Complete React and Redux Bootcamp Build 10 Hands-On-Projects
Loại khoá học: Web Development
Learn the Major Moncepts React JS like States , Hooks , Context API , REDUX by doing 10 Real-Time Hands-On Projects
Mô tả
React is a JavaScript library for building user interfaces.
Declarative: React makes it painless to create interactive UIs. Design simple views for each state in your application, and React will efficiently update and render just the right components when your data changes. Declarative views make your code more predictable, simpler to understand, and easier to debug.
Component-Based: Build encapsulated components that manage their own state, then compose them to make complex UIs. Since component logic is written in JavaScript instead of templates, you can easily pass rich data through your app and keep the state out of the DOM.
Learn Once, Write Anywhere: We don't make assumptions about the rest of your technology stack, so you can develop new features in React without rewriting existing code. React can also render on the server using Node and power mobile apps using React Native.
Redux is an open-source JavaScript library for managing application state. It works best in extensive, sprawling applications. However, to utilize it properly, first you need to prepare. Everything should go smoothly as long as your middleware is all done and you have full control over fetches, states, etc. In Redux, you don’t have to fetch everything all the time. This is the reason why Redux remains the most popular flux-based tool for state management.
From the architecture point of view, Redux helps maintain order in the folders and files of the project. It helps programmers understand the application’s structure and introduce new people to the project (providing that they have previous knowledge of Redux). Its components are: global JS object, reduction functions, actions and subscriptions.
The widespread use of Redux gets even funnier when you consider the fact that the creators themselves (Dan Abramov and Andrew Clark) used to say that you might not actually need Redux. It’s important to understand what you can do with it before you stick it in your programming tool belt. Especially since there’s another solution that works great not as a competition, but rather as a supplement: React’s API interface!
The origins of SPA, a single-page application, go back to 2013 and the creation of the React library, used by Facebook. One of its biggest advantages was performance, achieved with VDOM.
State management is the repository for the current state of the app and its data. It’s a common part of all the views. For example, in the case of user data; the avatar, the full name, etc. are stored in Redux.
Front-end state management is a kind of logic that stores and refreshes current data, such as the information about the options button being highlighted, about the authorization of the user, etc. Or, if we were to put it in a more abstract way, it makes sure that business transactions are complete – by storing input data of the user interface and synchronizing it across the pages, back-end, and front-end parts.
It’s also helpful in drawing a line between the business and view layers. This makes the app run faster without having to load the same elements all over again – they’re simply stored in Redux.
Bạn sẽ học được gì
React JS
Redux
React Hooks
HTTP Methods
Yêu cầu
- Javascript Basics
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
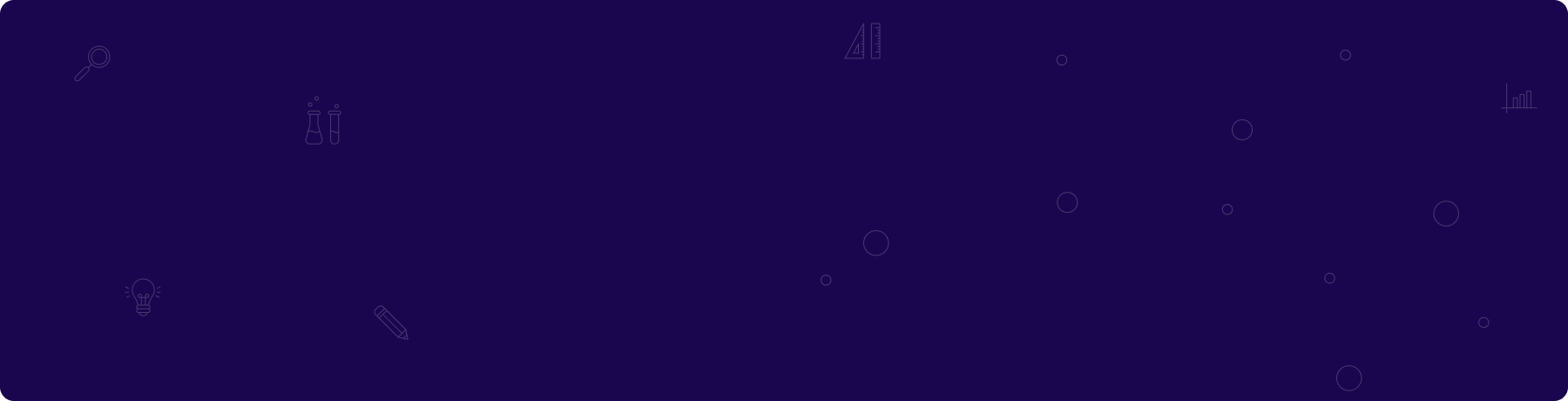
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng