TypeScript 5 for developers
Loại khoá học: Web Development
Use TypeScript like a pro! Quickly learn basic and advanced Typescript and practice it with Node, Express and React!
Mô tả
24.08.2023 - Full course remaster with TypeScript 5
TypeScript is one of the most loved languages of the moment. How can you learn it properly and go beyond basic examples?
Typescript has reinvented the way we code JavaScript, first of all by adding type safety, but also with many other great features like access modifiers, generics, interfaces, classes, decorators and many others.
Typescript combines the flexibility of modern JavaScript with the power of strongly typed languages like Java or C#, making it a great choice for your full stack app.
Welcome to my course, in which you will learn basic and advanced Typescript and NodeJs by practice. We will not waste much time on presentations or reading the documentation, which you can do yourself, no course needed. Instead we will focus on coding a real, full stack application.
Typescript features covered:
Installation and and setup inside a npm project
Basic TypeScript compiler options like sourceDir and Target
Primitive types, build-in types, advanced types, utility types
Any vs unknown vs never
Objects, Interfaces and Classes
Type aliases, type narrowing optional values, type intersection
Enums and exhaustive enums in TypeScript
Abstract classes and inheritance
Generics and decorators - both old and new (version 2 and 3)
Advanced types like conditional types, literal types, mapped types
The Advanced TypeScript environment:
Understand how npm packages work by building and using your own npm package with TypeScript
Run and analyze TypeScript code on both NodeJs and Browser
Deeply understand how module bundlers like WebPack and EsBuild work
Practice sessions with NodeJs, Express and React:
Build from scratch a CRUD application with Node, Express and TypeScript - Rest API
Understand how Express routing works
Understand how Express middleware works
Use ZOD for data validation inside a Node Express app
Deeply understand how module bundlers like WebPack and EsBuild work
Build from scratch a UI app with React and TypeScript
Recap for React state, props and hooks
Understand how React components work and how to pass data between child/parent components
Integrate the Express rest API with React
This course stands out with many advantages and highlights:
Concise and with deep respect for your time: only learn what you need. Most of the instructor typing is cut, focusing on why we write a certain way the code.
Unitary Typescript course theme - the same NodeJs application, so you don't get bored and get a rewarding sense of completion
Experienced and programming active instructor: a great teacher never looses touch with the industry. This is especially true for software development, where the industry is so dynamic. This helps the instructor stay up to date with the best coding guidelines and present you the challenging parts, not the "hello world"
GitHub Code diffs - for each lecture - this way you can keep up and quickly get an overview of the lecture, in case you missed something
Great visuals - dark background, big font, 1080p resolution
Certificate of completion
The best way to learn is by exercise, so I'll see you in class.
There will be code!
Bạn sẽ học được gì
Master the TypeScript type system
Learn how to install, configure and run TypeScript
Use special TypeScript types like unknown, any, never
Learn advanced JavaScript along the way
Deeply understand advanced TypeScript features like Generics, Mapped types, Decorators
Use ES modern syntax async/await, import/export
Learn how to use TypeScript with classes and Object Oriented Programming (OOP)
TypeScript with Express and React
Practice special TypeScript types like Records, mapped types, optional, literal and other utility types
Learn npm, async programming and the ES module system
Learn about module bundlers like Webpack and ESbuild
Build and consume a REST api with Express and React
Yêu cầu
- Some programming experience in any language
- Basic JavaScript
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
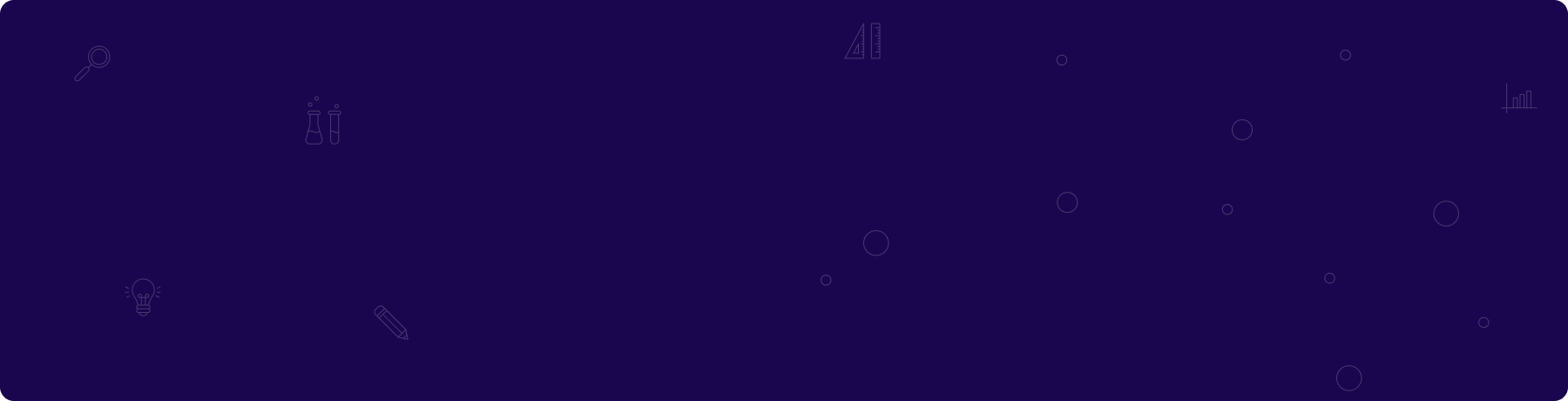
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng