The JavaScript Bible - JavaScript Bootcamp
Loại khoá học: Web Development
Understand and learn JavaScript and ES6 in a one challenge-based JavaScript Bootcamp course!
Mô tả
This course covers everything you need to know about JavaScript and become either Frontend Web developer, or Full-stack Web Developer, or Backend developer.
This course includes more than 70 CHALLENGES and all exercise files are available in Git repositories.
We will start from the very beginning and you will learn fundamentals and basic concepts of JavaScript.
Than you will learn new features included in ES6, ES7 etc.
Also we will dive into the Node.js - environment for JavaScript code execution and you will understand what is the difference between Web Browser and Node.js.
In separate sections we will discuss Babel, NPM, Webpack and MongoDB.
Also you will learn most popular JavaScript framework - React.
JavaScript Bible was designed for developers with different levels of JavaScript knowledge.
If you are BEGINNER in JavaScript - start with very first section called JavaScript Basics.
In case you have SOME experience with JavaScript - jump directly in the sections where I cover ES6 topics such as rest/spread parameters, arrow functions, ES6 Classes etc.
If you are experienced MIDDLE or SENIOR developer with years of JavaScript development background - jump directly into the Challenges and test your knowledge. Each challenge has task and solution in separate Git branches.
All videos have different labels:
LECTURE: in those videos I explain different features and concepts of the language. Main main goal in those videos is to teach you HOW specific feature work under the hood. I don't teach HOW TO USE feature.
Instead I teach you WHY and HOW specific feature works.
PRACTICE: here I will dive into the coding and show you different real-world examples of the usage of specific feature. Usually I will present to you several examples for each specific feature. I strongly recommend you to follow me in those videos and code along with me.
CHALLENGE: each challenge (except simple and short challenges) has START and FINISH branches with task and solution. PLEASE don't skip challenges even if you are already familiar with the topic. Try to solve each challenge yourself.
DEMO: in some videos I will demonstrate you examples where you don't necessarily need to follow me and code along with me
If you want to become an Expert in JavaScript, please join this course now!
See you onboard!
Bạn sẽ học được gì
Become a Senior JavaScript developer by learning and practicing all modern features of the JavaScript. Become able easily apply to Web Developer, Frontend Developer, Backend Developer or Full Stack Web Developer jobs.
Learn JavaScript, ES6, NPM, Webpack, Babel, Node, React by solving tens of CHALLENGES with real-world scenarios. Each CHALLENGE has task and solution. And all of them are available in the GIT repositories that you will download at the beginning of the course.
Learn all FUNDAMENTAL features of the JavaScript starting from basic concepts such as Variables, Objects, Functions, Scopes, Operators and finishing with ADVANCED topics as Closures, Hoisting, IIFEs (Immediately Invoked Function Expressions), Classes and many more.
Learn and understand ES6 features such as Arrow functions, Destructuring, Default function parameters, Template Literals, Array helper methods, Classes
Understand proper methods to work with Arrays - map, forEach, reduce, filter, includes
Deeply understand purpose of the NPM - Node Package Manager
Learn and practice Babel - JavaScript Compiler
Learn basics of the most popular NoSQL database with JavaScript Engine - MongoDB
Yêu cầu
- Just your computer
- Readiness to solve different Challenges yourself
- Passion for coding and learning
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
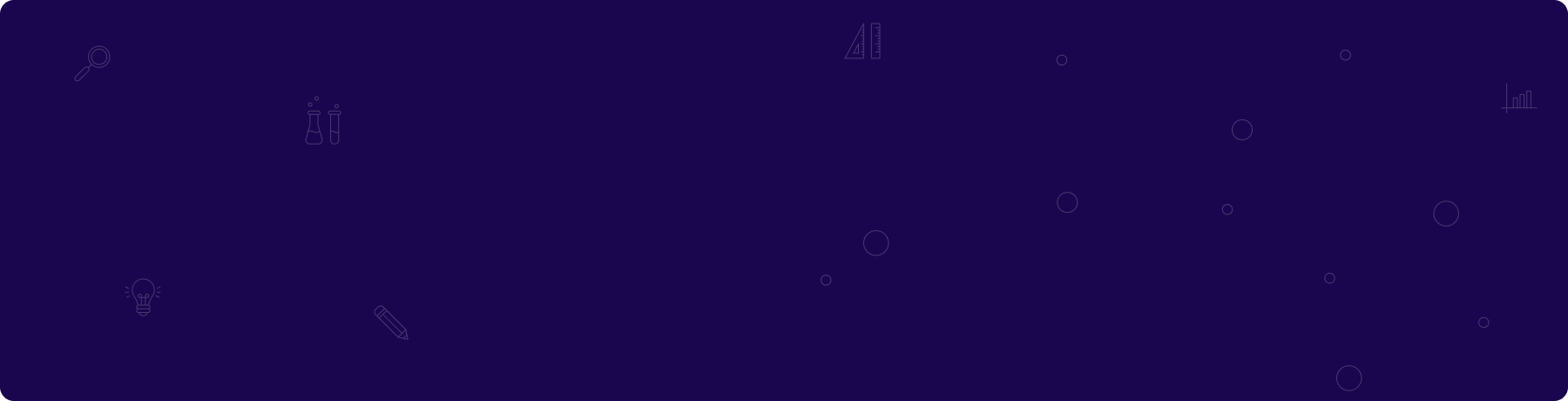
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng