The Complete Data Structures and Algorithms Course in Python
Loại khoá học: Web Development
100+ DSA Interview Questions for Cracking FAANG with Animated Examples for Deeper Understanding and Faster Learning
Mô tả
Welcome to the Complete Data Structures and Algorithms in Python Bootcamp, the most modern, and the most complete Data Structures and Algorithms in Python course on the internet.
At 40+ hours, this is the most comprehensive course online to help you ace your coding interviews and learn about Data Structures and Algorithms in Python. You will see 100+ Interview Questions done at the top technology companies such as Apple,Amazon, Google and Microsoft and how to face Interviews with comprehensive visual explanatory video materials which will bring you closer towards landing the tech job of your dreams!
Learning Python is one of the fastest ways to improve your career prospects as it is one of the most in demand tech skills! This course will help you in better understanding every detail of Data Structures and how algorithms are implemented in high level programming language.
We'll take you step-by-step through engaging video tutorials and teach you everything you need to succeed as a professional programmer.
After finishing this course, you will be able to:
Learn basic algorithmic techniques such as greedy algorithms, binary search, sorting and dynamic programming to solve programming challenges.
Learn the strengths and weaknesses of a variety of data structures, so you can choose the best data structure for your data and applications
Learn many of the algorithms commonly used to sort data, so your applications will perform efficiently when sorting large datasets
Learn how to apply graph and string algorithms to solve real-world challenges: finding shortest paths on huge maps and assembling genomes from millions of pieces.
Why this course is so special and different from any other resource available online?
This course will take you from very beginning to a very complex and advanced topics in understanding Data Structures and Algorithms!
You will get video lectures explaining concepts clearly with comprehensive visual explanations throughout the course.
You will also see Interview Questions done at the top technology companies such as Apple,Amazon, Google and Microsoft.
I cover everything you need to know about technical interview process!
So whether you are interested in learning the top programming language in the world in-depth
And interested in learning the fundamental Algorithms, Data Structures and performance analysis that make up the core foundational skillset of every accomplished programmer/designer or software architect and is excited to ace your next technical interview this is the course for you!
And this is what you get by signing up today:
Lifetime access to 40+ hours of HD quality videos. No monthly subscription. Learn at your own pace, whenever you want
Friendly and fast support in the course Q&A whenever you have questions or get stuck
FULL money back guarantee for 30 days!
Who is this course for?
Self-taught programmers who have a basic knowledge in Python and want to be professional in Data Structures and Algorithms and begin interviewing in tech positions!
As well as students currently studying computer science and want supplementary material on Data Structures and Algorithms and interview preparation for after graduation!
As well as professional programmers who need practice for upcoming coding interviews.
And finally anybody interested in learning more about data structures and algorithms or the technical interview process!
This course is designed to help you to achieve your career goals. Whether you are looking to get more into Data Structures and Algorithms , increase your earning potential or just want a job with more freedom, this is the right course for you!
The topics that are covered in this course.
Section 1 - Introduction
What are Data Structures?
What is an algorithm?
Why are Data Structures and Algorithms important?
Types of Data Structures
Types of Algorithms
Section 2 - Recursion
What is Recursion?
Why do we need recursion?
How Recursion works?
Recursive vs Iterative Solutions
When to use/avoid Recursion?
How to write Recursion in 3 steps?
How to find Fibonacci numbers using Recursion?
Section 3 - Cracking Recursion Interview Questions
Question 1 - Sum of Digits
Question 2 - Power
Question 3 - Greatest Common Divisor
Question 4 - Decimal To Binary
Section 4 - Bonus CHALLENGING Recursion Problems (Exercises)
power
factorial
productofArray
recursiveRange
fib
reverse
isPalindrome
someRecursive
flatten
captalizeFirst
nestedEvenSum
capitalizeWords
stringifyNumbers
collectStrings
Section 5 - Big O Notation
Analogy and Time Complexity
Big O, Big Theta and Big Omega
Time complexity examples
Space Complexity
Drop the Constants and the non dominant terms
Add vs Multiply
How to measure the codes using Big O?
How to find time complexity for Recursive calls?
How to measure Recursive Algorithms that make multiple calls?
Section 6 - Top 10 Big O Interview Questions (Amazon, Facebook, Apple and Microsoft)
Product and Sum
Print Pairs
Print Unordered Pairs
Print Unordered Pairs 2 Arrays
Print Unordered Pairs 2 Arrays 100000 Units
Reverse
O(N) Equivalents
Factorial Complexity
Fibonacci Complexity
Powers of 2
Section 7 - Arrays
What is an Array?
Types of Array
Arrays in Memory
Create an Array
Insertion Operation
Traversal Operation
Accessing an element of Array
Searching for an element in Array
Deleting an element from Array
Time and Space complexity of One Dimensional Array
One Dimensional Array Practice
Create Two Dimensional Array
Insertion - Two Dimensional Array
Accessing an element of Two Dimensional Array
Traversal - Two Dimensional Array
Searching for an element in Two Dimensional Array
Deletion - Two Dimensional Array
Time and Space complexity of Two Dimensional Array
When to use/avoid array
Section 8 - Python Lists
What is a List? How to create it?
Accessing/Traversing a list
Update/Insert a List
Slice/ from a List
Searching for an element in a List
List Operations/Functions
Lists and strings
Common List pitfalls and ways to avoid them
Lists vs Arrays
Time and Space Complexity of List
List Interview Questions
Section 9 - Cracking Array/List Interview Questions (Amazon, Facebook, Apple and Microsoft)
Question 1 - Missing Number
Question 2 - Pairs
Question 3 - Finding a number in an Array
Question 4 - Max product of two int
Question 5 - Is Unique
Question 6 - Permutation
Question 7 - Rotate Matrix
Section 10 - CHALLENGING Array/List Problems (Exercises)
Middle Function
2D Lists
Best Score
Missing Number
Duplicate Number
Pairs
Section 11 - Dictionaries
What is a Dictionary?
Create a Dictionary
Dictionaries in memory
Insert /Update an element in a Dictionary
Traverse through a Dictionary
Search for an element in a Dictionary
Delete / Remove an element from a Dictionary
Dictionary Methods
Dictionary operations/ built in functions
Dictionary vs List
Time and Space Complexity of a Dictionary
Dictionary Interview Questions
Section 12 - Tuples
What is a Tuple? How to create it?
Tuples in Memory / Accessing an element of Tuple
Traversing a Tuple
Search for an element in Tuple
Tuple Operations/Functions
Tuple vs List
Time and Space complexity of Tuples
Tuple Questions
Section 13 - Linked List
What is a Linked List?
Linked List vs Arrays
Types of Linked List
Linked List in the Memory
Creation of Singly Linked List
Insertion in Singly Linked List in Memory
Insertion in Singly Linked List Algorithm
Insertion Method in Singly Linked List
Traversal of Singly Linked List
Search for a value in Single Linked List
Deletion of node from Singly Linked List
Deletion Method in Singly Linked List
Deletion of entire Singly Linked List
Time and Space Complexity of Singly Linked List
Section 14 - Circular Singly Linked List
Creation of Circular Singly Linked List
Insertion in Circular Singly Linked List
Insertion Algorithm in Circular Singly Linked List
Insertion method in Circular Singly Linked List
Traversal of Circular Singly Linked List
Searching a node in Circular Singly Linked List
Deletion of a node from Circular Singly Linked List
Deletion Algorithm in Circular Singly Linked List
Method in Circular Singly Linked List
Deletion of entire Circular Singly Linked List
Time and Space Complexity of Circular Singly Linked List
Section 15 - Doubly Linked List
Creation of Doubly Linked List
Insertion in Doubly Linked List
Insertion Algorithm in Doubly Linked List
Insertion Method in Doubly Linked List
Traversal of Doubly Linked List
Reverse Traversal of Doubly Linked List
Searching for a node in Doubly Linked List
Deletion of a node in Doubly Linked List
Deletion Algorithm in Doubly Linked List
Deletion Method in Doubly Linked List
Deletion of entire Doubly Linked List
Time and Space Complexity of Doubly Linked List
Section 16 - Circular Doubly Linked List
Creation of Circular Doubly Linked List
Insertion in Circular Doubly Linked List
Insertion Algorithm in Circular Doubly Linked List
Insertion Method in Circular Doubly Linked List
Traversal of Circular Doubly Linked List
Reverse Traversal of Circular Doubly Linked List
Search for a node in Circular Doubly Linked List
Delete a node from Circular Doubly Linked List
Deletion Algorithm in Circular Doubly Linked List
Deletion Method in Circular Doubly Linked List
Entire Circular Doubly Linked List
Time and Space Complexity of Circular Doubly Linked List
Time Complexity of Linked List vs Arrays
Section 17 - Cracking Linked List Interview Questions (Amazon, Facebook, Apple and Microsoft)
Linked List Class
Question 1 - Remove Dups
Question 2 - Return Kth to Last
Question 3 - Partition
Question 4 - Sum Linked Lists
Question 5 - Intersection
Section 18 - Stack
What is a Stack?
Stack Operations
Create Stack using List without size limit
Operations on Stack using List (push, pop, peek, isEmpty, )
Create Stack with limit (pop, push, peek, isFull, isEmpty, )
Create Stack using Linked List
Operation on Stack using Linked List (pop, push, peek, isEmpty, )
Time and Space Complexity of Stack using Linked List
When to use/avoid Stack
Stack Quiz
Section 19 - Queue
What is Queue?
Queue using Python List - no size limit
Queue using Python List - no size limit , operations (enqueue, dequeue, peek)
Circular Queue - Python List
Circular Queue - Python List, Operations (enqueue, dequeue, peek, )
Queue - Linked List
Queue - Linked List, Operations (Create, Enqueue)
Queue - Linked List, Operations (Dequeue(), isEmpty, Peek)
Time and Space complexity of Queue using Linked List
List vs Linked List Implementation
Collections Module
Queue Module
Multiprocessing module
Section 20 - Cracking Stack and Queue Interview Questions (Amazon,Facebook, Apple, Microsoft)
Question 1 - Three in One
Question 2 - Stack Minimum
Question 3 - Stack of Plates
Question 4 - Queue via Stacks
Question 5 - Animal Shelter
Section 21 - Tree / Binary Tree
What is a Tree?
Why Tree?
Tree Terminology
How to create a basic tree in Python?
Binary Tree
Types of Binary Tree
Binary Tree Representation
Create Binary Tree (Linked List)
PreOrder Traversal Binary Tree (Linked List)
InOrder Traversal Binary Tree (Linked List)
PostOrder Traversal Binary Tree (Linked List)
LevelOrder Traversal Binary Tree (Linked List)
Searching for a node in Binary Tree (Linked List)
Inserting a node in Binary Tree (Linked List)
Delete a node from Binary Tree (Linked List)
Delete entire Binary Tree (Linked List)
Create Binary Tree (Python List)
Insert a value Binary Tree (Python List)
Search for a node in Binary Tree (Python List)
PreOrder Traversal Binary Tree (Python List)
InOrder Traversal Binary Tree (Python List)
PostOrder Traversal Binary Tree (Python List)
Level Order Traversal Binary Tree (Python List)
Delete a node from Binary Tree (Python List)
Entire Binary Tree (Python List)
Linked List vs Python List Binary Tree
Section 22 - Binary Search Tree
What is a Binary Search Tree? Why do we need it?
Create a Binary Search Tree
Insert a node to BST
Traverse BST
Search in BST
Delete a node from BST
Delete entire BST
Time and Space complexity of BST
Section 23 - AVL Tree
What is an AVL Tree?
Why AVL Tree?
Common Operations on AVL Trees
Insert a node in AVL (Left Left Condition)
Insert a node in AVL (Left Right Condition)
Insert a node in AVL (Right Right Condition)
Insert a node in AVL (Right Left Condition)
Insert a node in AVL (all together)
Insert a node in AVL (method)
Delete a node from AVL (LL, LR, RR, RL)
Delete a node from AVL (all together)
Delete a node from AVL (method)
Delete entire AVL
Time and Space complexity of AVL Tree
Section 24 - Binary Heap
What is Binary Heap? Why do we need it?
Common operations (Creation, Peek, sizeofheap) on Binary Heap
Insert a node in Binary Heap
Extract a node from Binary Heap
Delete entire Binary Heap
Time and space complexity of Binary Heap
Section 25 - Trie
What is a Trie? Why do we need it?
Common Operations on Trie (Creation)
Insert a string in Trie
Search for a string in Trie
Delete a string from Trie
Practical use of Trie
Section 26 - Hashing
What is Hashing? Why do we need it?
Hashing Terminology
Hash Functions
Types of Collision Resolution Techniques
Hash Table is Full
Pros and Cons of Resolution Techniques
Practical Use of Hashing
Hashing vs Other Data structures
Section 27 - Sort Algorithms
What is Sorting?
Types of Sorting
Sorting Terminologies
Bubble Sort
Selection Sort
Insertion Sort
Bucket Sort
Merge Sort
Quick Sort
Heap Sort
Comparison of Sorting Algorithms
Section 28 - Searching Algorithms
Introduction to Searching Algorithms
Linear Search
Linear Search in Python
Binary Search
Binary Search in Python
Time Complexity of Binary Search
Section 29 - Graph Algorithms
What is a Graph? Why Graph?
Graph Terminology
Types of Graph
Graph Representation
Create a graph using Python
Graph traversal - BFS
BFS Traversal in Python
Graph Traversal - DFS
DFS Traversal in Python
BFS Traversal vs DFS Traversal
Topological Sort
Topological Sort Algorithm
Topological Sort in Python
Single Source Shortest Path Problem (SSSPP)
BFS for Single Source Shortest Path Problem (SSSPP)
BFS for Single Source Shortest Path Problem (SSSPP) in Python
Why does BFS not work with weighted Graphs?
Why does DFS not work for SSSP?
Dijkstra's Algorithm for SSSP
Dijkstra's Algorithm in Python
Dijkstra Algorithm with negative cycle
Bellman Ford Algorithm
Bellman Ford Algorithm with negative cycle
Why does Bellman Ford run V-1 times?
Bellman Ford in Python
BFS vs Dijkstra vs Bellman Ford
All pairs shortest path problem
Dry run for All pair shortest path
Floyd Warshall Algorithm
Why Floyd Warshall?
Floyd Warshall with negative cycle,
Floyd Warshall in Python,
BFS vs Dijkstra vs Bellman Ford vs Floyd Warshall,
Minimum Spanning Tree,
Disjoint Set,
Disjoint Set in Python,
Kruskal Algorithm,
Kruskal Algorithm in Python,
Prim's Algorithm,
Prim's Algorithm in Python,
Prim's vs Kruskal
Section 30 - Greedy Algorithms
What is Greedy Algorithm?
Well known Greedy Algorithms
Activity Selection Problem
Activity Selection Problem in Python
Coin Change Problem
Coin Change Problem in Python
Fractional Knapsack Problem
Fractional Knapsack Problem in Python
Section 31 - Divide and Conquer Algorithms
What is a Divide and Conquer Algorithm?
Common Divide and Conquer algorithms
How to solve Fibonacci series using Divide and Conquer approach?
Number Factor
Number Factor in Python
House Robber
House Robber Problem in Python
Convert one string to another
Convert One String to another in Python
Zero One Knapsack problem
Zero One Knapsack problem in Python
Longest Common Sequence Problem
Longest Common Subsequence in Python
Longest Palindromic Subsequence Problem
Longest Palindromic Subsequence in Python
Minimum cost to reach the Last cell problem
Minimum Cost to reach the Last Cell in 2D array using Python
Number of Ways to reach the Last Cell with given Cost
Number of Ways to reach the Last Cell with given Cost in Python
Section 32 - Dynamic Programming
What is Dynamic Programming? (Overlapping property)
Where does the name of DC come from?
Top Down with Memoization
Bottom Up with Tabulation
Top Down vs Bottom Up
Is Merge Sort Dynamic Programming?
Number Factor Problem using Dynamic Programming
Number Factor : Top Down and Bottom Up
House Robber Problem using Dynamic Programming
House Robber : Top Down and Bottom Up
Convert one string to another using Dynamic Programming
Convert String using Bottom Up
Zero One Knapsack using Dynamic Programming
Zero One Knapsack - Top Down
Zero One Knapsack - Bottom Up
Section 33 - CHALLENGING Dynamic Programming Problems
Longest repeated Subsequence Length problem
Longest Common Subsequence Length problem
Longest Common Subsequence problem
Diff Utility
Shortest Common Subsequence problem
Length of Longest Palindromic Subsequence
Subset Sum Problem
Egg Dropping Puzzle
Maximum Length Chain of Pairs
Section 34 - A Recipe for Problem Solving
Introduction
Step 1 - Understand the problem
Step 2 - Examples
Step 3 - Break it Down
Step 4 - Solve or Simplify
Step 5 - Look Back and Refactor
Bạn sẽ học được gì
Learn, implement, and use different Data Structures
Learn, implement and use different Algorithms
Become a better developer by mastering computer science fundamentals
Learn everything you need to ace difficult coding interviews
Cracking the Coding Interview with 100+ questions with explanations
Time and Space Complexity of Data Structures and Algorithms
Recursion
Big O
Yêu cầu
- Basic Python Programming skills
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
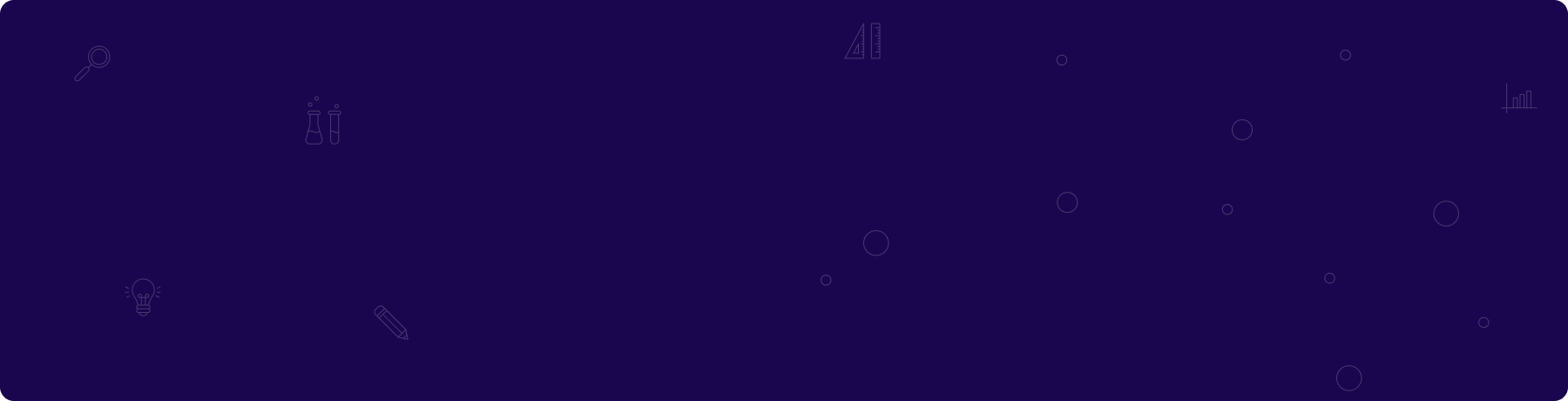
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng