Automation Framework Development with Playwright in C# .NET
Loại khoá học: Software Testing
An End-to-End Playwright automation framework development course
Mô tả
Automation framework development with Playwright in C#.NET is an advanced course designed to address End-to-End test framework development with clean coding and SOLID patterns in place.
The curriculum of this course is designed not just by randomly choosing some of the topics that I know, the course has evolved over a period (15+ years) by giving training to more than 270,000+ professionals (both online and corporate training) and working in automation testing field 15+ years now
Hence the course is much-refined version, meaning the course won't deviate from the topics it is meant to be.
The course includes all the source codes which we will discuss in the course, hence one must have all the source codes required at the end of every section.
Finally, the course is for those, who are serious about automation testing framework development from the complete ground up and understand all the nuts and bots of building them for extensible usage at work
In this course, you will learn to automate ASP NET Web API and WebUI projects using tools such as
Playwright (latest version)
.NET 7
C# 11
XUnit
AutoFixture
Fluent Assertion
Specflow
We will also be using the following patterns in this course
Dependency Injection
Factory Pattern
Page Object Model Pattern
Lazy Initialization
At the end of this course, you will have
Complete understanding of C# with .NET 7
Complete understanding of Playwright with latest and greatest changes
Designing a complete framework from the ground up
All the idea to design a more extendable framework
Bạn sẽ học được gì
Basics of Playwright to more advanced way to write custom frameworks in Playwright
Modern testing techniques and coding standards in .NET
Efficient DI based framework creation
Working with XUnit, AutoFixture, DI and Specflow will make testing with Playwright more awesome
Yêu cầu
- Basics of .NET
- Basics of Selenium or any related tool is a plus
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
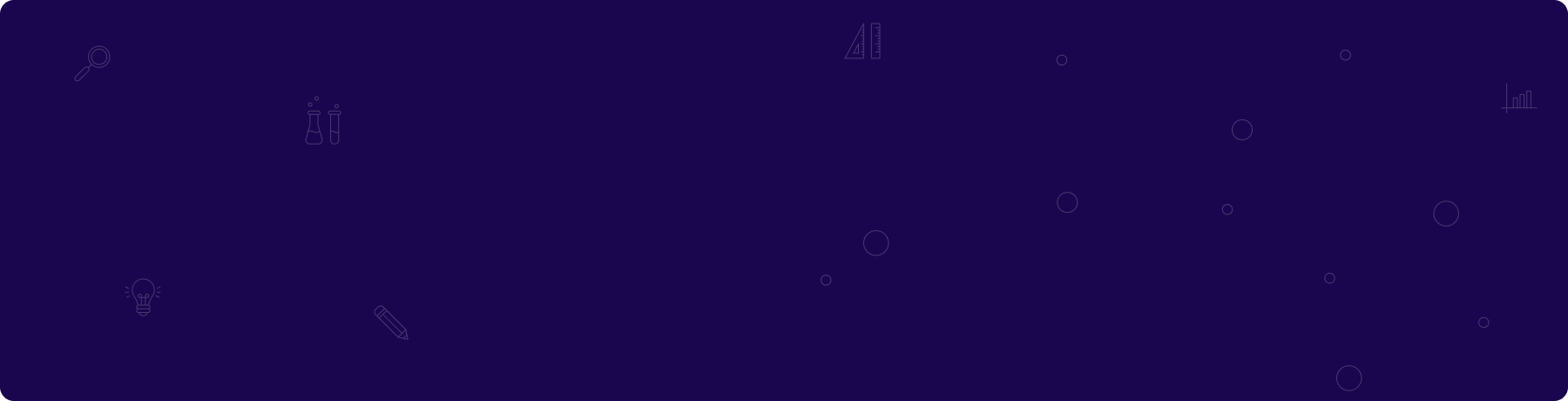
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng