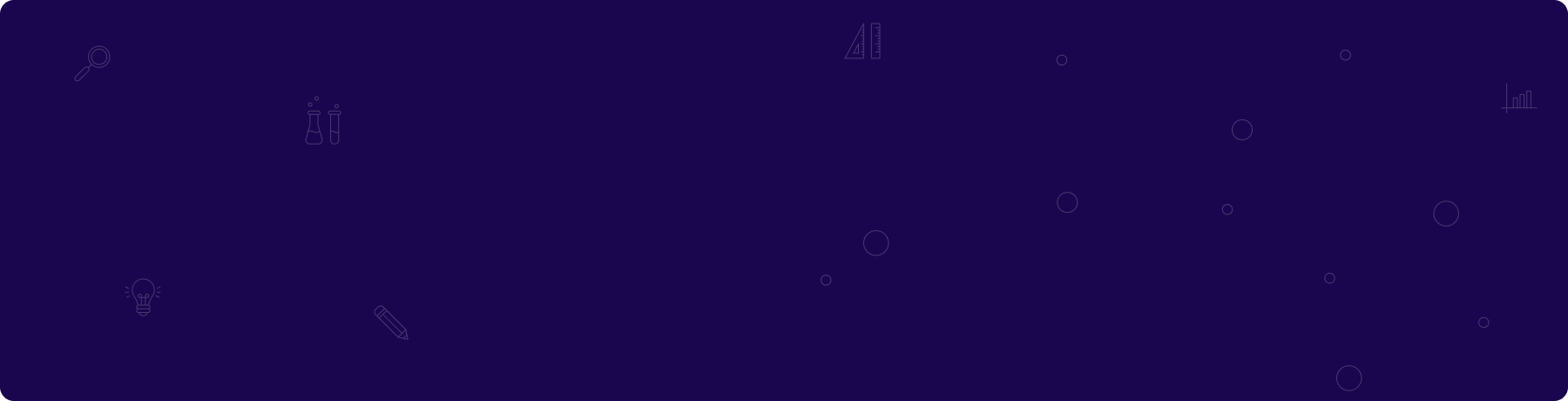
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng