Arduino under the Hood - AVR for Professionals
Loại khoá học: Hardware
From C, Makefiles, and Compiler to all Registers, Periphery, Fuses, Power Reduction Tricks, ISP, DebugWIRE, and LockBits
Mô tả
As one of the most extensive courses available: we will look at everything and more under the hood of Arduino. At its core, the ATmega328P MCU integrates many features that are not exposed through the Arduino library. While learning how the periphery works, the student writes code, exhibiting high performance at low power consumption. Embedded systems deployed in the field and powered by small batteries can run and collect data for years.
The enormous advantage of the AVR platform is that many concepts introduced in this course also apply to other MCUs. Therefore, an entire portfolio of MCUs, ranging from the small ATtiny to the larger ATmega series, becomes accessible. The ATmega328P used in this course is just an example and the gained knowledge is applicable to many embedded systems not limited to the Arduino platform. This is motivated by practical sessions and exercises in which real-world problems are to be solved. In case questions during implementation arise, the student can peek at hints and tips and if nothing works, also at the solutions with plenty of comments in the source code.
This course provides handmade captions in English covering the following topics, which focus on the practical aspects and what a concept can be used for. In addition, the course does not follow a particular outline and the topics can be taken in any order based on the interest and needs. The covered topics include:
GNU Compiler Collection and what happens in every Step and why
A brief introduction to C and Pointers
Moving from the Arduino library to AVR: Less Luxury, faster Execution, fewer Resources
How to compile with Makefiles? Targets, Rules, and Shortcuts
Easy Mistakes in C and how to avoid them
Attack Vectors
Peripheries:
Timers, Counters, and Pulse Width Modulation
Interrupts
Communication Protocols
UART/USART
I2C/TWI
SPI
DHT and 1-Wire
USI
How to mimic other, formerly incompatible, and unsupported Protocols?
Analog-Digital Converter
How does it work?
Measuring the Temperature without external Components
Measuring Battery Level with the ADC
Analog Comparator
Non-volatile Memories
Flash
How to use the Flash (Program Memory) for Constants?
How to use the Flash while executing a Program?
EEPROM
Fuses
Clock Sources
Lock Bits
Debugging
Running the AVR on a Breadboard
Saving power
Sleeping
Dynamic Clock Frequency
Turning off unnecessary Components
Wake-up Sources
Debugging (with practical examples)
Simulators
JTAG
debugWIRE
Bonus Chapter: Parallel Task Execution
After completing this course, the successful student will have the experience not only to evaluate existing software but also create implementations that are highly optimized to be deployed on small microcontrollers to squeeze out every quantum of performance and battery life.
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
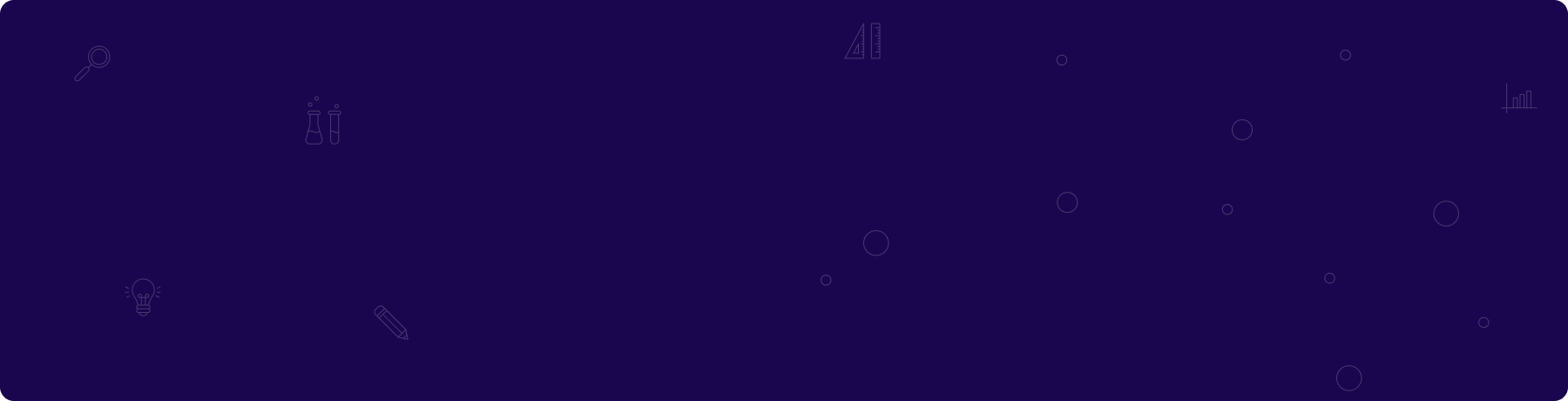
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng