Complete Modern C++ (C++11/14/17)
Loại khoá học: Other IT & Software
Learn about move semantics, lambda expressions, smart pointers, concurrency, template, STL & more
Mô tả
Learn C++ in depth with modern features introduced with C++11/14/17
Updated with C++17 features!
C++ is a general purpose programming language invented by Bjarne Stroustrup. It is still one of the more popular programming languages, used for creating high performance applications across a wide variety of domains & platforms.
In 2011, C++11 was born. This revision added lot of new features to the language and it got a new name, Modern C++. This emphasizes writing C++ code using modern features of the language such as move semantics, automatic type inference, threading, lambda expressions, smart pointers and a lot more. C++11 was followed by C++14, that added even more features and enhanced existing ones. C++17 released in 2017 added a filesystem library (covered in the course), parallel versions of STL algorithms, new library types such as std::optional, std::any and more.
This course teaches C++ as an object oriented language with modern features. It focuses on teaching C++ concepts, both old and new, with clear examples. It builds upon the basic language facilities that are then used to build more complex programs with classes, operator overloading, composition, inheritance, polymorphism, templates, concurrency, etc. It even digs deep into assembly to understand few concepts better. After every few topics, a quiz is presented that tests your understanding of the previous topics. Have fun learning Modern C++.
Note that this course is not for you if
You have never programmed before
You don't know ANY programming language
You want to learn basics of programming
Update [April 19, 2020] : Biggest update so far. Added C++17 core language changes, template enhancement and new library types.
Update [April 11, 2020] : Added content on copy elision, type traits, static_assert & generalized lambda capture, C++11 unions
Update [Mar 25, 2020]: XCode installation & high-level concurrency (std::async)
Update [Mar 1, 2019] : More videos added for memory management with smart pointers (shared_ptr, unique_ptr, weak_ptr_)
Update [Oct 19, 2017] :Instructions added for installing Visual Studio Community 2017, Cevelop (Eclipse) & Code::Blocks
Update [Sep 29, 2107] : Added more content on dynamic memory allocation (malloc, new[], 2D arrays, strings)
Update [Sep 16, 2017] : C++ concurrency (std::thread, std::mutex, etc)
Update [April 27, 2017] : Templates, Function Object, Lambda Expressions, Standard Template Library
Update [Mar 23, 2017] : Virtual Inheritance, Exception handling, File I/O, std::filesystem (C++17)
Update [Mar 4, 2017] : Strings, stringstreams, enums, inheritance, polymorphism
Update [Feb 25, 2017] : Source Code of existing topics added
Bạn sẽ học được gì
Use C++ as an object-oriented language
Demystify function & class templates
Use STL components in your applications
Write real-world applications in C++
Apply Modern C++ (C++11/14/17) in programs
Yêu cầu
- Basic programming knowledge in any computer language
- Some experience of writing programs in any language
- Modern C++ compiler, preferably Visual Studio 2015/17/19 Community (or XCode, Clang, g++, Cevelop, Eclipse CDT, Code::Blocks)
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
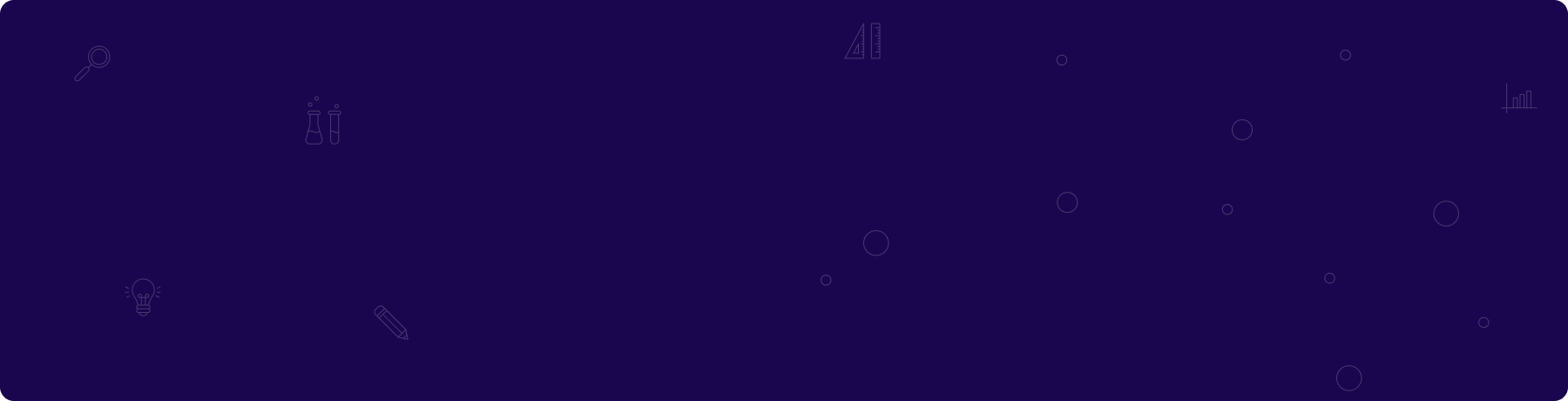
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng