Spring Boot Microservices and Spring Cloud. Build & Deploy.
Loại khoá học: Web Development
Spring Cloud Config Server, API Gateway, Eureka, Feign, Resilience4J, Load Balancing, Spring Security, ELK, Docker, AWS.
Mô tả
This video course is for Beginners who have never build RESTful Web Services and Microservices before. It will guide you step-by-step through basics and will help you create and run RESTful Microservices from scratch. You will learn how to run Microservices on your own developer's machine as well as in Docker Containers on AWS EC2 Linux machines.
By the end of this course, you will have your own RESTful Spring Boot Microservices built and running in Spring Cloud.
You will learn how to create and run your own:
RESTful Microservices,
Eureka Discovery Standalone Server,
Eureka Discovery Server Cluster,
Zuul API Gateway,
Spring Cloud API Gateway,
Load Balancer,
Spring Cloud Config Server,
You will learn to use:
Spring Cloud Bus and Rabbit MQ,
Spring Boot Actuator
You will also learn how to implement for your REST API features like:
User Authentication(Login) and,
User Authorization(Registration),
Role-based access control(RBAC)
You will learn to use:
Spring Security and JWT
You will learn how to use:
Spring Data JPA to store user details in a database,
H2 in-memory database and a database console,
MySQL database server,
Postman HTTP Client,
Spring Tool Suite,
Spring Initializer
This course also covers how to:
Trace HTTP Requests with Spring Cloud Sleuth and Zipkin(Distributed tracing)
Aggregate log files in one place(Centralized logging) with ELK stack(Logstash, Elasticsearch, Kibana).
You will also learn how to:
Start up AWS EC2 Linux machine,
Install Docker,
Create Docker images,
Run Microservices in Docker containers on multiple EC2 Linux machines in Amazon AWS Cloud.
Bạn sẽ học được gì
Build and run RESTful Microservices
Implement User Authentication
Eureka Discovery Service
Implement User Authorization with Spring Security and JWT
Spring Cloud API Gateway
Learn to use JPA to persist data into a Database
Use Cloud Cloud Config Server. Learn to Encrypt Sensitive Data.
Learn to install MySQL Server and persist data into MySQL
Spring Cloud Bus and Rabbit MQ
H2 in-memory database and H2 Console
Spring Boot Actuator
Learn to use HTTP Postman
Use Spring Security
Learn to use Spring Initializer
Distributed Tracing with Sleuth and Zipkin
Learn to use Spring Tool Suite
Centralized Logging with ELK Stack(Logstash, Elasticsearch, Kibana)
Run Microservices in Docker Containers
Use Method Level Security to protect API Endpoints
Deploy Microservices to AWS
Yêu cầu
- Know Java
- Mac computer
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
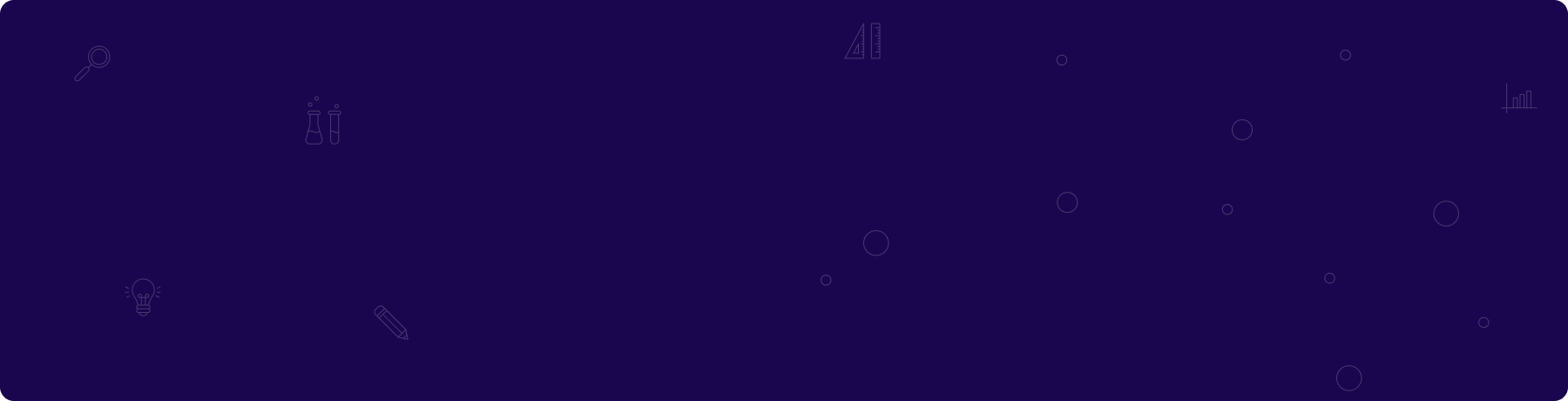
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng