Vue Masterclass (Covers Vue 2 and 3)
Loại khoá học: Web Development
Learn VueJS and build a complete real-world project using Vue, Pinia, TypeScript, TailwindCSS, Vitest, and more.
Mô tả
Welcome to the most comprehensive Vue course on Udemy!
The Vue Masterclass introduces you to the powerful VueJS library for building dynamic, reactive front end interfaces.
VueJS has taken the web development community by storm and is a fantastic technology to learn in 2023:
Vue has been used to built over 1,000,000 websites
Vue has over 200,000 stars on GitHub
Vue downloads on NPM have doubled year-over-year
Vue is used by leading tech companies like Netflix, Apple, GitLab, and Nintendo.
The best way to learn a technology is to create something with it. That's why the Vue Masterclass consists of a complete real-world project that we'll build together from scratch. I'll be coding alongside you from the very first line of code.
I believe this course is the closest I've come to capturing what it feels like to be a Vue developer on the job. We'll introduce and complete user stories, discuss tradeoffs of different technical approaches, summarize what we've learned at the end of each section, and more.
No prior experience with Vue (or any other front end library) is needed. Beginners are welcome!
The course starts with Vue basics and progresses to advanced Vue concepts including:
Creating Vue components
Styling Vue components
Using Vue directives to compose dynamic interfaces
Passing props between components
Emitting events
Routing our user from page to page
Testing Vue components
and more!
Throughout 50+ hours of video content, we'll cover numerous aspects of the Vue ecosystem including:
Vue (including both the Options API from Vue 2 and the new Composition API in Vue 3)
Pinia for global state management
Vue Router for navigating the user across pages in our application
Testing with Vue Testing Library and Vitest
Scaffolding Vue applications with Create Vue
Styling components with Tailwind CSS
Adding type checking with TypeScript
Linting our code with ESLint
Formatting our code with Prettier
and more!
Another aspect that makes the course special is its emphasis on testing. We'll discuss how to unit test our Vue applications using the Vitest and Vue Testing Library packages. We'll also walk through various testing methodologies, including test-driven development (TDD).
Vue Masterclass offers you an incredible, comprehensive introduction to the powerful Vue library. I'm super excited to build this project together with you and I can't wait to see you in the course!
Bạn sẽ học được gì
Build a complete, real-world Vue application using Vue, Pinia, Vue Router, and more
Master modern front end technologies like TailwindCSS, TypeScript, and Prettier
Use Vitest and Vue Testing Libary to write unit tests for all elements of the Vue ecosystem
Learn best practices for Vue component design
Covers both Vue 2's Options API and Vue 3's Composition API
Yêu cầu
- HTML
- CSS
- JavaScript (ES6 features)
- Terminal
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
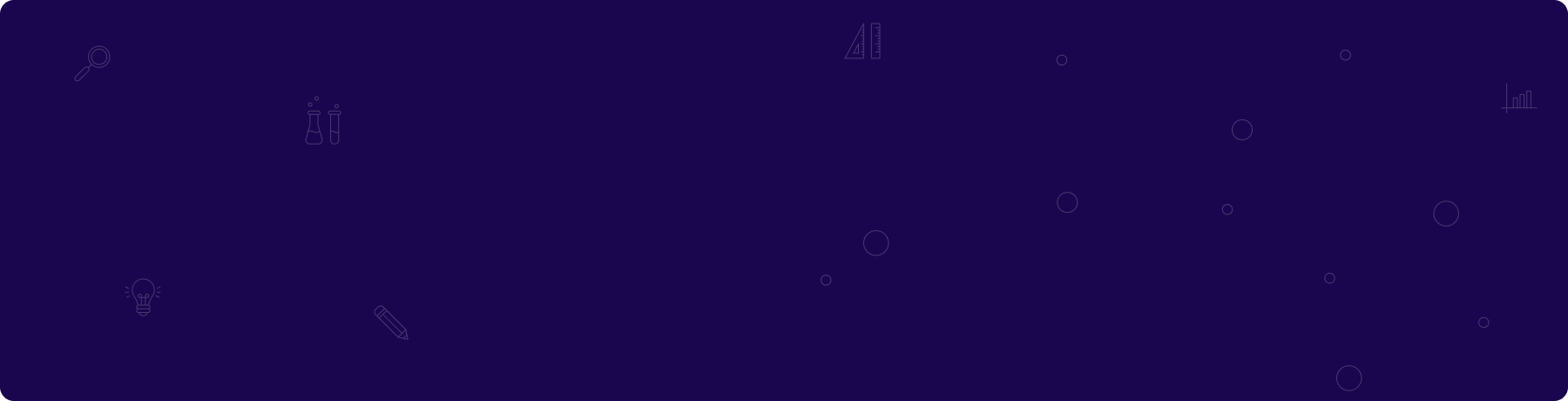
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng