Spring Boot REST APIs Ultimate Course
Loại khoá học: Web Development
Hands-on REST API Development with Spring Boot: Design, Implement, Document, Secure, Test, Consume RESTful APIs
Mô tả
Welcome to Spring Boot REST APIs Ultimate course!
My name is Nam Ha Minh. I’m the instructor who will lead you through this course. You know, I’ve been programming with Java for nearly 2 decades, since the days of Java 1.3 and 1.4. I’m a professional Java developer certified by Oracle.
I’m very glad that you’re interested in this course. Let me introduce exactly what this course is about, and everything you need to know before enrolling.
What this Course is Exactly About:
This is a comprehensive course about REST API development, from understanding REST API Core Concepts to API Design Best Practices and Implementing APIs with Spring Boot; from Securing APIs to Testing APIs; from Documenting APIs to Deploying APIs.
This course is also about development of different kinds of REST Client applications such as Web app (Spring MVC), React app, desktop app (Java Swing) and mobile app (Android).
What You Will Learn to Build:
Through this comprehensive course, you will learn to build Weather APIs Service using Spring Boot with MySQL Database.
Then you will learn to build a React application for managing weather data.
Use Spring MVC to build a web application for managing users and API clients.
And another Spring MVC application for a Weather Forecast website.
Then you will learn to build a sample mobile app that displays embedded weather information, using Android.
Finally, you will learn to build a Java desktop application with Swing, that demonstrates updating weather data from a weather station.
The React app, Weather Forecast website, Android app and Swing app are consumers of the Weather APIs Service.
Key technologies will be used:
You'll learn how to use the following technologies (not all mentioned):
- Back-end technologies: Spring framework, Spring Boot, Spring Data JPA, Spring Data REST, Spring REST Docs, Spring HATEOAS
- Thymeleaf as server-side template engine for Spring MVC application
- FasterXML/jackson Java JSON library
- Security technologies: Spring Security, Spring Security OAuth2, JSON Web Token (JWT) for Java and Android
- Database technologies: MySQL Relational database, Hibernate ORM framework
- Testing technologies: mockito - mocking framework for unit tests, JUnit testing framework
- Redis as caching solution
- Bucket4J as API Rate Limit solution
- REST Clients: React for Single Page Application, Android for mobile app, Java Swing for desktop app
- Front-end technologies: HTML, CSS, Javascript, Bootstrap, jQuery.
Main software programs & tools will be used:
To develop the applications in this course, you will need to use (not all mentioned): Java Development Kit (JDK), Spring Tool Suite IDE, MySQL Community server, MySQL Workbench, curl, Postman, Redis, Docker, Swagger online editor and codegen, Git, Heroku CLI, AWS CLI.
What you will get by finishing this course:
By completing this course, you will get comprehensive REST API expertise in Analysis, Design, Implement, Test, Secure, Deploy & Best Practices. In other words, you master all the techniques involved in REST API development.
You will also get Full-stack development expertise in Backend (Java and Spring), Frontend (React, Javascript, HTML, CSS), Database (MySQL), Deployment (Heroku, AWS), Version Control (Git).
More importantly, when you complete learning this course, I believe you will get Job–ready Skills, such as Technical competence, Self management, Accuracy and Attention to Detail, Professionalism that your employer will love, and Problem Solving skill which is important to succeed in your career.
And finally, you will get a Certificate of Completion issued by Udemy, which is a great thing you can add to your Resume.
Bạn sẽ học được gì
Understanding Core Concepts of REST APIs
Understanding REST API Design Best Practices
Design REST APIs with OpenAPI and Swagger
Writing Code to Implement REST APIs with Spring Boot
Handling Error for REST APIs
Validate REST API Requests
Testing REST APIs (Unit Tests, Integration Tests)
Securing REST APIs with Spring Security, JWT and OAuth2
Documenting REST APIs with Spring REST Docs
Deploying REST APIs on Cloud (Heroku, AWS, Google Cloud, Azure)
Caching REST APIs with Redis as Cache Server
API Rate Limiting with Bucket4J
Build Different Kinds of REST Clients (Spring MVC, React, Android and Java Swing)
Yêu cầu
- Basic knowledge in web development with HTML, Javascript and CSS
- Basic knowledge in Java Spring framework and Spring Boot
- Basic knowledge in Java programming (Java core and Java web)
- No prior knowledge in REST API required. I will teach you REST API development from the beginning
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
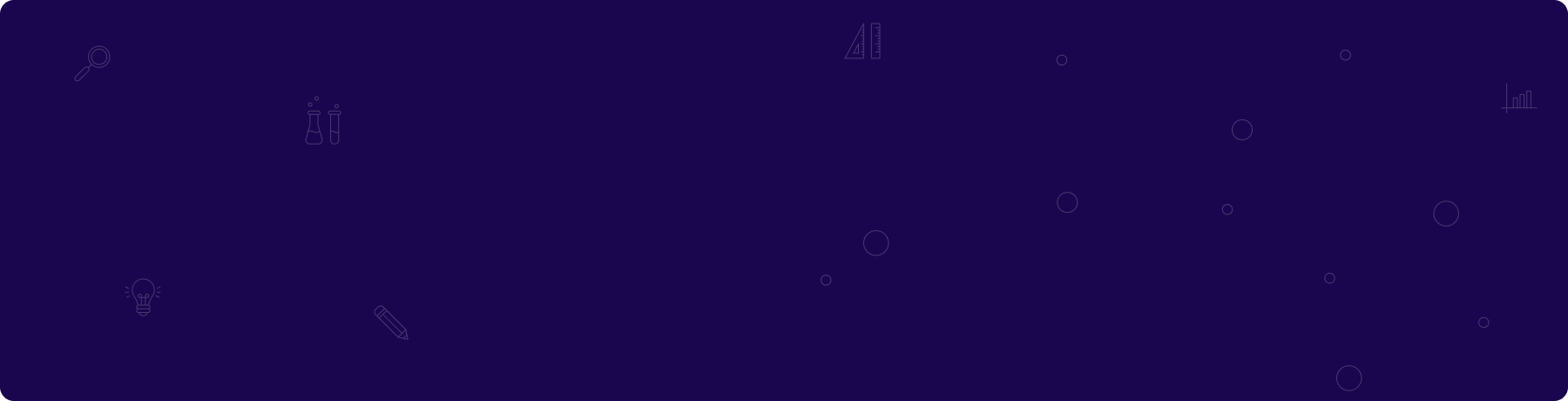
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng