Full Stack Project: Spring Boot, React, Redux
Loại khoá học: Other IT & Software
Build a Personal Project Management Tool from scratch
Mô tả
If you know the basics of java and the spring framework, the next thing to do is to keep on practicing! Building apps with real life features is a great next step in your journey to becoming a developer. The main benefits of this is that it sharpens your skills and helps you build your portfolio for prospective employers. In this course, we will build a prototype of a personal project management tool using Spring boot 2.0 in the backend, ReactJS and Redux on the front end.
These are some of the cool things we will work on:
We will build our REST APIs with Spring boot for CRUD operations
We will create our front end using ReactJS and Boostrap
And will use Redux and Thunk to manage the state of our application in the front-end
We will secure our application using JWT tokens
Last but not least we will deploy our application to Heroku’s free tier. This is a great opportunity to get practical experience with two amazing technologies that are highly desirable by prospective employers.
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
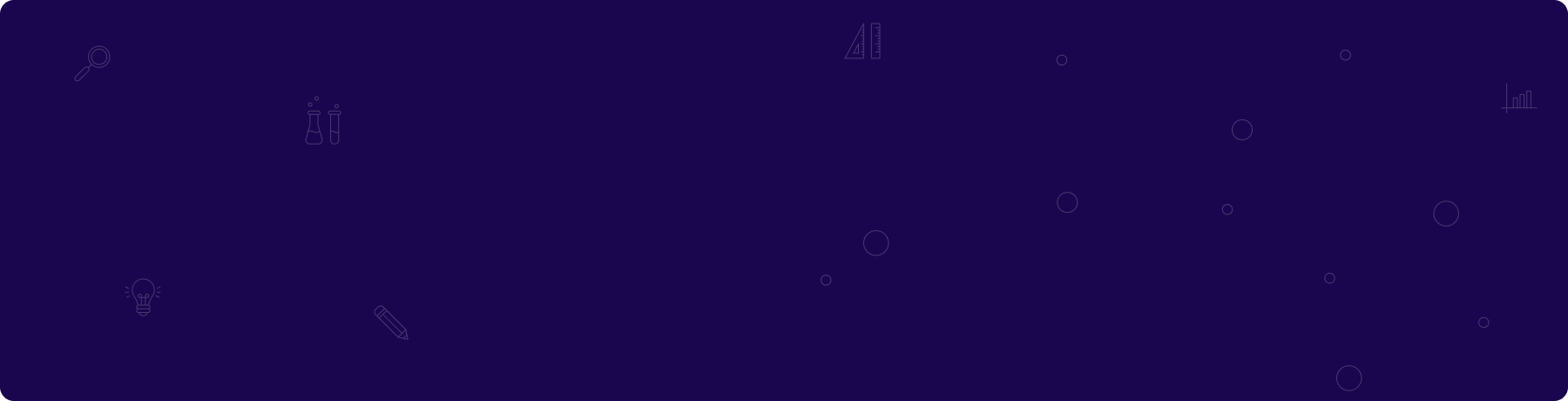
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng